Display project information, filter function and like function
Enterprise Project Management: for a wide range of projects within an enterprise, a Kanban board that contains all projects and supports classification by project type and quick search for projects is very useful. Employees can express their recognition of a project through one-click likes; One-click sharing facilitates the rapid dissemination of information between different levels.
Communication platform of School scientific research groups: research teams can use this kind of project display to track the research parts they are responsible for, and encourage positive interaction among members through praise mechanism. At the same time, the research results can be easily shared with other interested scholars or submitted directly to the tutor for review.
Prerequisites
This tutorial uses some basic functions of YIDA. You can first learn about the following functions.
- Custom page settings
- YIDA platform interface (page data source can be called directly)
- Add JSAPI
- EXIST form formula
Effect
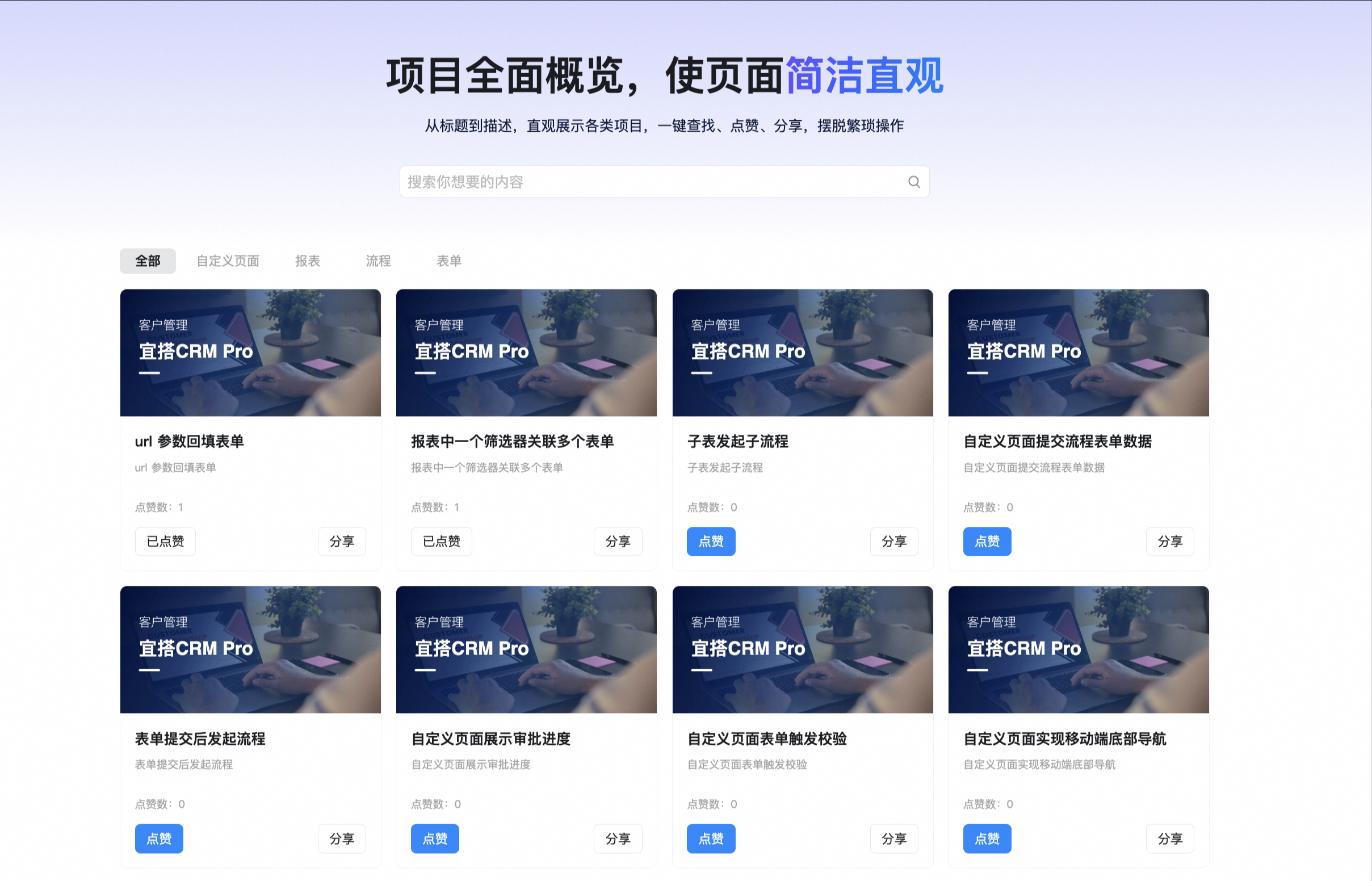
Create a normal form
Create a common form page. For more information, seeCommon form.
Project category
It is used to enter basic data of project categories, including category number, project category, and sorting weight. It can be associated with the [project entry] and [praise record] pages.
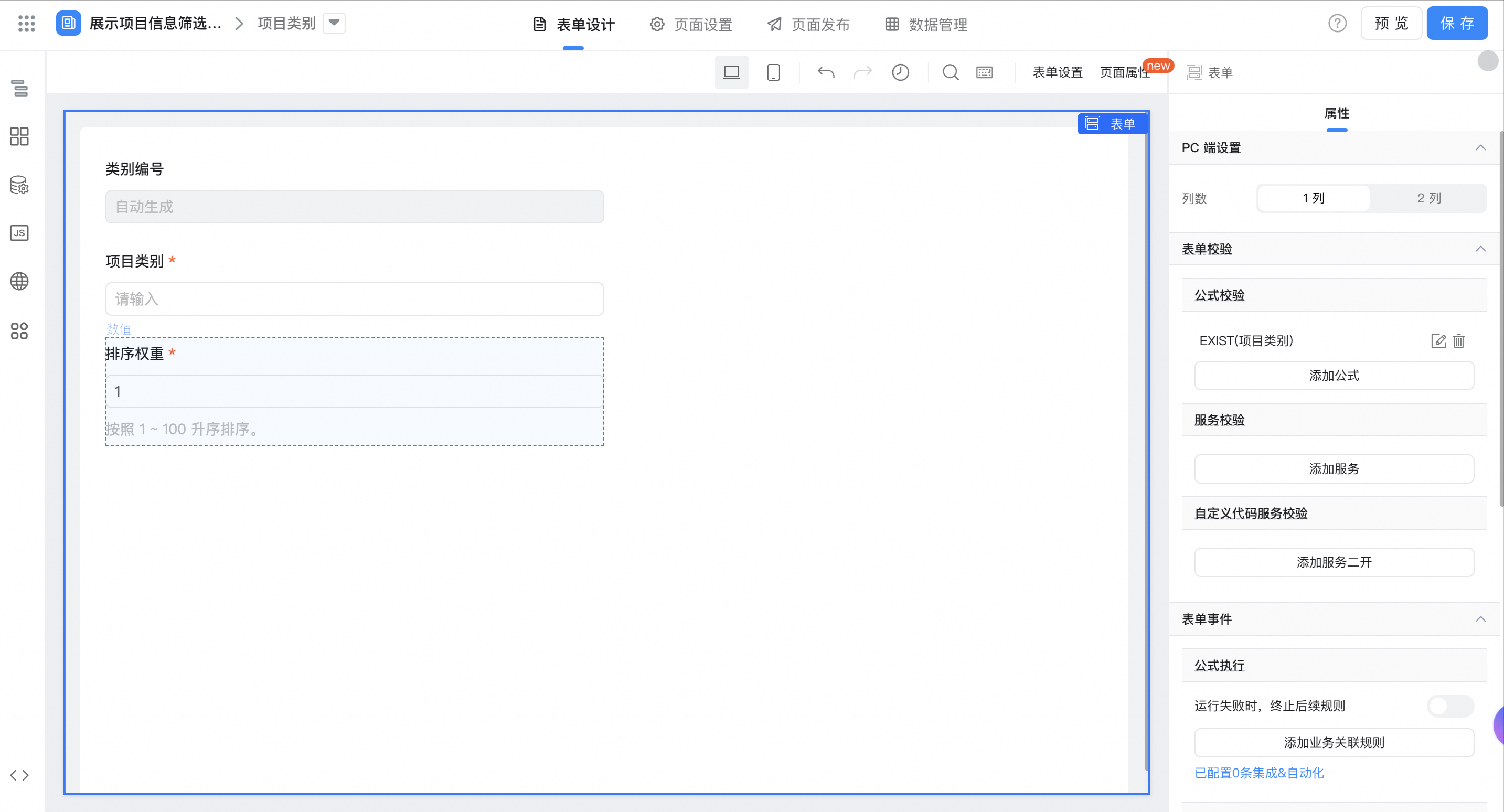
Set the form verification function "EXIST" to control the uniqueness of input item categories.
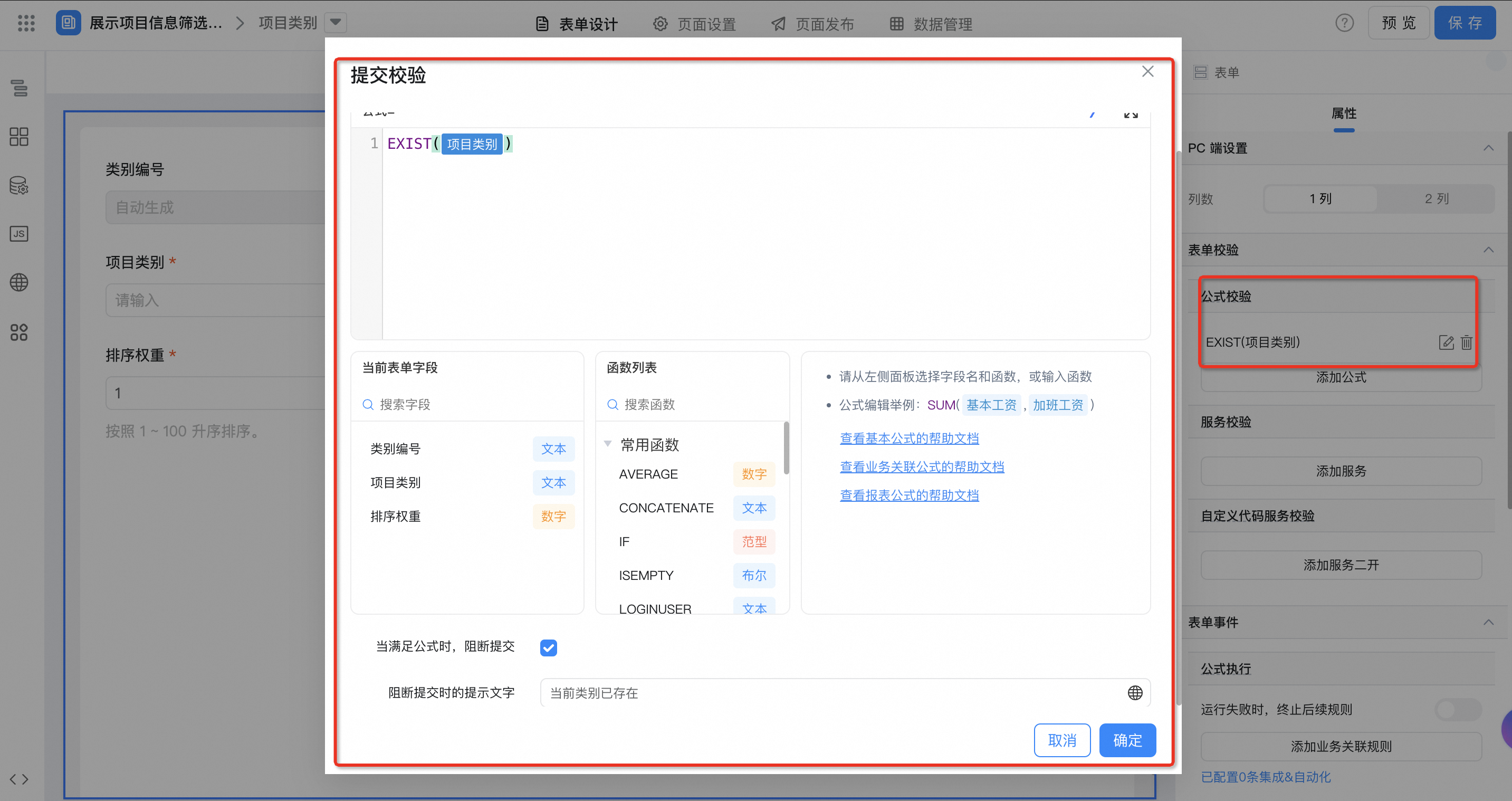
Project entry
On the project data entry page, the basic display component includes the project number, project name, project description, project description, and system field: the number of project likes is used to record the total number of current project likes.
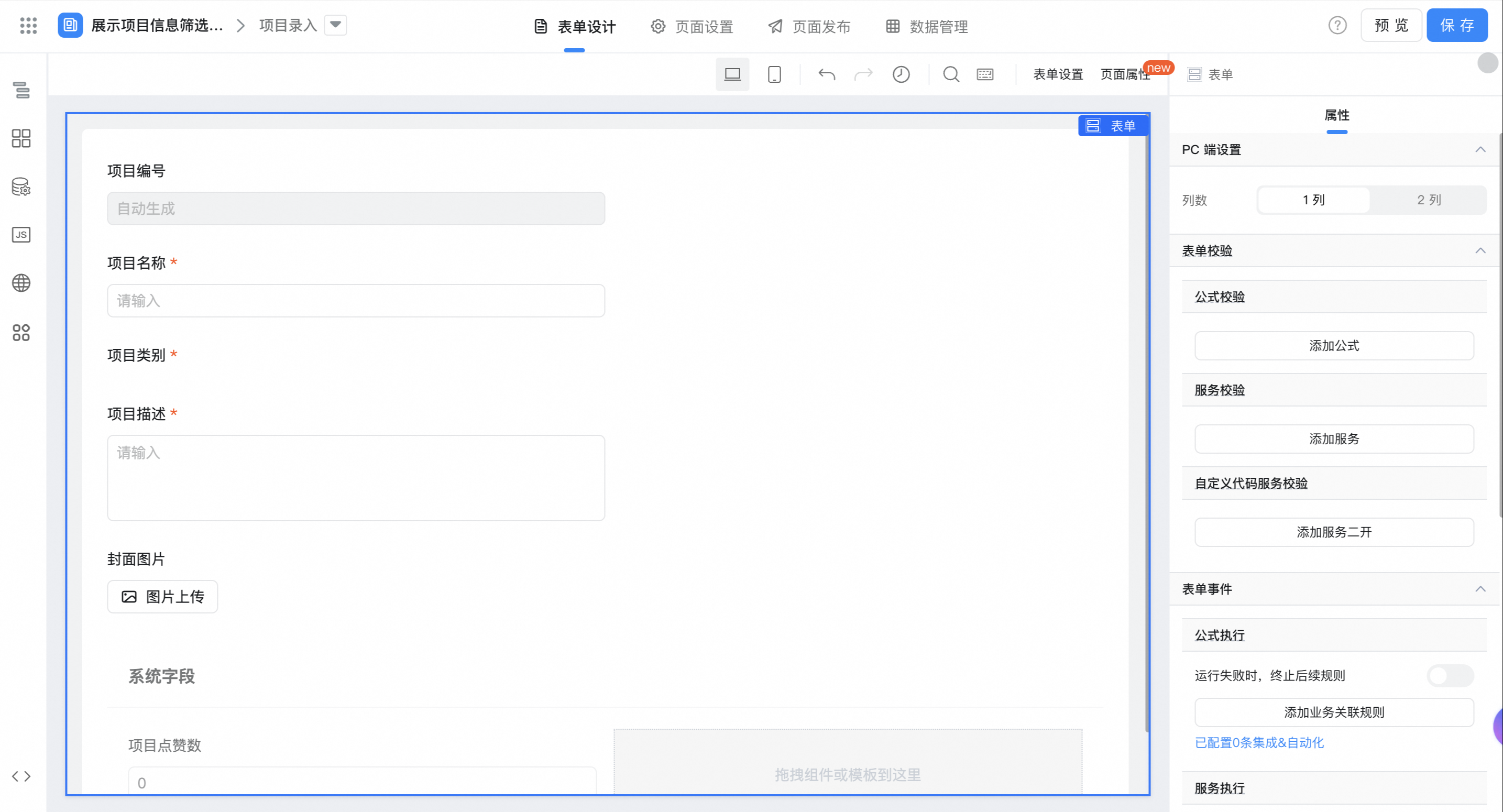
Project category sets the type of options associated with other form data.
