Custom page embedded in AMAP map
1. Usage scenarios
This example describes how to use AMAP on the YIDA custom page.
2. Implement functions
2.1. Apply for AMAP open platform JSAPI Key
2.1.1. LoginAMAP open platform
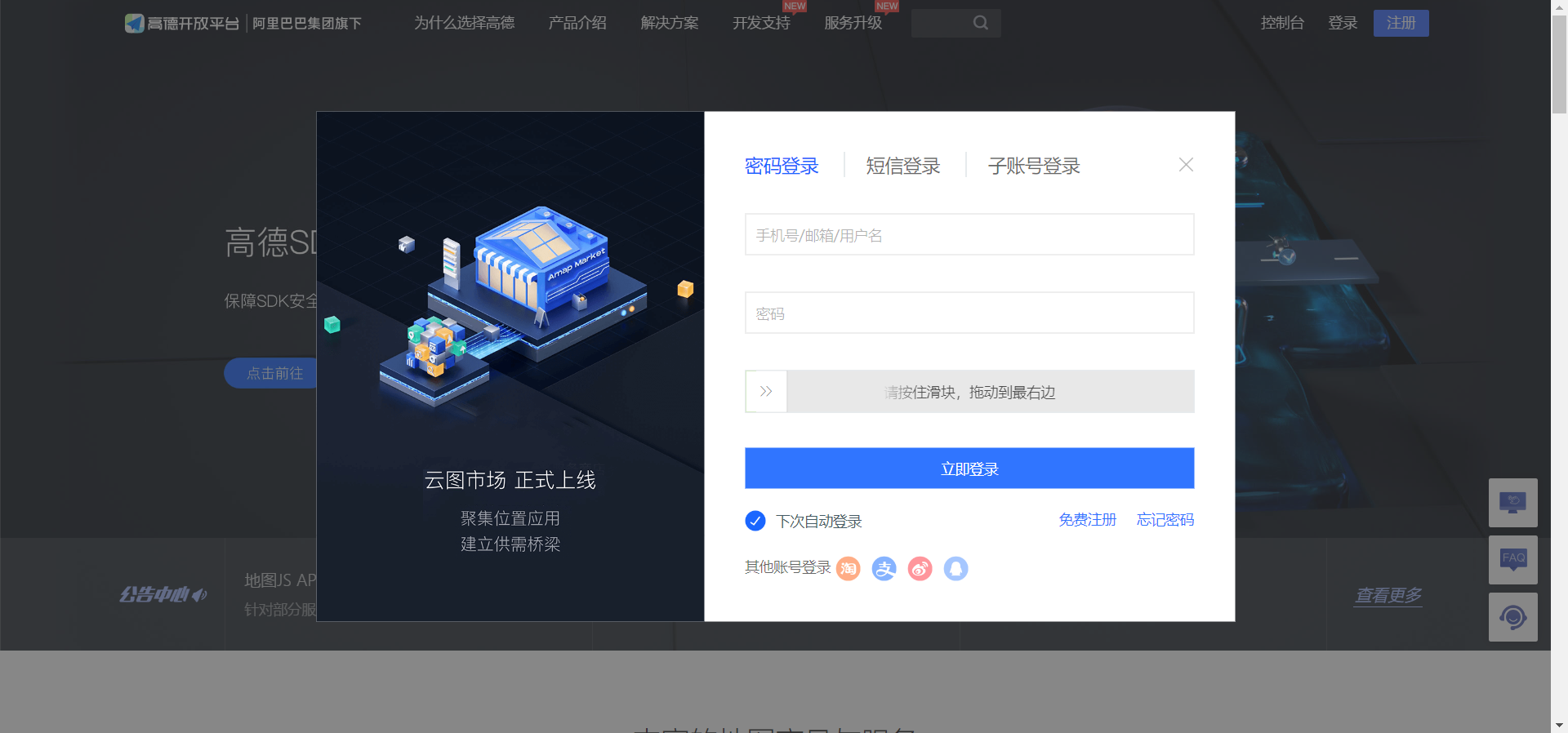
After logging in, click console in the upper-right corner to enter the console.
2.1.2. Account authentication

2.1.3. Manage (create) keys