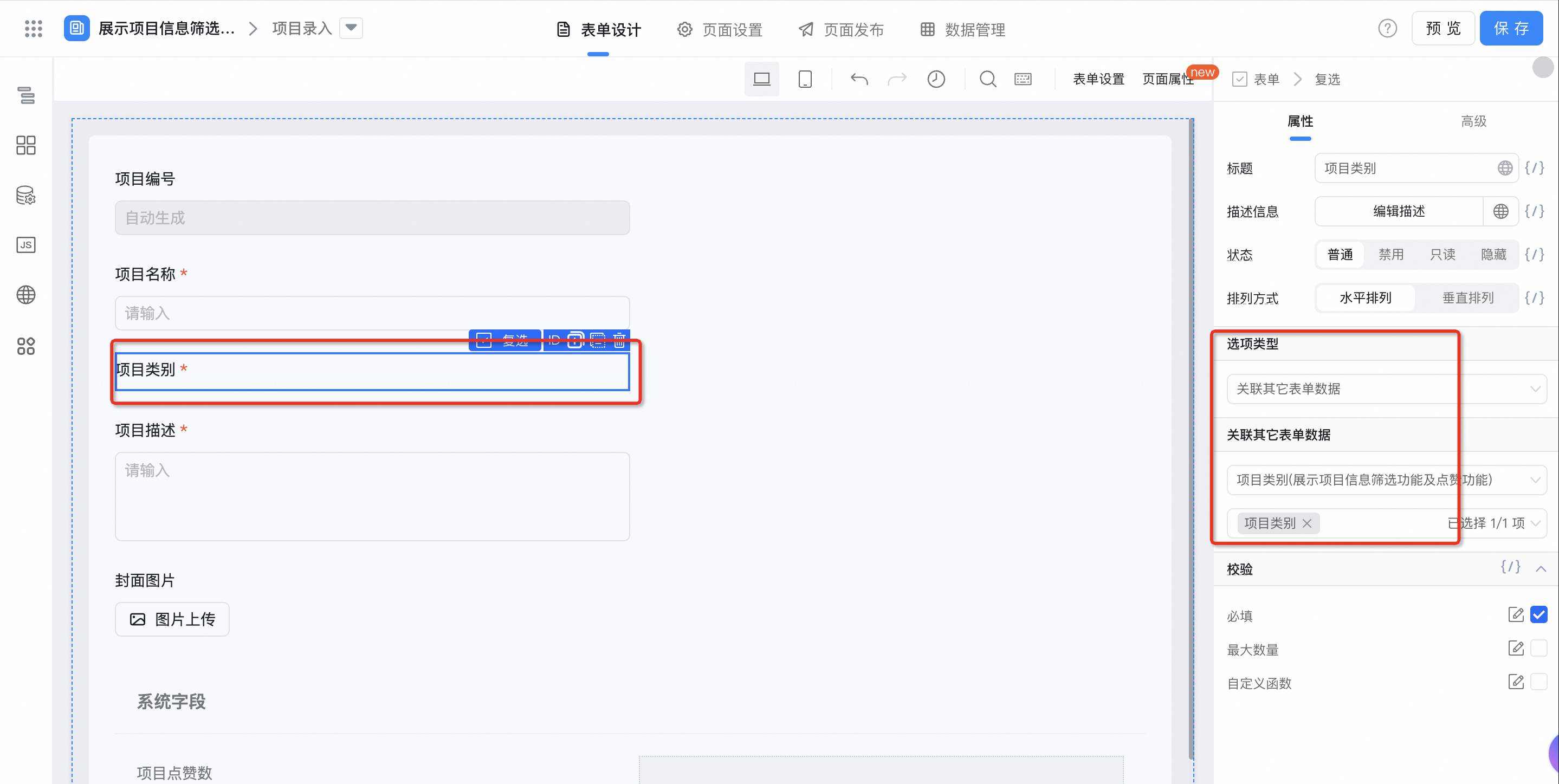
Praise record
It is used to record the basic information of the like person and the like project, including the like person, project number, project category, and the ID of the project instance to be praised.

Project category sets the type of options associated with other form data.
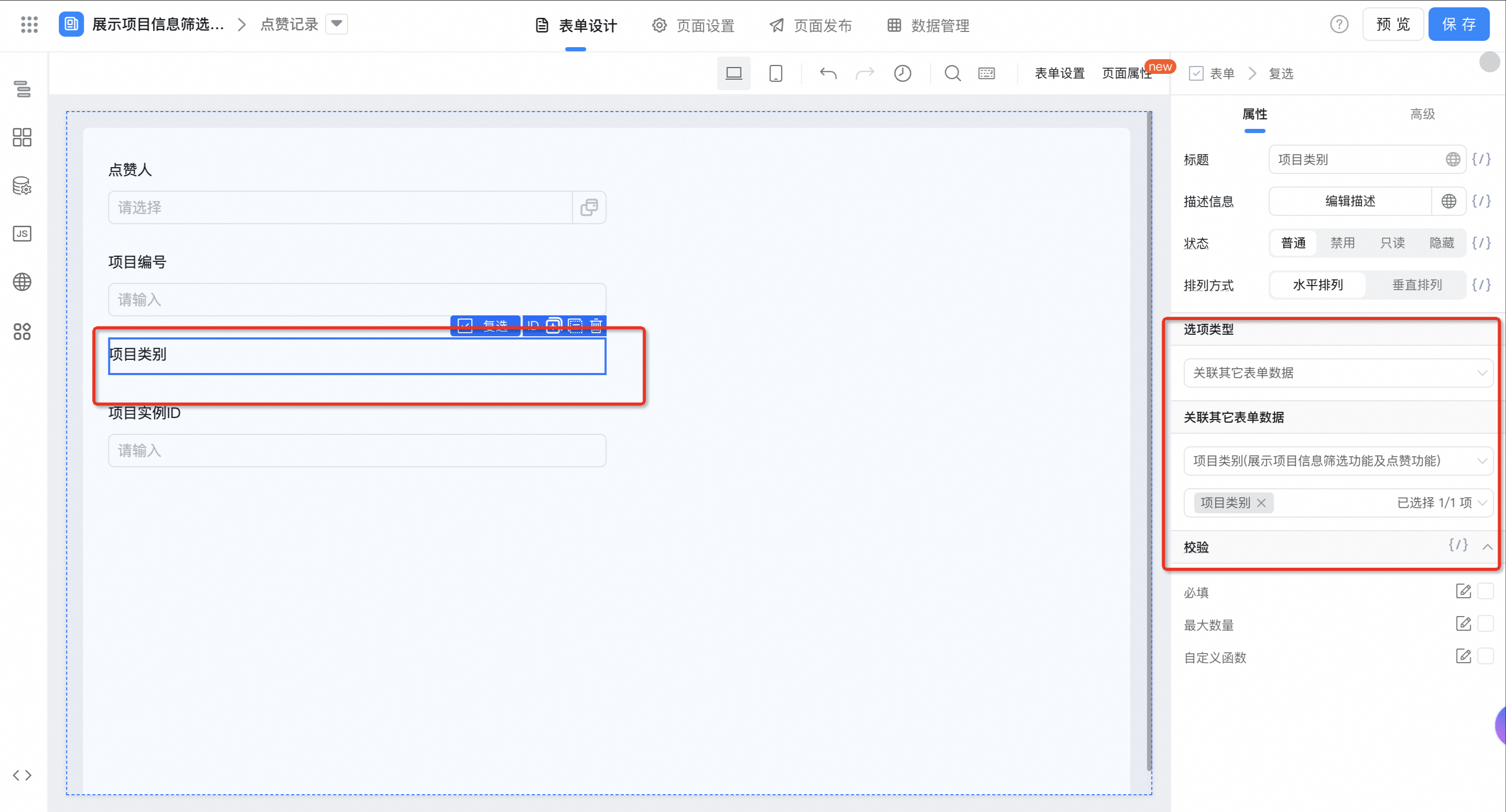
Create a custom page
Create a custom page. For more information, seeCreate a custom page.
Configure remote data sources and variables
Configure remote data sources
Reference documents:Cross-application data source API
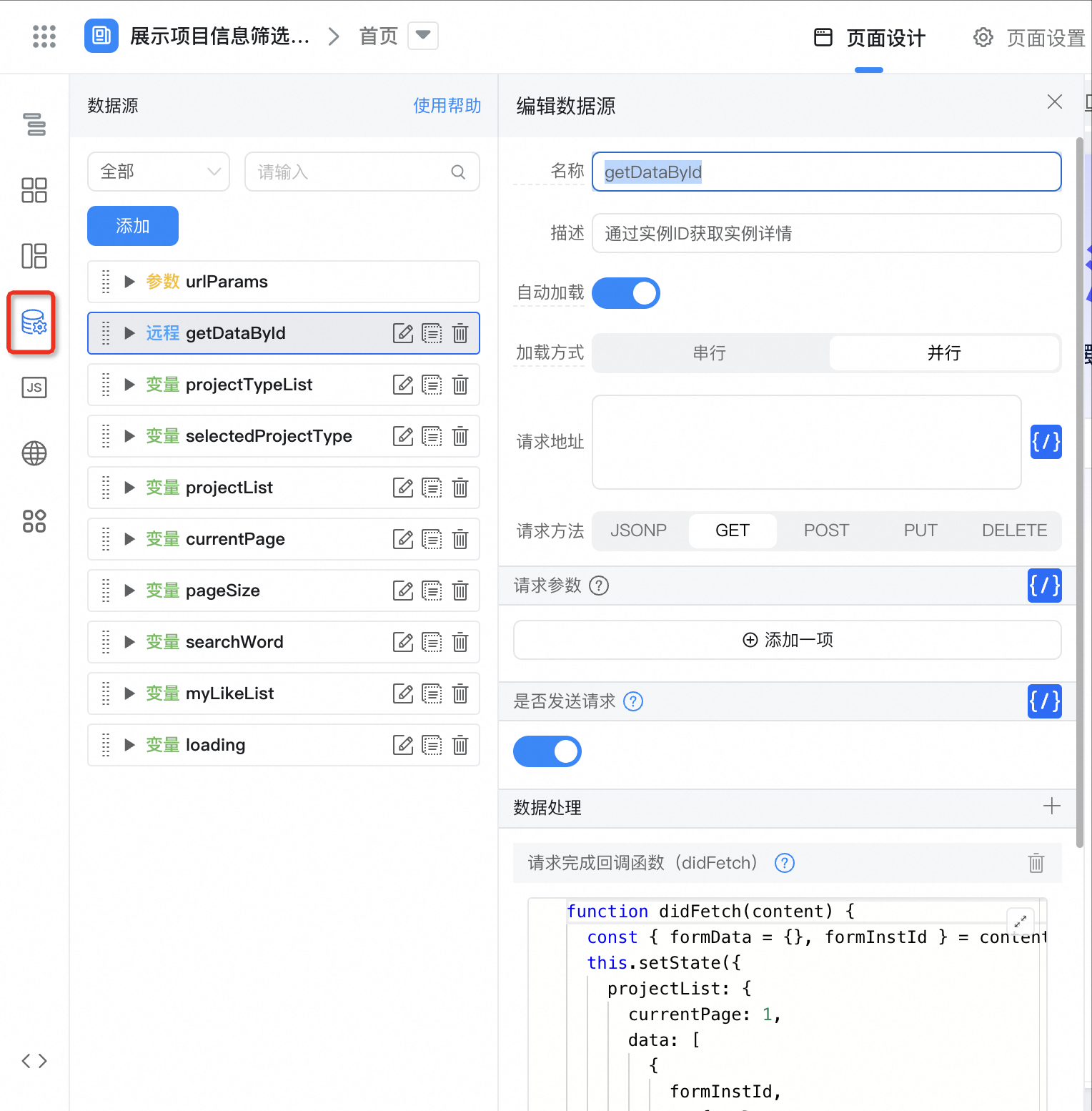
Configure the request address:
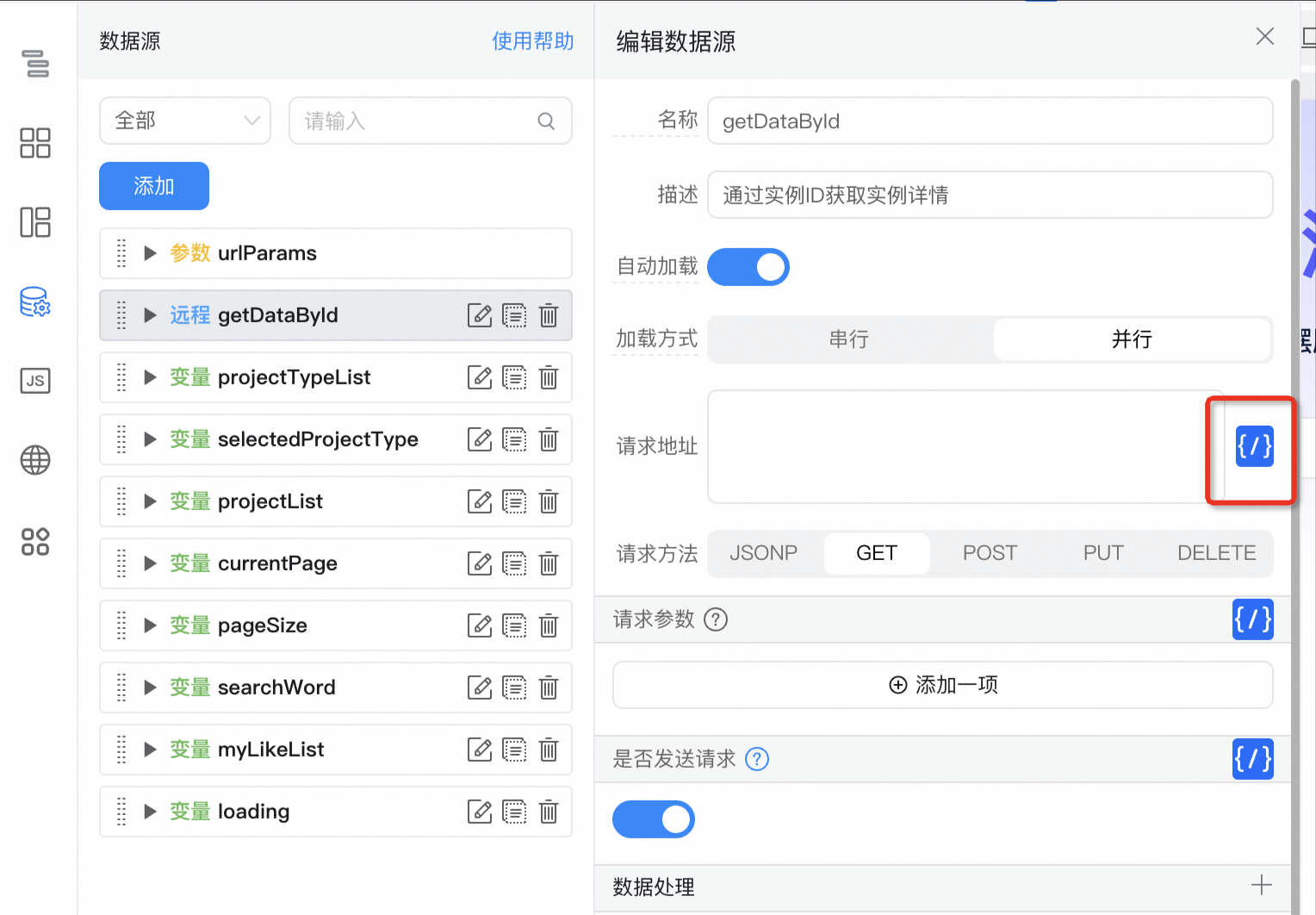
`/${window.pageConfig.appType || window.g_config.appKey}/v1/form/getFormDataById.json`
Configure request parameters:
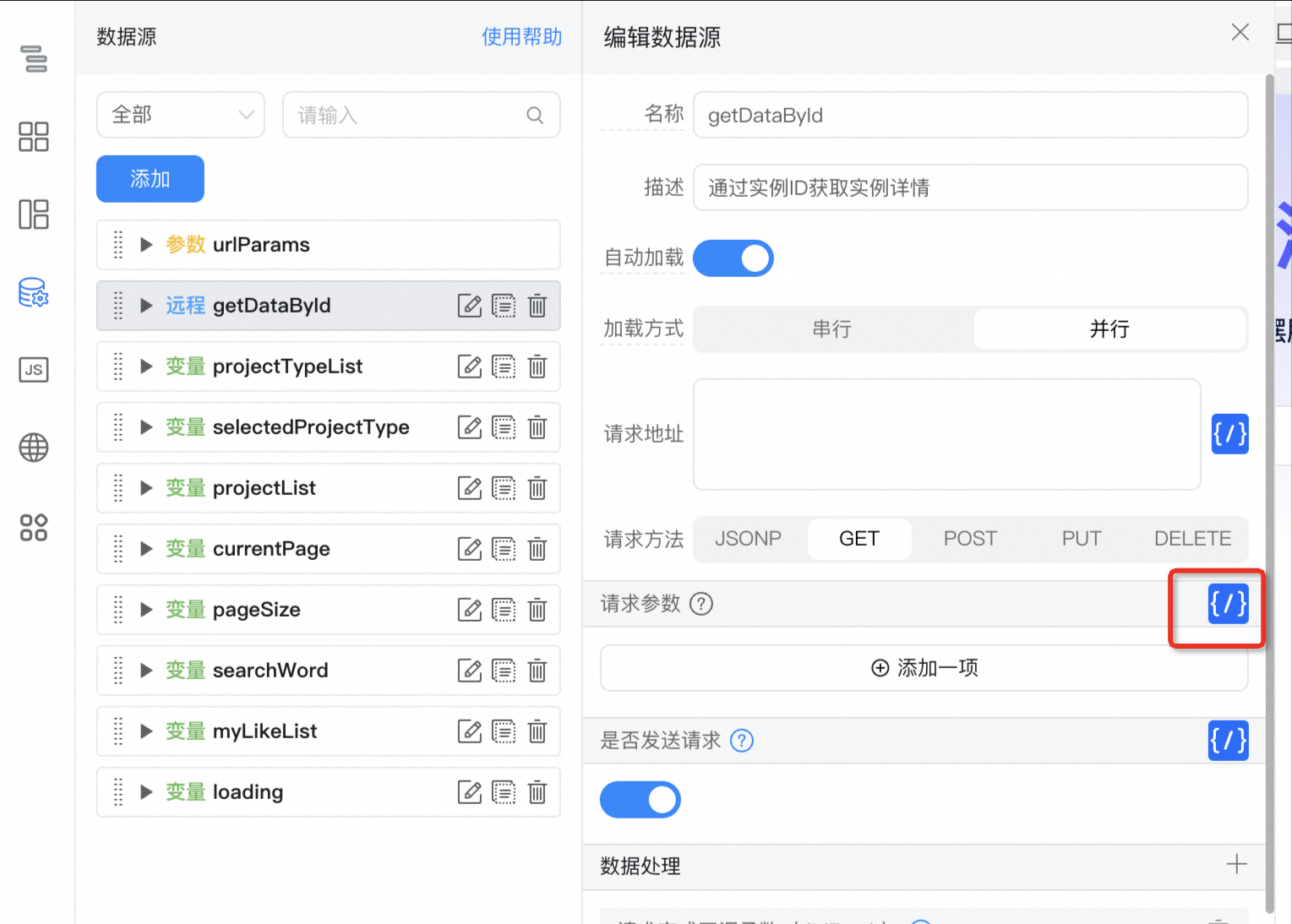
{
formInstId: state.urlParams.projectId,
}
Configure whether to send a request:
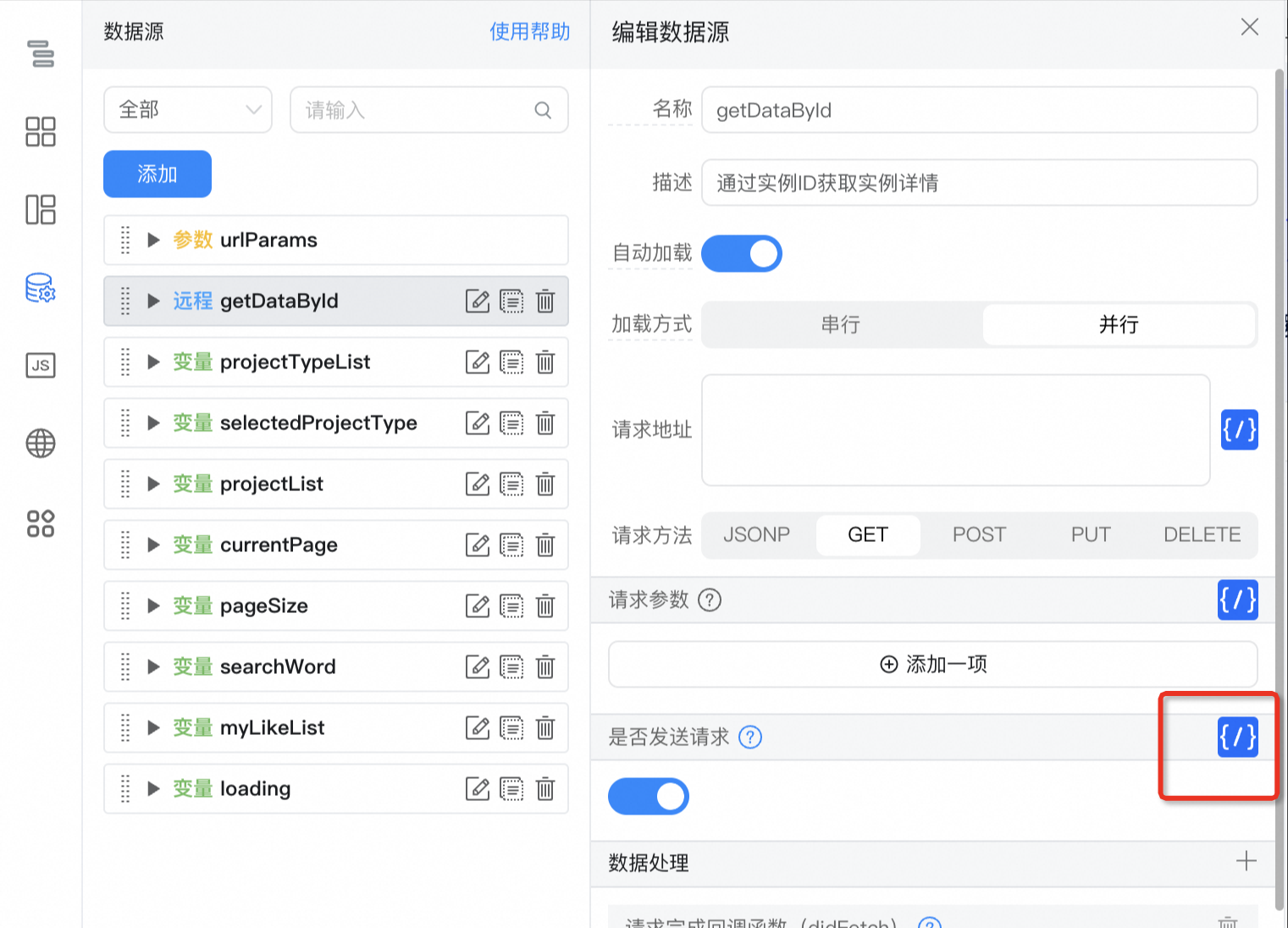
!!state.urlParams.projectId
Add request callback parameter didFetch
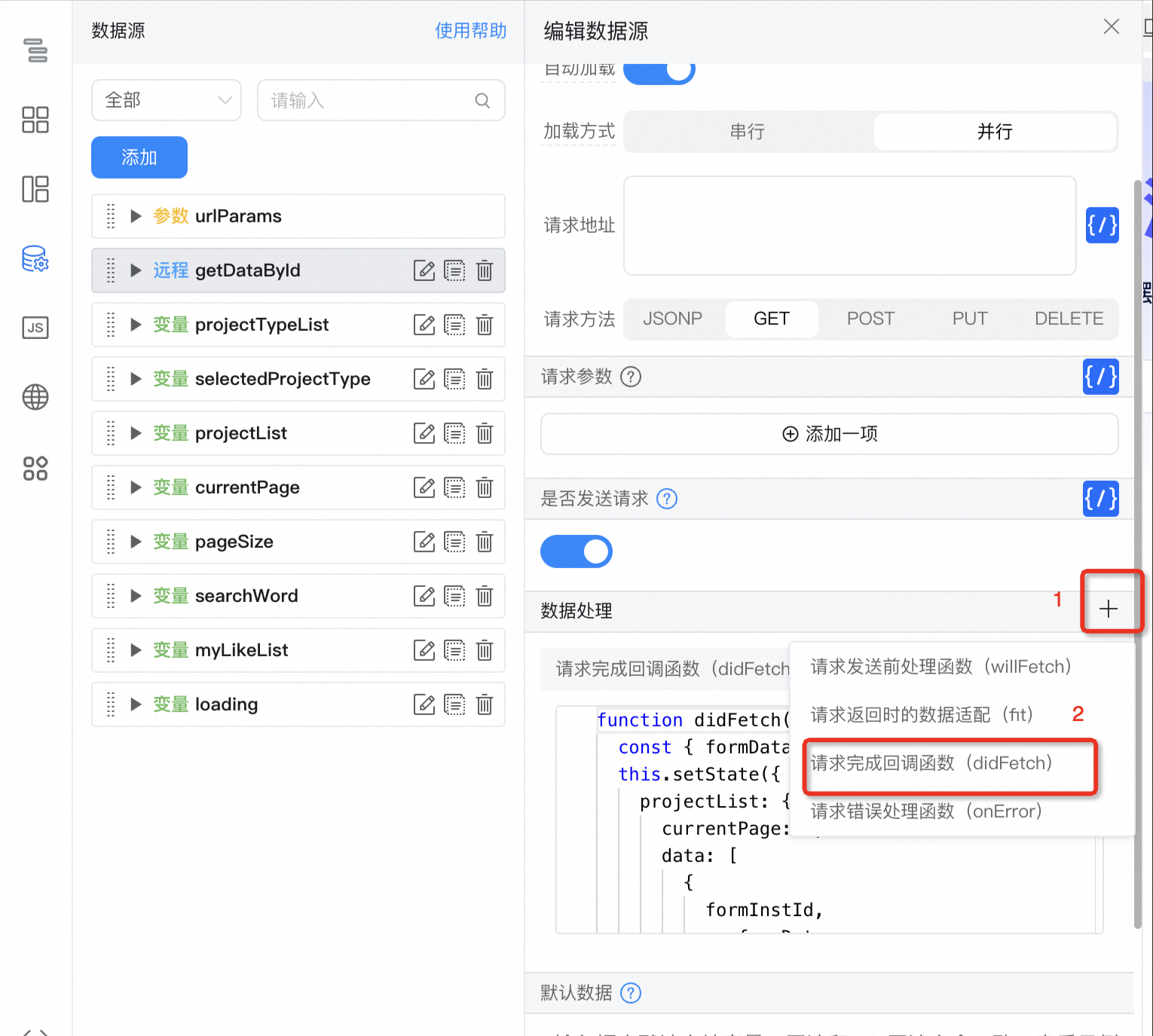
function didFetch(content) {
const { formData = {}, formInstId } = content;
this.setState({
projectList: {
currentPage: 1,
data: [
{
formInstId,
...formData,
},
],
totalCount: 1,
},
searchWord: formData.textField_lbef2r08,
loading: false,
});
return {
formInstId,
...formData,
};
}
Configure data source variables
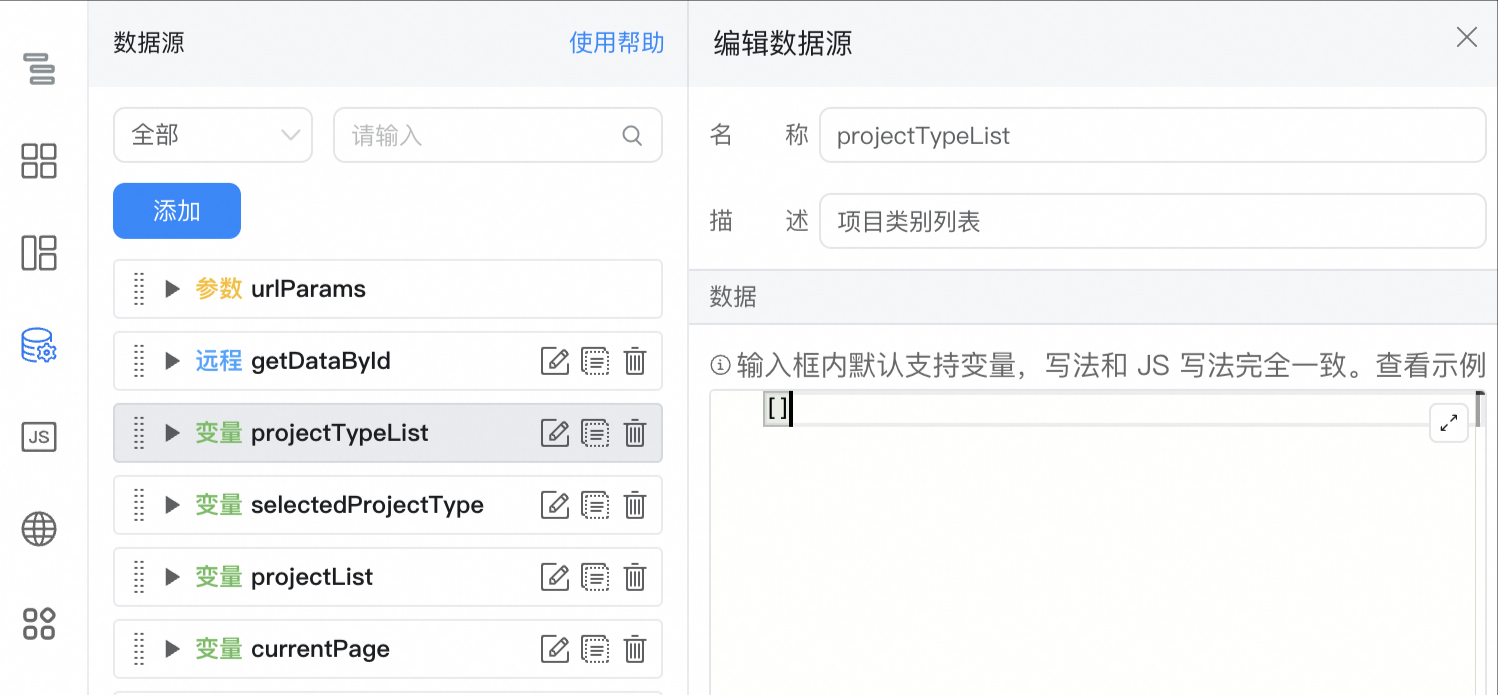
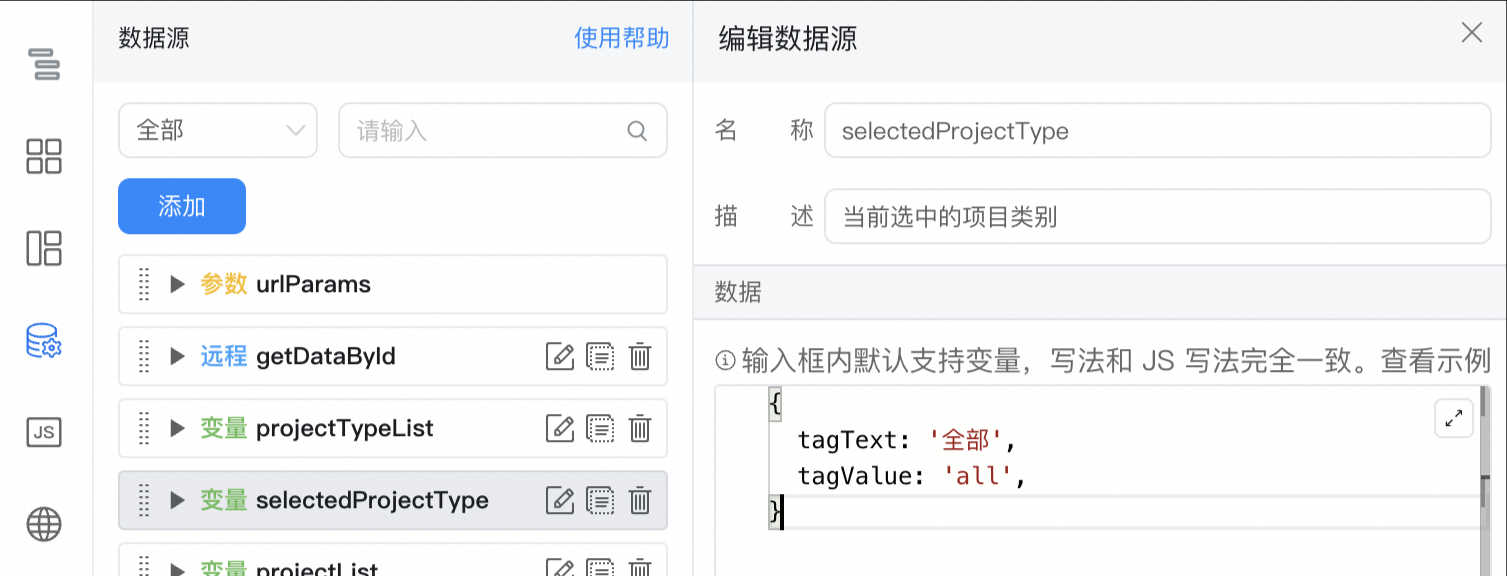

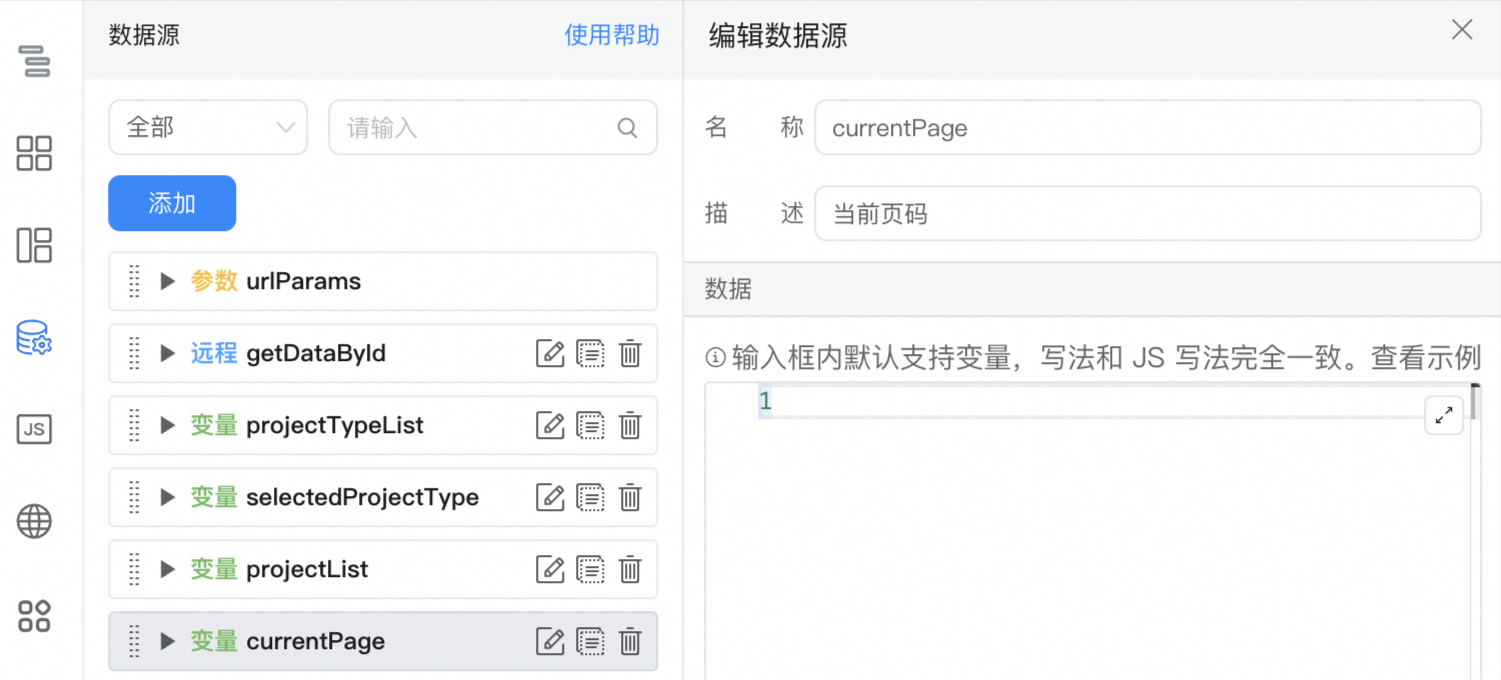
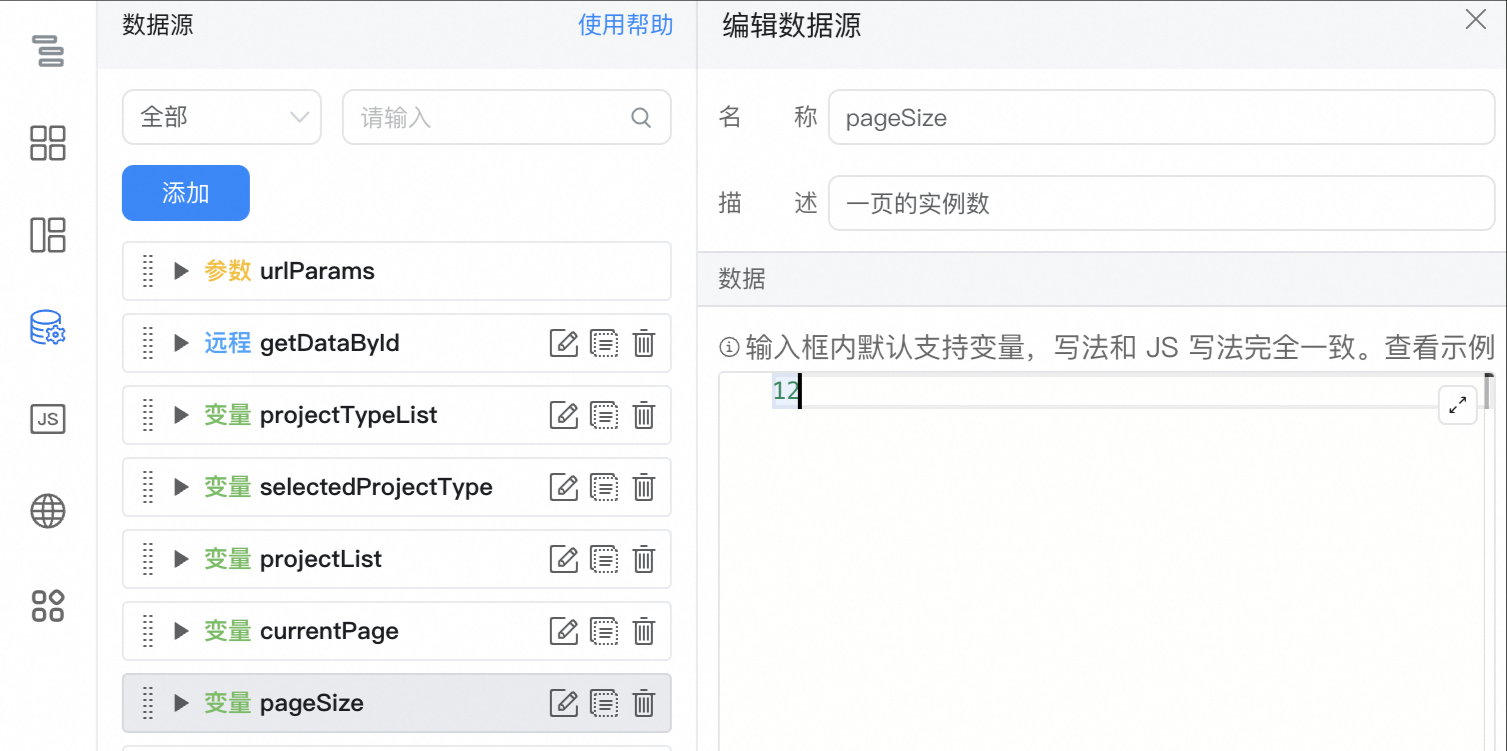
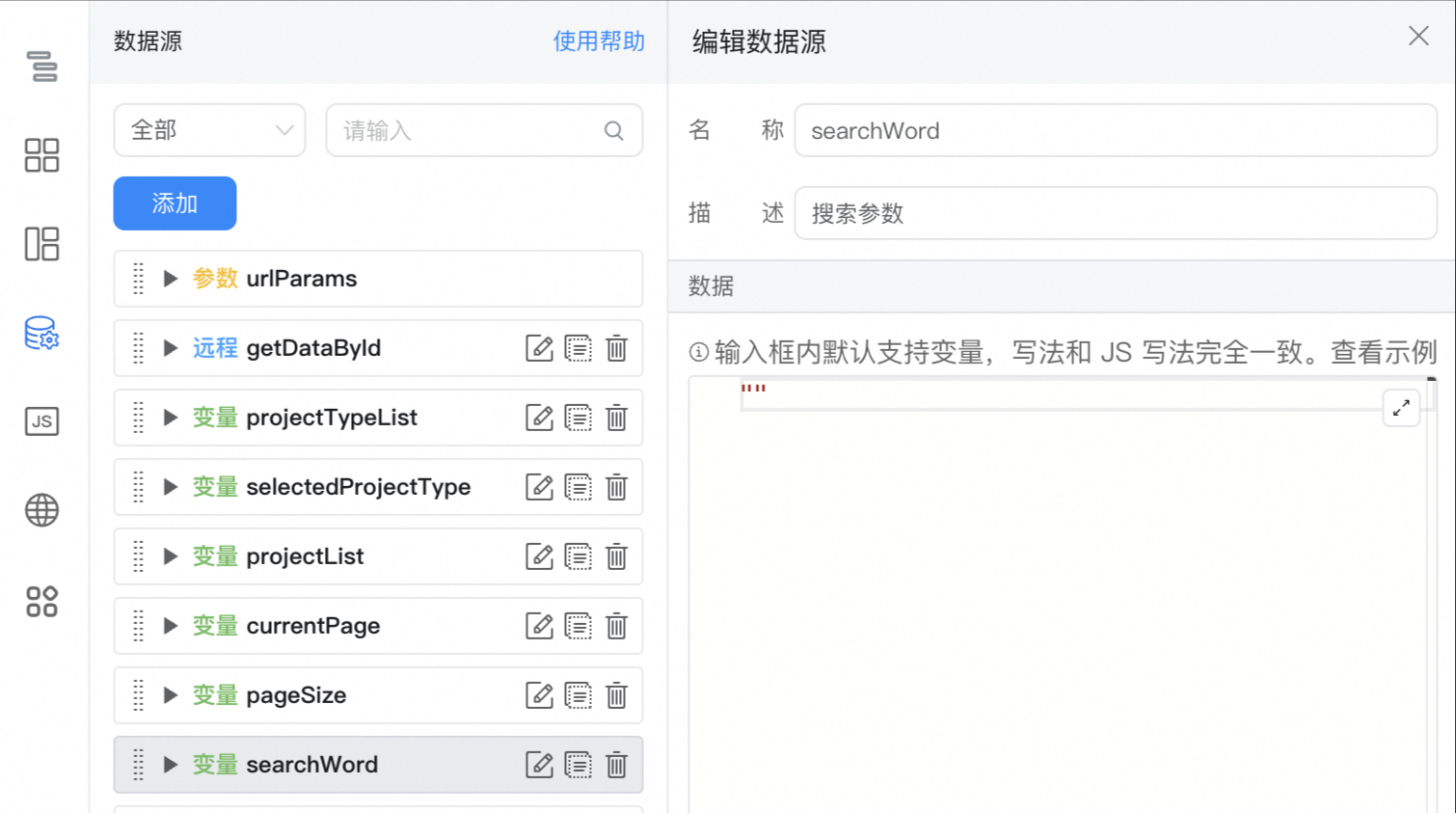
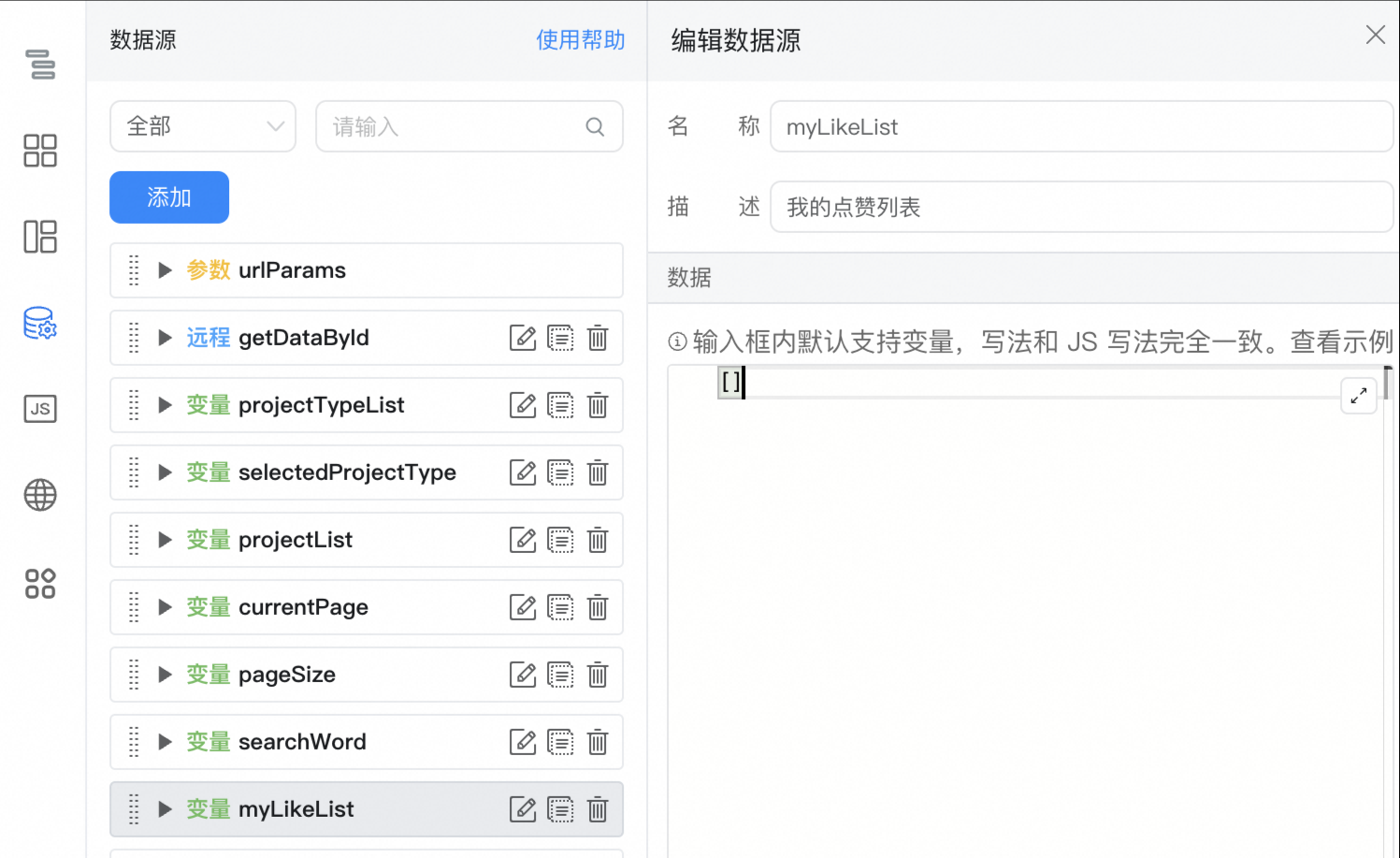
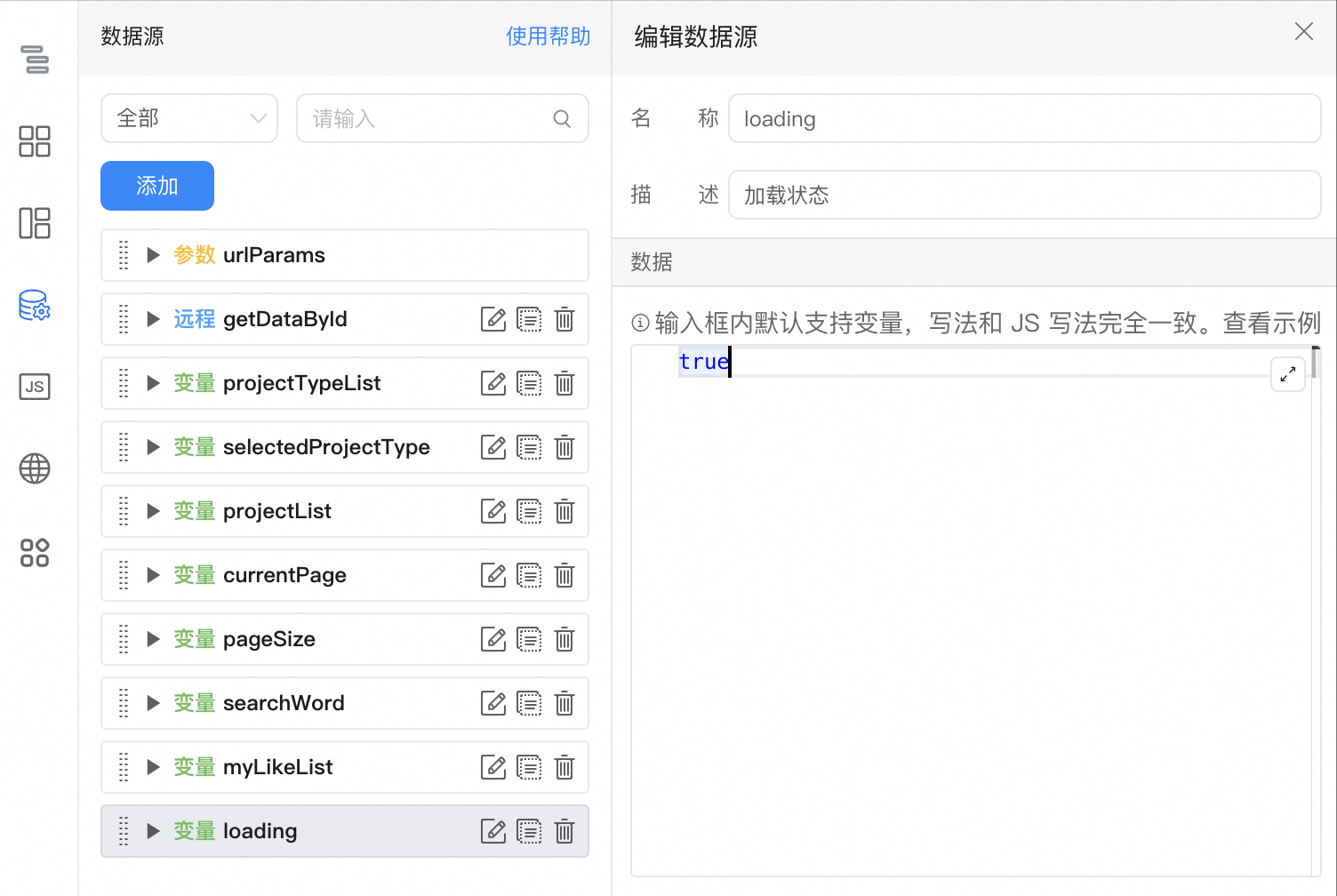
Obtain data
Project category data acquisition
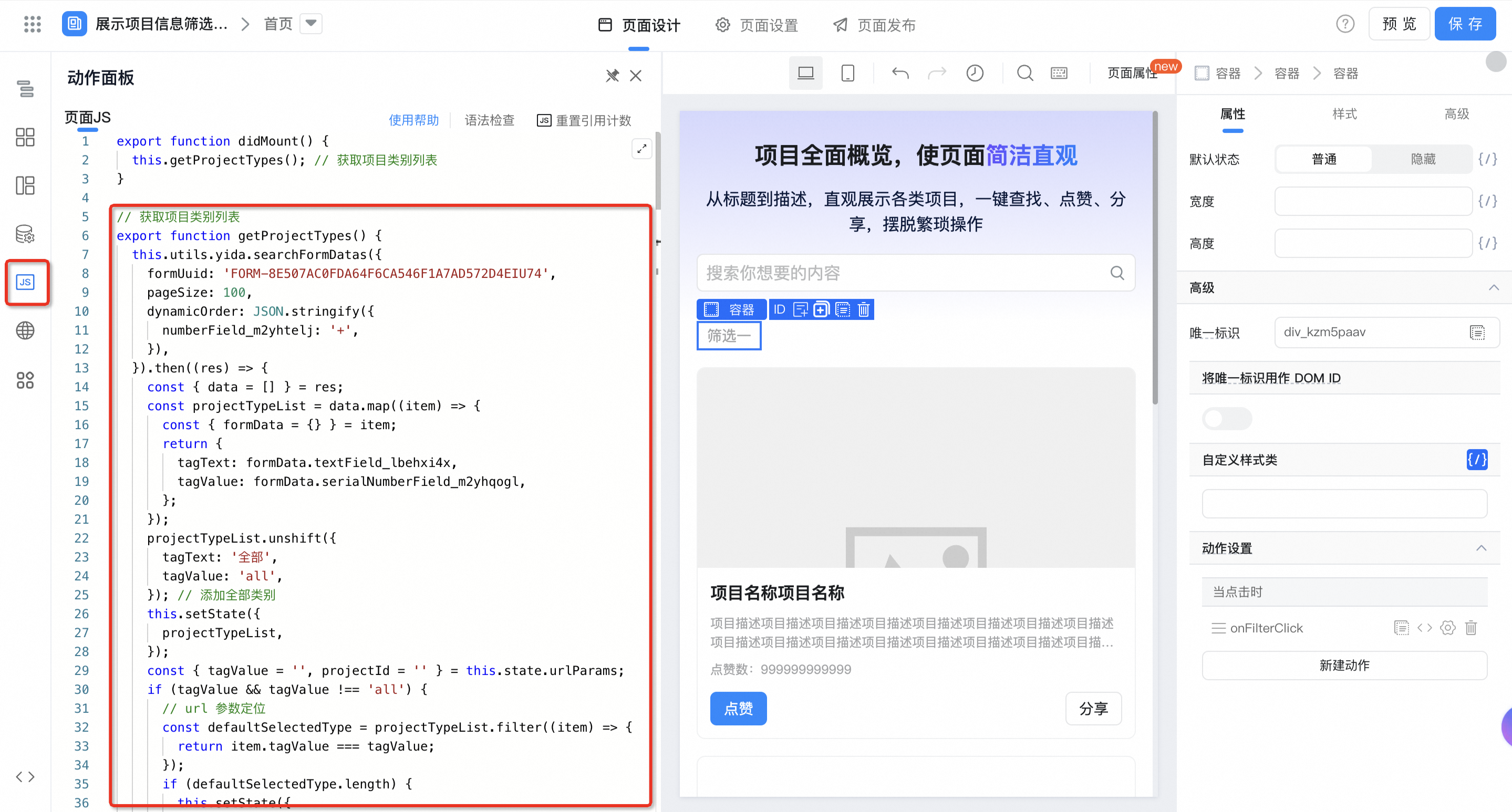
// 获取项目类别列表
export function getProjectTypes() {
this.utils.yida.searchFormDatas({
formUuid: 'FORM-8E507AC0FDA64F6CA546F1A7AD572D4EIU74',
pageSize: 100,
dynamicOrder: JSON.stringify({
numberField_m2yhtelj: '+',
}),
}).then((res) => {
const { data = [] } = res;
const projectTypeList = data.map((item) => {
const { formData = {} } = item;
return {
tagText: formData.textField_lbehxi4x,
tagValue: formData.serialNumberField_m2yhqogl,
};
});
projectTypeList.unshift({
tagText: '全部',
tagValue: 'all',
}); // 添加全部类别
this.setState({
projectTypeList,
});
const { tagValue = '', projectId = '' } = this.state.urlParams;
if (tagValue && tagValue !== 'all') {
// url 参数定位
const defaultSelectedType = projectTypeList.filter((item) => {
return item.tagValue === tagValue;
});
if (defaultSelectedType.length) {
this.setState({
selectedProjectType: defaultSelectedType[0],
});
}
}
if (!projectId) {
this.getProjectList(); // 获取项目列表
}
this.getMyLikeList(); // 获取我的点赞列表
}).catch(({ message }) => {
this.utils.toast({
title: message,
type: 'error',
});
});
}
Get project list data
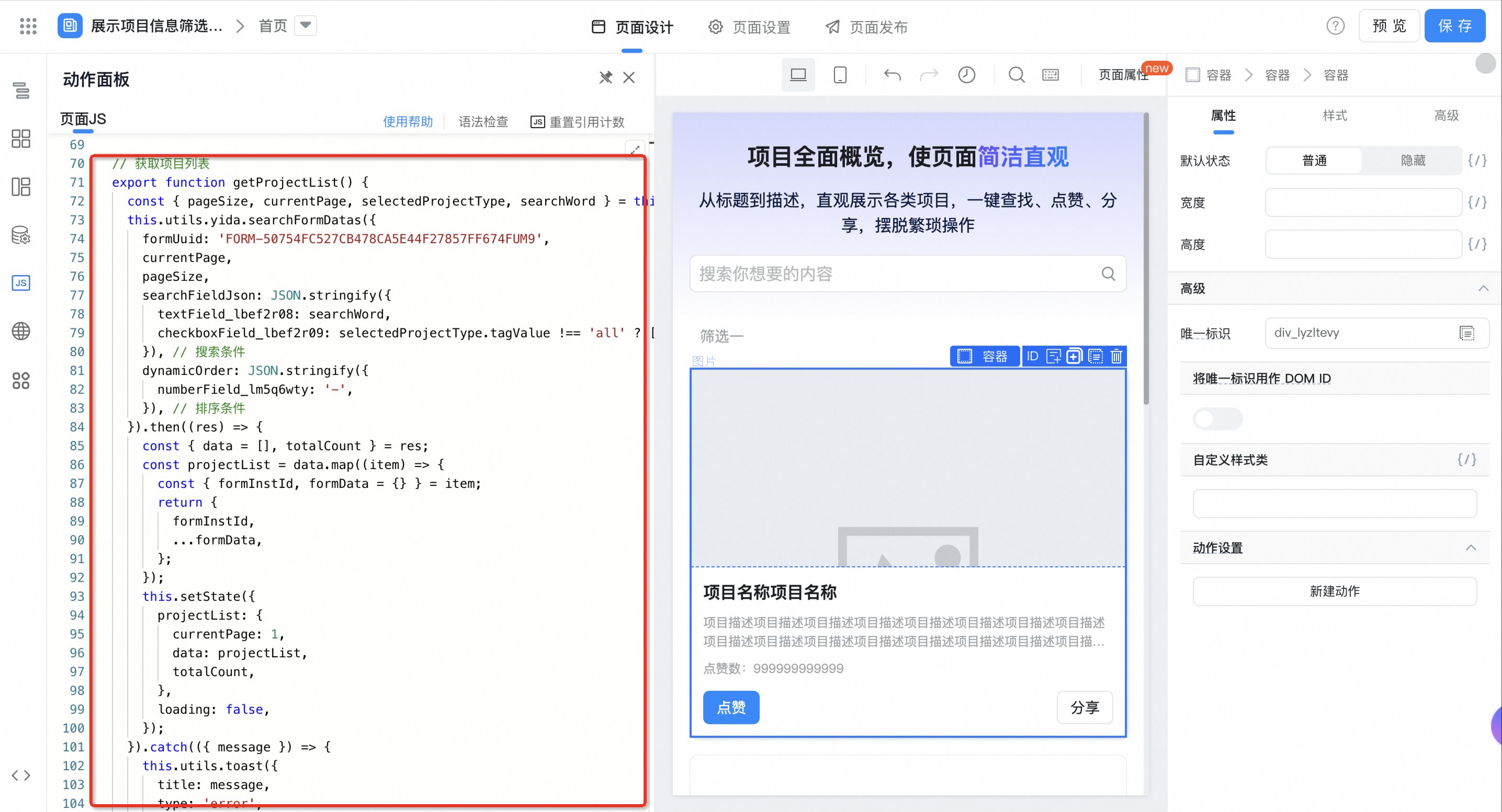
// 获取项目列表
export function getProjectList() {
const { pageSize, currentPage, selectedProjectType, searchWord } = this.state;
this.utils.yida.searchFormDatas({
formUuid: 'FORM-50754FC527CB478CA5E44F27857FF674FUM9',
currentPage,
pageSize,
searchFieldJson: JSON.stringify({
textField_lbef2r08: searchWord,
checkboxField_lbef2r09: selectedProjectType.tagValue !== 'all' ? [selectedProjectType.tagText] : '',
}), // 搜索条件
dynamicOrder: JSON.stringify({
numberField_lm5q6wty: '-',
}), // 排序条件
}).then((res) => {
const { data = [], totalCount } = res;
const projectList = data.map((item) => {
const { formInstId, formData = {} } = item;
return {
formInstId,
...formData,
};
});
this.setState({
projectList: {
currentPage: 1,
data: projectList,
totalCount,
},
loading: false,
});
}).catch(({ message }) => {
this.utils.toast({
title: message,
type: 'error',
});
this.setState({
loading: false,
});
});
}
Click like record data acquisition
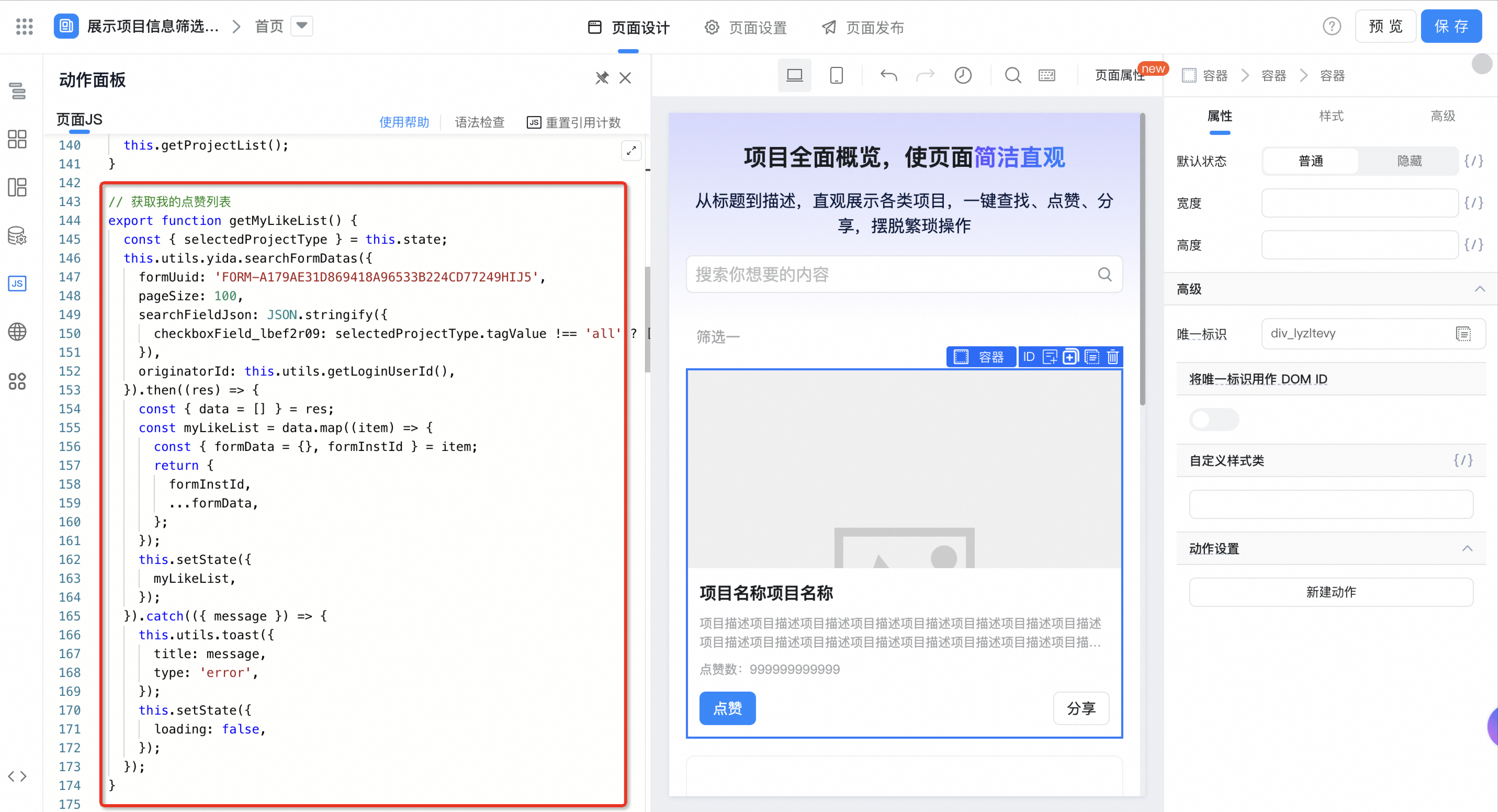
// 获取我的点赞列表
export function getMyLikeList() {
const { selectedProjectType } = this.state;
this.utils.yida.searchFormDatas({
formUuid: 'FORM-A179AE31D869418A96533B224CD77249HIJ5',
pageSize: 100,
searchFieldJson: JSON.stringify({
checkboxField_lbef2r09: selectedProjectType.tagValue !== 'all' ? [selectedProjectType.tagText] : '',
}),
originatorId: this.utils.getLoginUserId(),
}).then((res) => {
const { data = [] } = res;
const myLikeList = data.map((item) => {
const { formData = {}, formInstId } = item;
return {
formInstId,
...formData,
};
});
this.setState({
myLikeList,
});
}).catch(({ message }) => {
this.utils.toast({
title: message,
type: 'error',
});
this.setState({
loading: false,
});
});
}
Configure custom page display
Page layout outline tree configuration
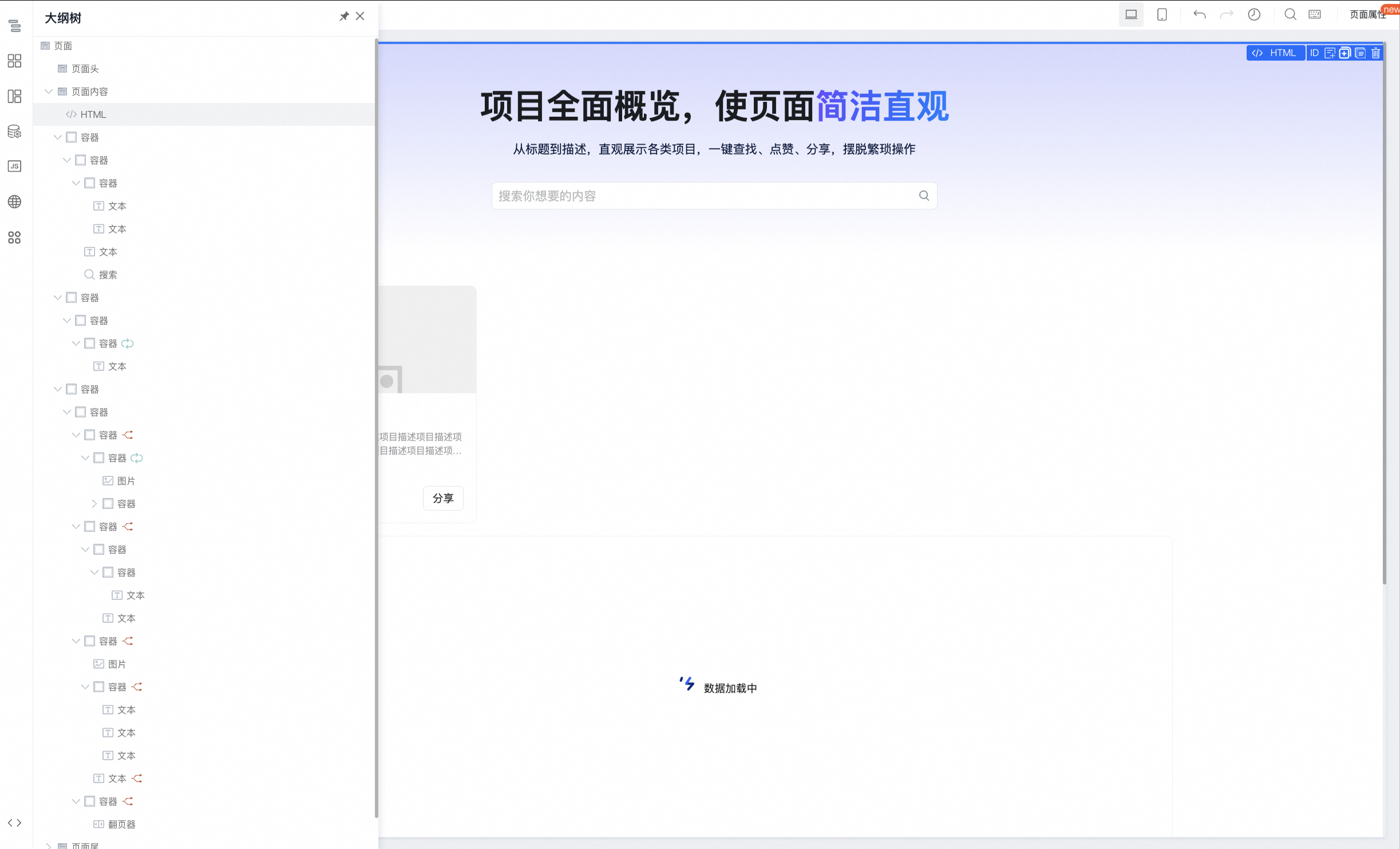
Project category circular data binding

Project category custom style class to control the selection effect:
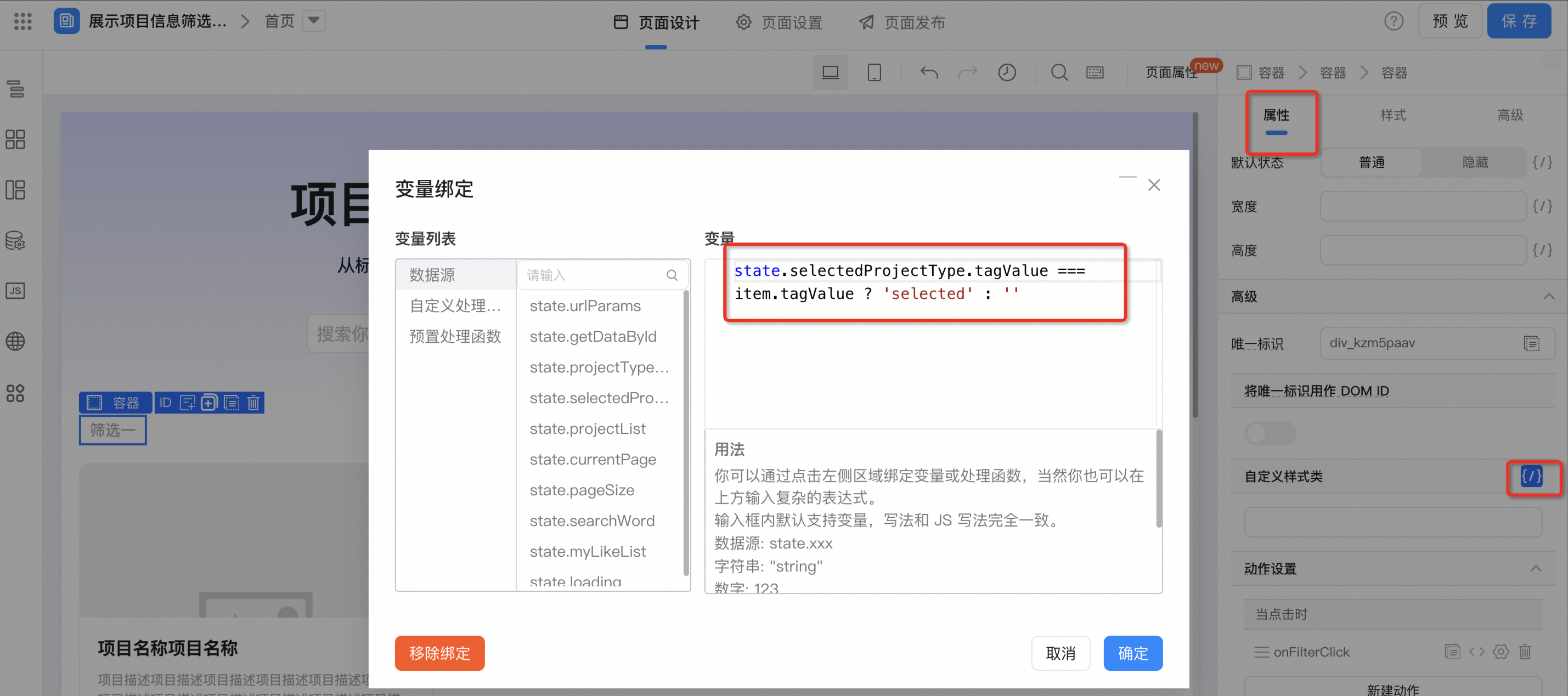
state.selectedProjectType.tagValue === item.tagValue ? 'selected' : ''
Project category text content binding
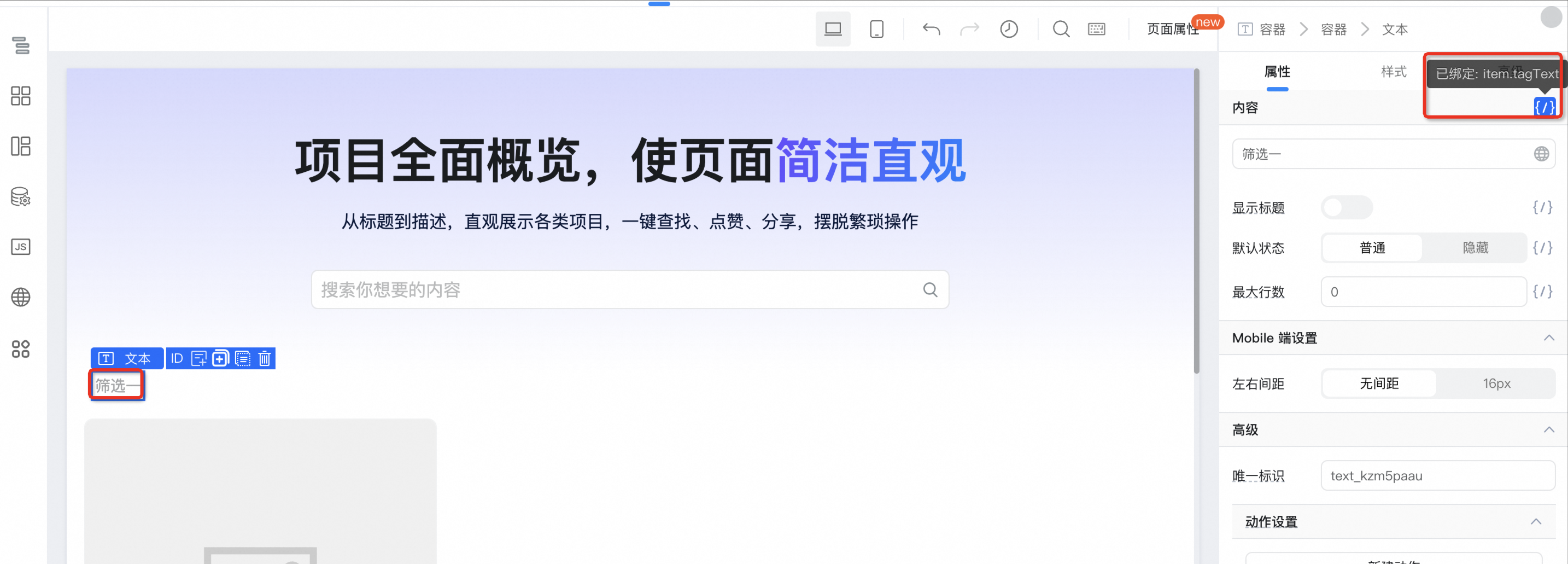
Project information list circular data binding
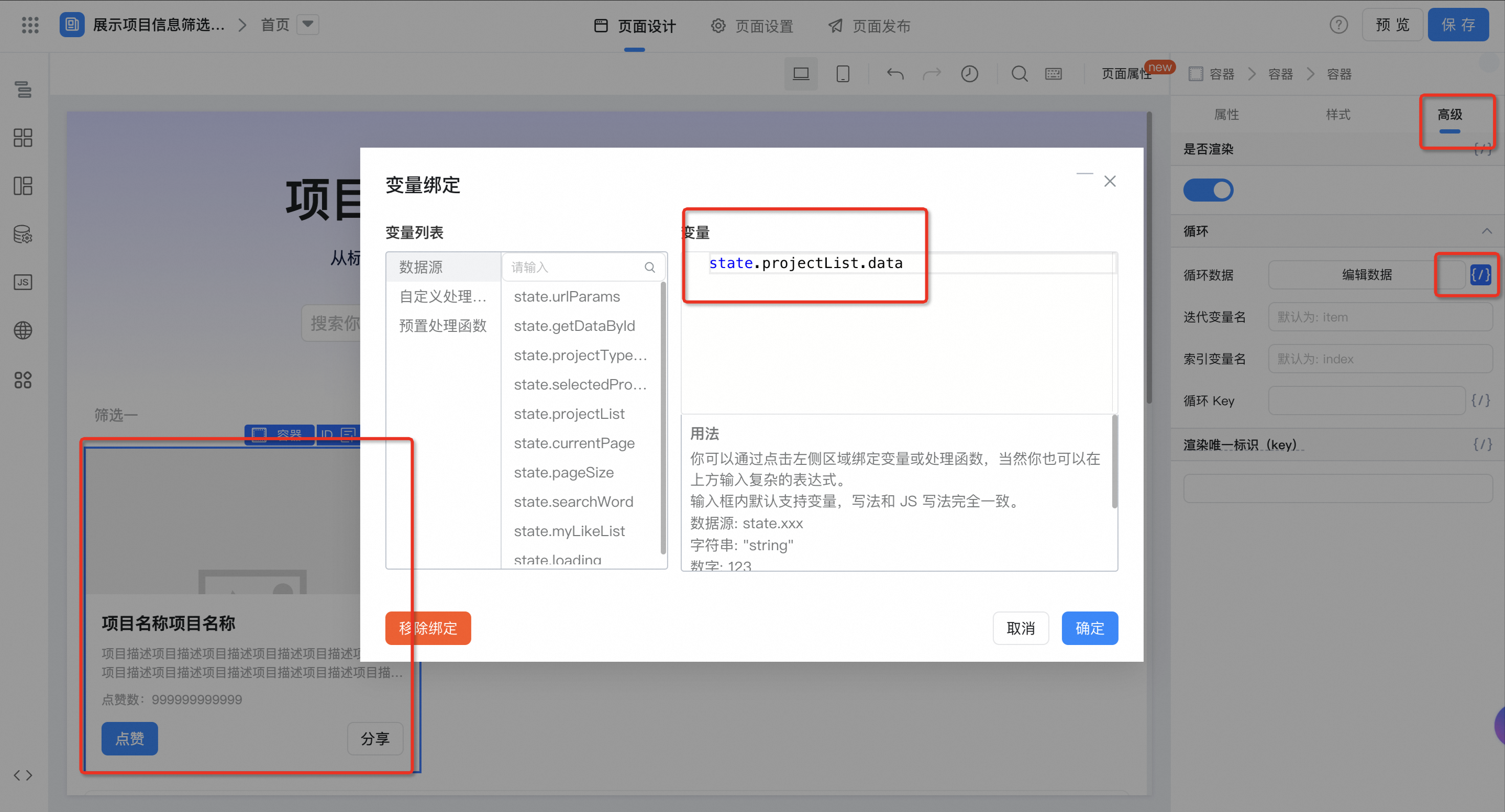
state.projectList.data
Add Event binding
Project category filtering configuration
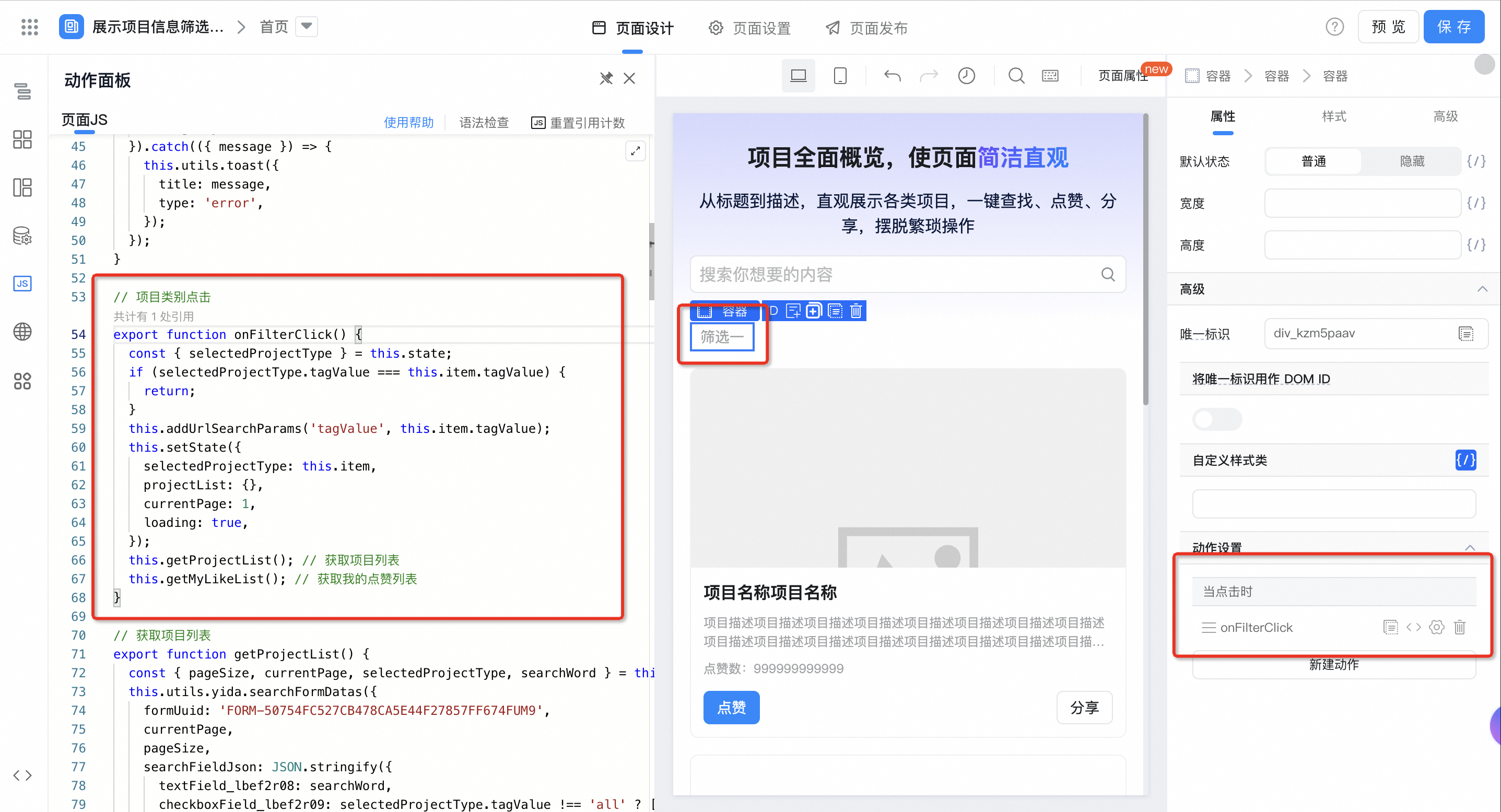
// 项目类别点击
export function onFilterClick() {
const { selectedProjectType } = this.state;
if (selectedProjectType.tagValue === this.item.tagValue) {
return;
}
this.addUrlSearchParams('tagValue', this.item.tagValue);
this.setState({
selectedProjectType: this.item,
projectList: {},
currentPage: 1,
loading: true,
});
this.getProjectList(); // 获取项目列表
this.getMyLikeList(); // 获取我的点赞列表
}
/**
* 新增&更新 URL 查询参数
* @param key {String} 需要新增&更新的参数名称
* @param value {String} 需要新增&更新的参数值
*/
export function addUrlSearchParams(key, value) {
const newUrl = new URL(window.location.href);
newUrl.searchParams.set(key, value);
window.history.pushState({ path: newUrl.href }, '', newUrl.href);
}
Search box configuration
Bind the default variable of the search box
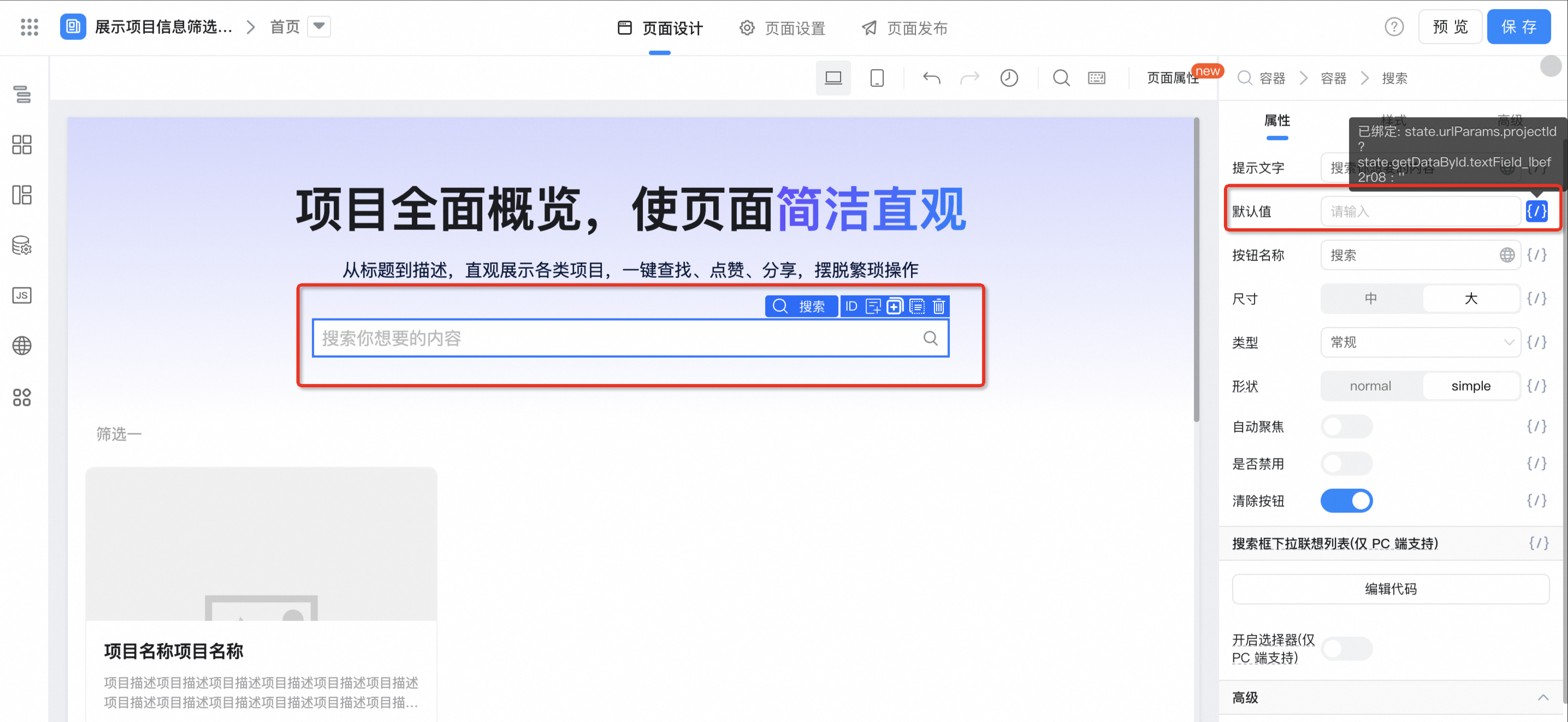
state.urlParams.projectId ? state.getDataById.textField_lbef2r08 : ''
Bind search box events
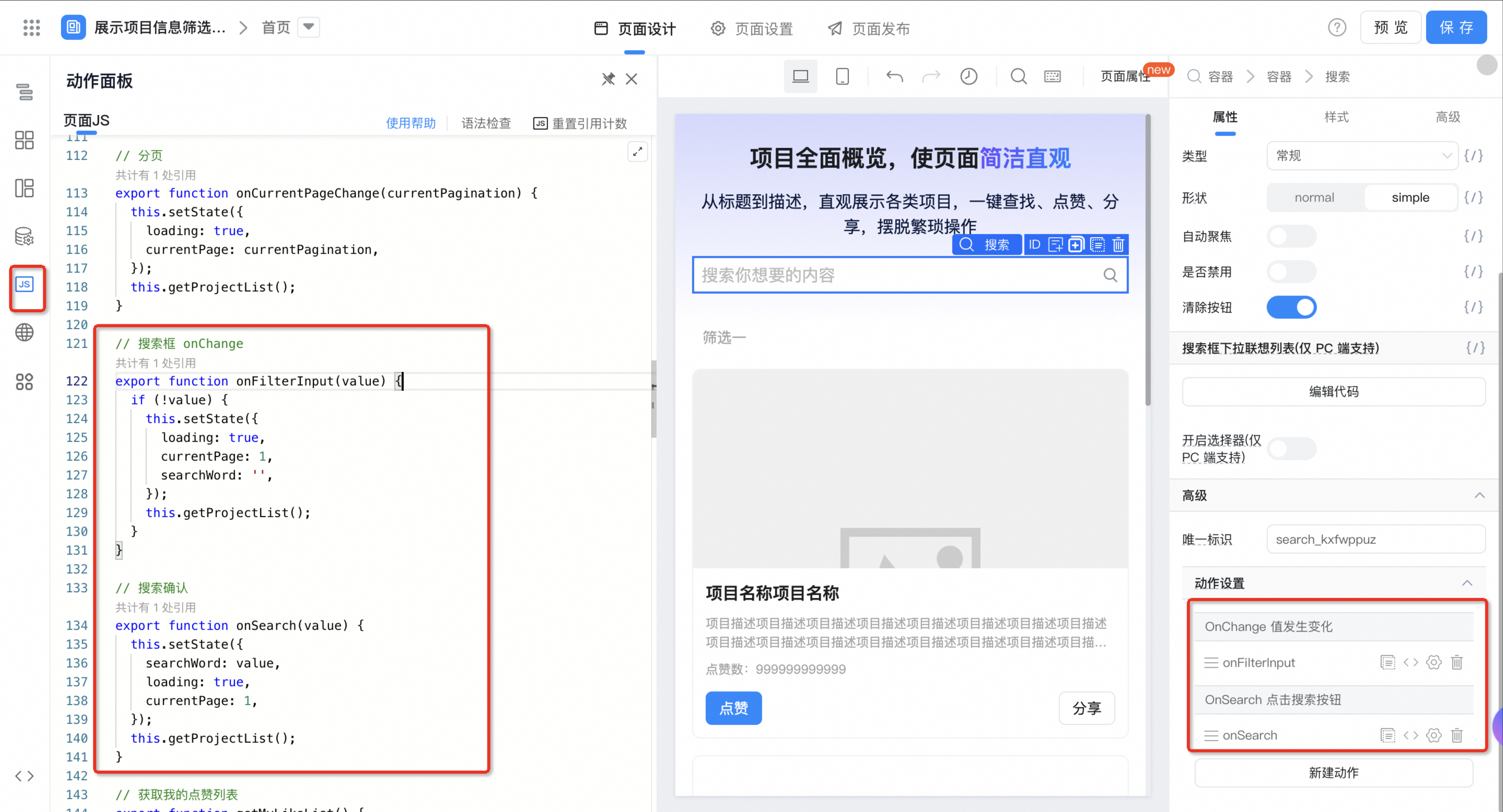
// 搜索框 onChange
export function onFilterInput(value) {
if (!value) {
this.setState({
loading: true,
currentPage: 1,
searchWord: '',
});
this.getProjectList();
}
}
// 搜索确认
export function onSearch(value) {
this.setState({
searchWord: value,
loading: true,
currentPage: 1,
});
this.getProjectList();
}
Like configuration
Click Like button to bind variables
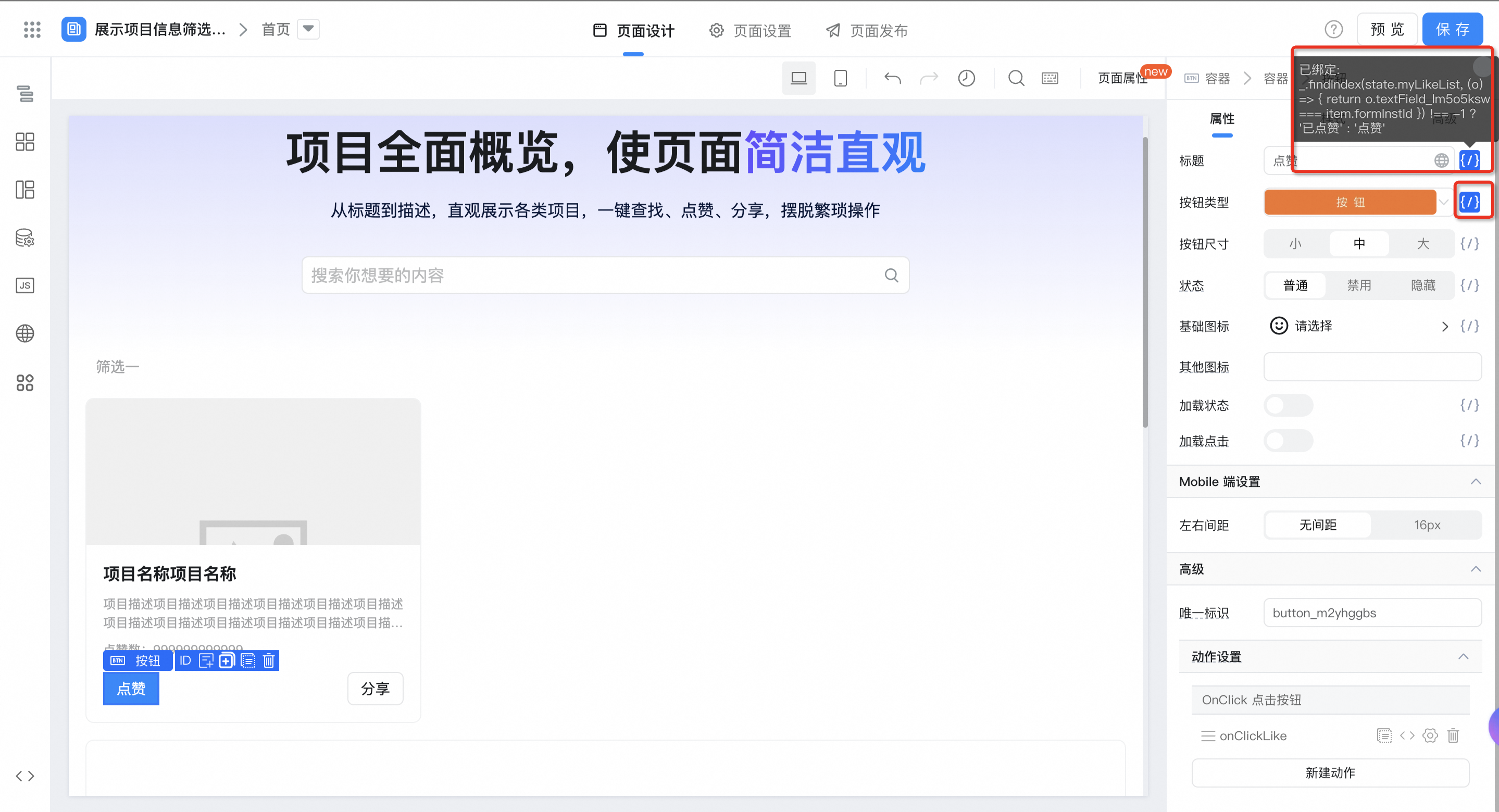
_.findIndex(state.myLikeList, (o) => { return o.textField_lm5o5ksw === item.formInstId }) !== -1 ? '已点赞' : '点赞'
_.findIndex(state.myLikeList, (o) => { return o.textField_lm5o5ksw === item.formInstId }) !== -1 ? 'normal' : 'primary'
Bind like events
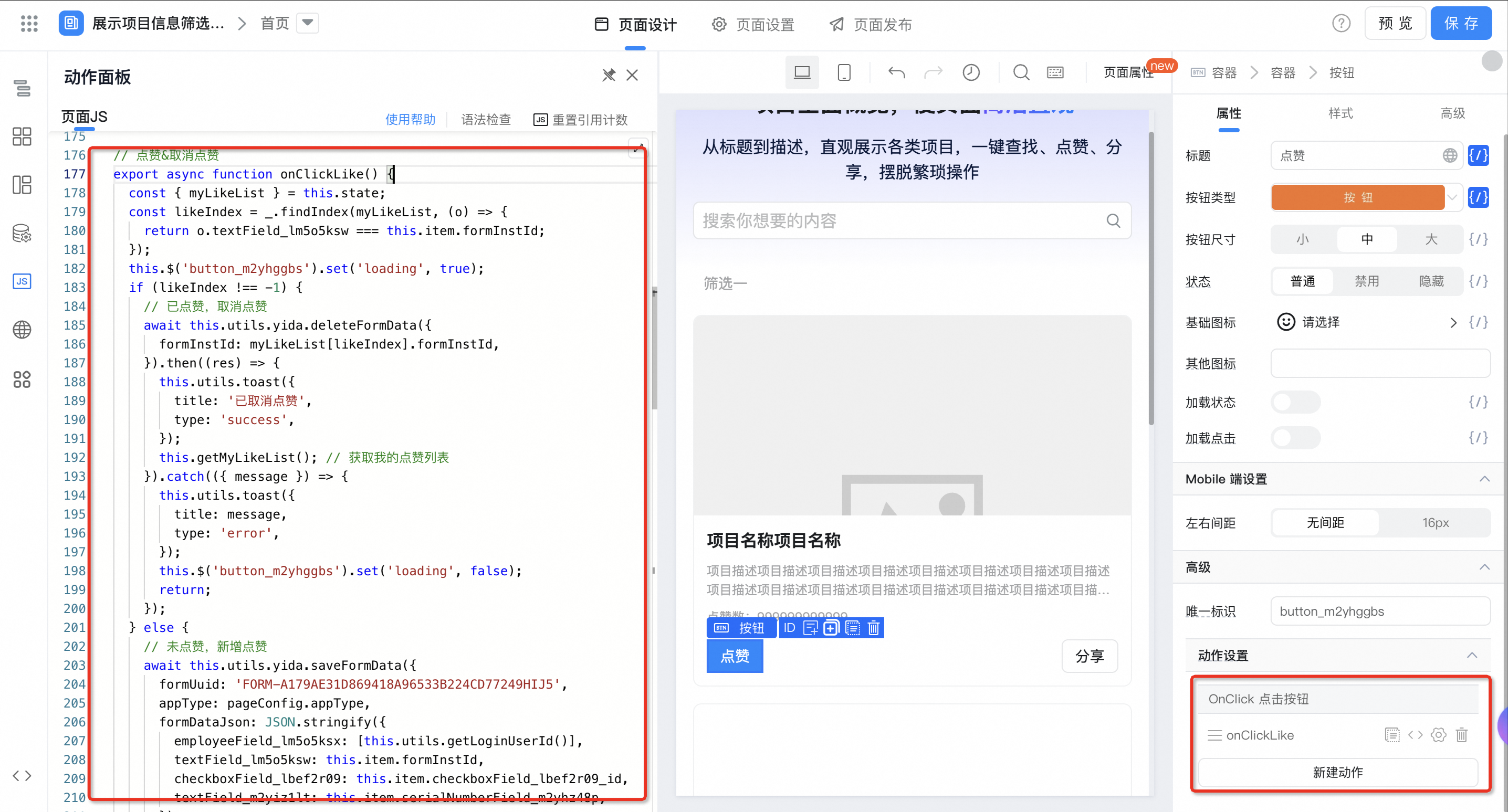
// 点赞&取消点赞
export async function onClickLike() {
const { myLikeList } = this.state;
const likeIndex = _.findIndex(myLikeList, (o) => {
return o.textField_lm5o5ksw === this.item.formInstId;
});
this.$('button_m2yhggbs').set('loading', true);
if (likeIndex !== -1) {
// 已点赞,取消点赞
await this.utils.yida.deleteFormData({
formInstId: myLikeList[likeIndex].formInstId,
}).then((res) => {
this.utils.toast({
title: '已取消点赞',
type: 'success',
});
this.getMyLikeList(); // 获取我的点赞列表
}).catch(({ message }) => {
this.utils.toast({
title: message,
type: 'error',
});
this.$('button_m2yhggbs').set('loading', false);
return;
});
} else {
// 未点赞,新增点赞
await this.utils.yida.saveFormData({
formUuid: 'FORM-A179AE31D869418A96533B224CD77249HIJ5',
appType: pageConfig.appType,
formDataJson: JSON.stringify({
employeeField_lm5o5ksx: [this.utils.getLoginUserId()],
textField_lm5o5ksw: this.item.formInstId,
checkboxField_lbef2r09: this.item.checkboxField_lbef2r09_id,
textField_m2yiz1lt: this.item.serialNumberField_m2yhz48p,
}),
}).then((res) => {
this.utils.toast({
title: '点赞成功',
type: 'success',
});
this.getMyLikeList(); // 获取我的点赞列表
}).catch(({ message }) => {
this.utils.toast({
title: message,
type: 'error',
});
this.$('button_m2yhggbs').set('loading', false);
return;
});
}
setTimeout(() => {
// 若出现票数不正确,可按需修改延时器的时间,目前 0 ms
this.utils.yida.searchFormDatas({
formUuid: 'FORM-A179AE31D869418A96533B224CD77249HIJ5',
searchFieldJson: JSON.stringify({
textField_lm5o5ksw: this.item.formInstId,
}), // 搜索条件
}).then((res) => {
const { totalCount = 0 } = res;
const { projectList } = this.state;
projectList.data[this.index].numberField_lm5q6wty = totalCount;
this.setState({
projectList,
}); // 更新 state 数据
// 更新实际数据
this.utils.yida.updateFormData({
formInstId: this.item.formInstId,
updateFormDataJson: JSON.stringify({
numberField_lm5q6wty: totalCount,
}),
useLatestVersion: 'y', // 是否使用最新的表单版本进行更新
}).catch(({ message }) => {
this.utils.toast({
title: message,
type: 'error',
});
});
this.$('button_m2yhggbs').set('loading', false);
}).catch(({ message }) => {
this.utils.toast({
title: message,
type: 'error',
});
this.$('button_m2yhggbs').set('loading', false);
});
}, 0);
}
Share configuration
Share event binding
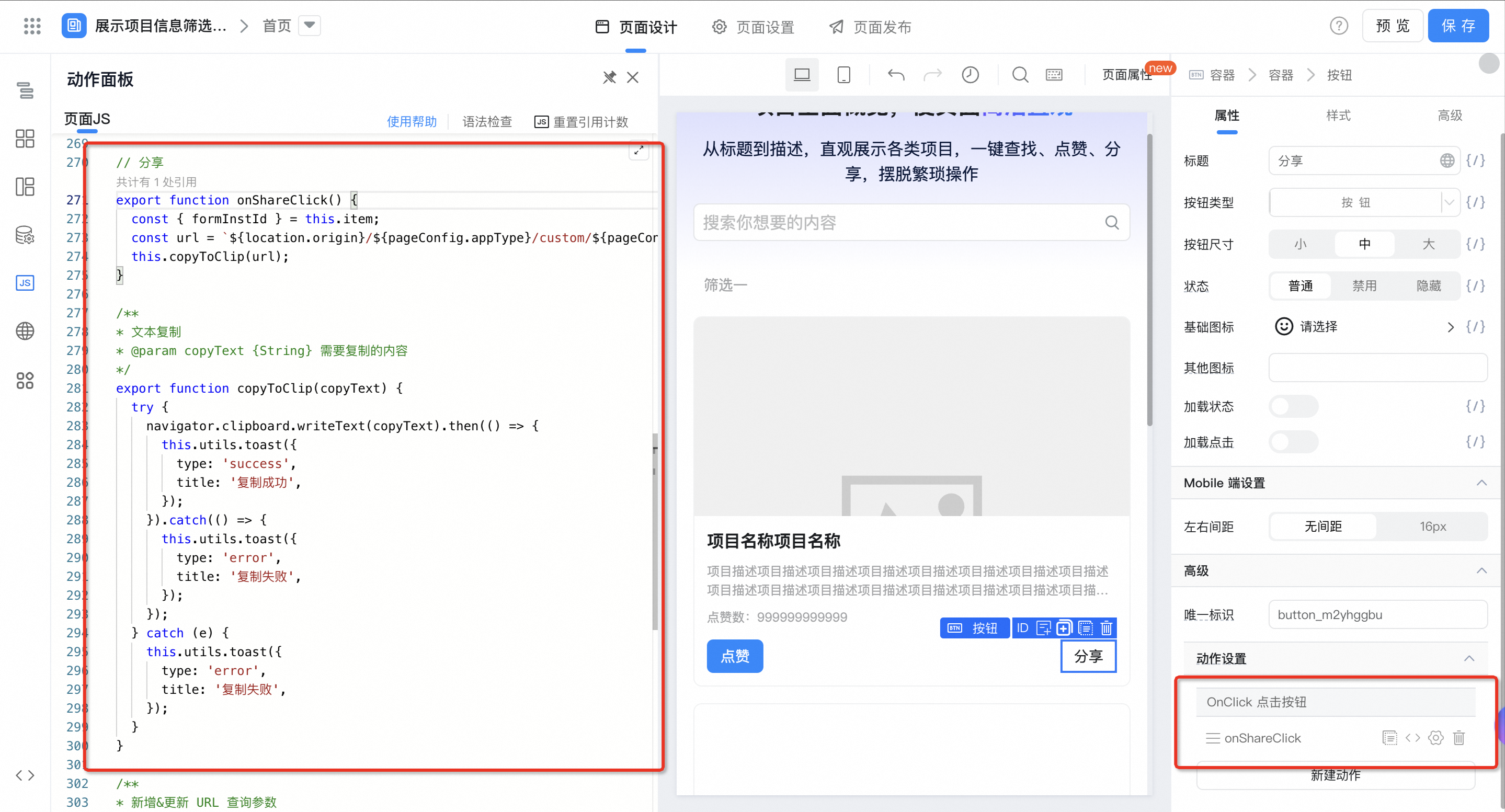
// 分享
export function onShareClick() {
const { formInstId } = this.item;
const url = `${location.origin}/${pageConfig.appType}/custom/${pageConfig.formUuid}?projectId=${formInstId}&corpid=${pageConfig.corpId}&isRenderNav=false`;
this.copyToClip(url);
}
/**
* 文本复制
* @param copyText {String} 需要复制的内容
*/
export function copyToClip(copyText) {
try {
navigator.clipboard.writeText(copyText).then(() => {
this.utils.toast({
type: 'success',
title: '复制成功',
});
}).catch(() => {
this.utils.toast({
type: 'error',
title: '复制失败',
});
});
} catch (e) {
this.utils.toast({
type: 'error',
title: '复制失败',
});
}
}
Pagination configuration
Pagination variable binding
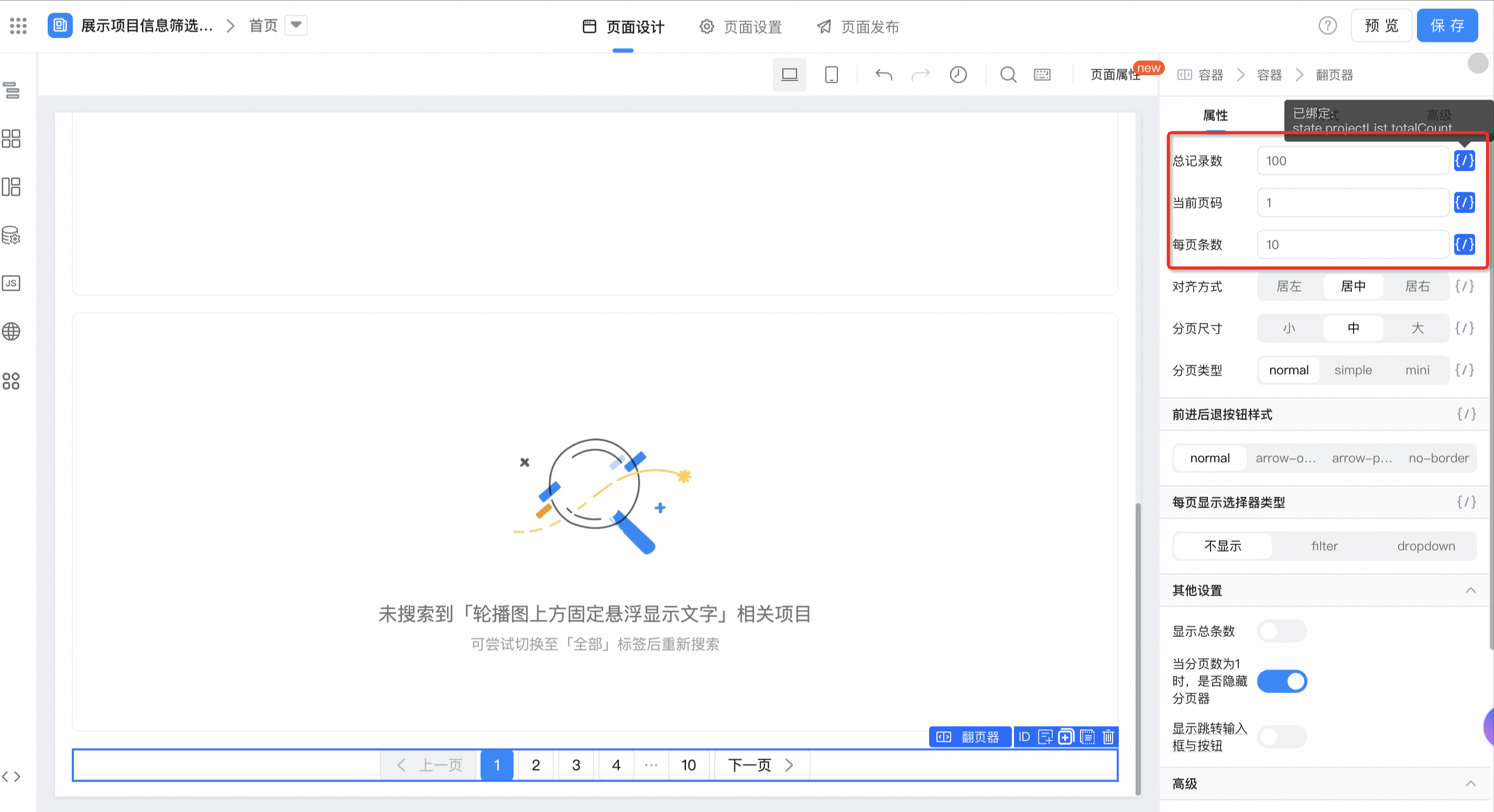
state.projectList.totalCount
state.currentPage
state.pageSize
Pagination event binding
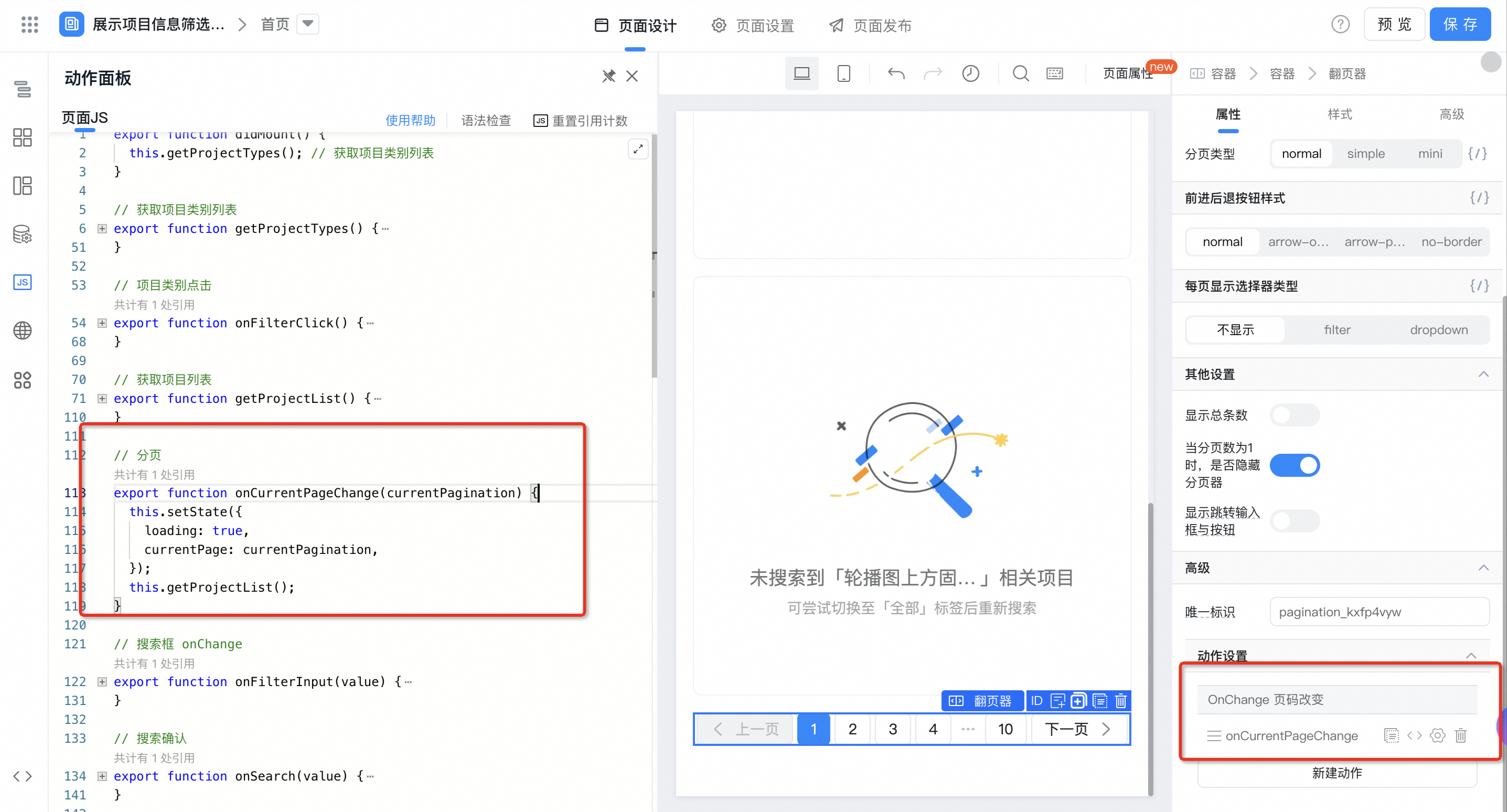
// 分页
export function onCurrentPageChange(currentPagination) {
this.setState({
loading: true,
currentPage: currentPagination,
});
this.getProjectList();
}
Image preview configuration
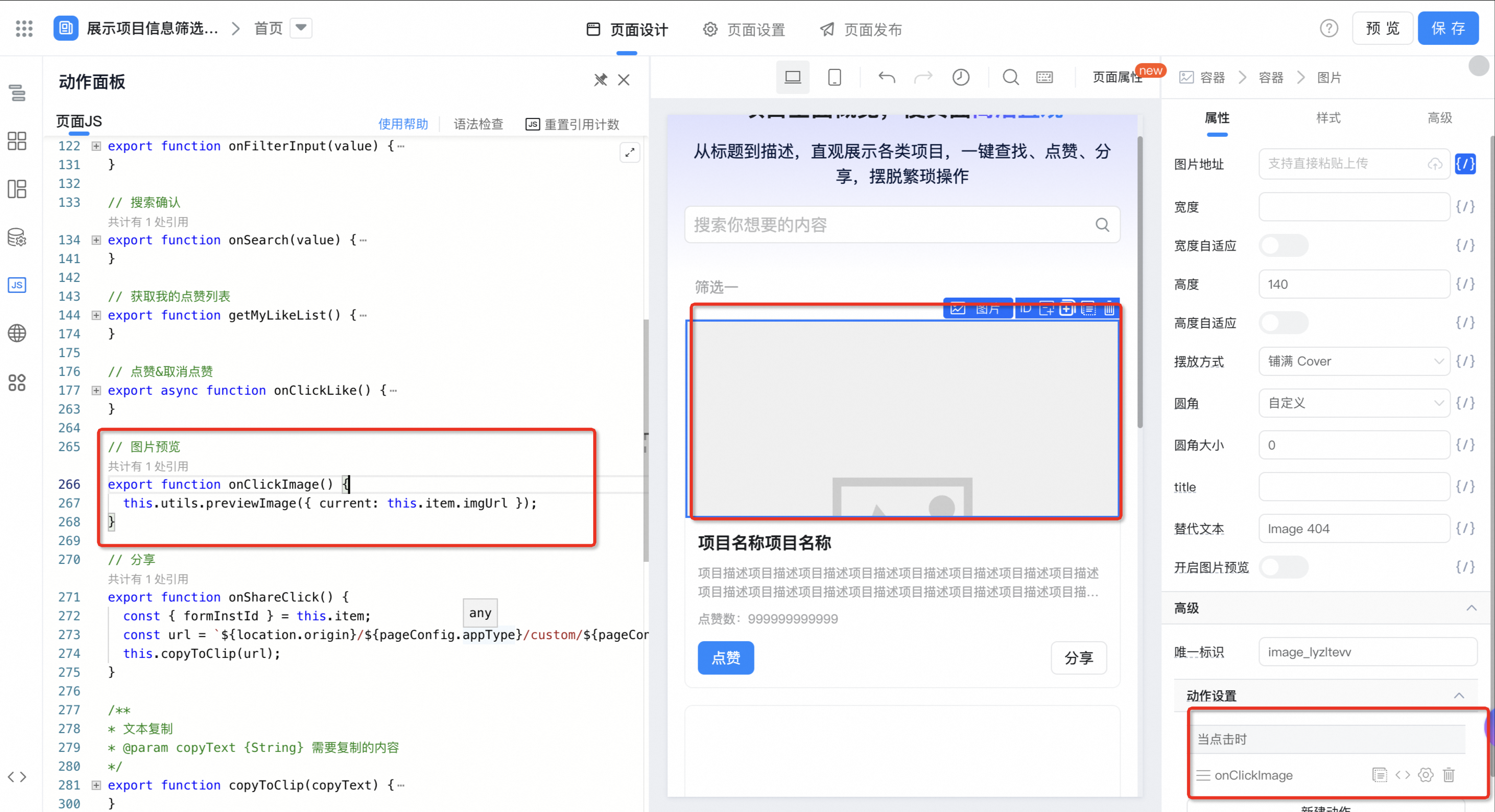
// 图片预览
export function onClickImage() {
this.utils.previewImage({ current: this.item.imgUrl });
}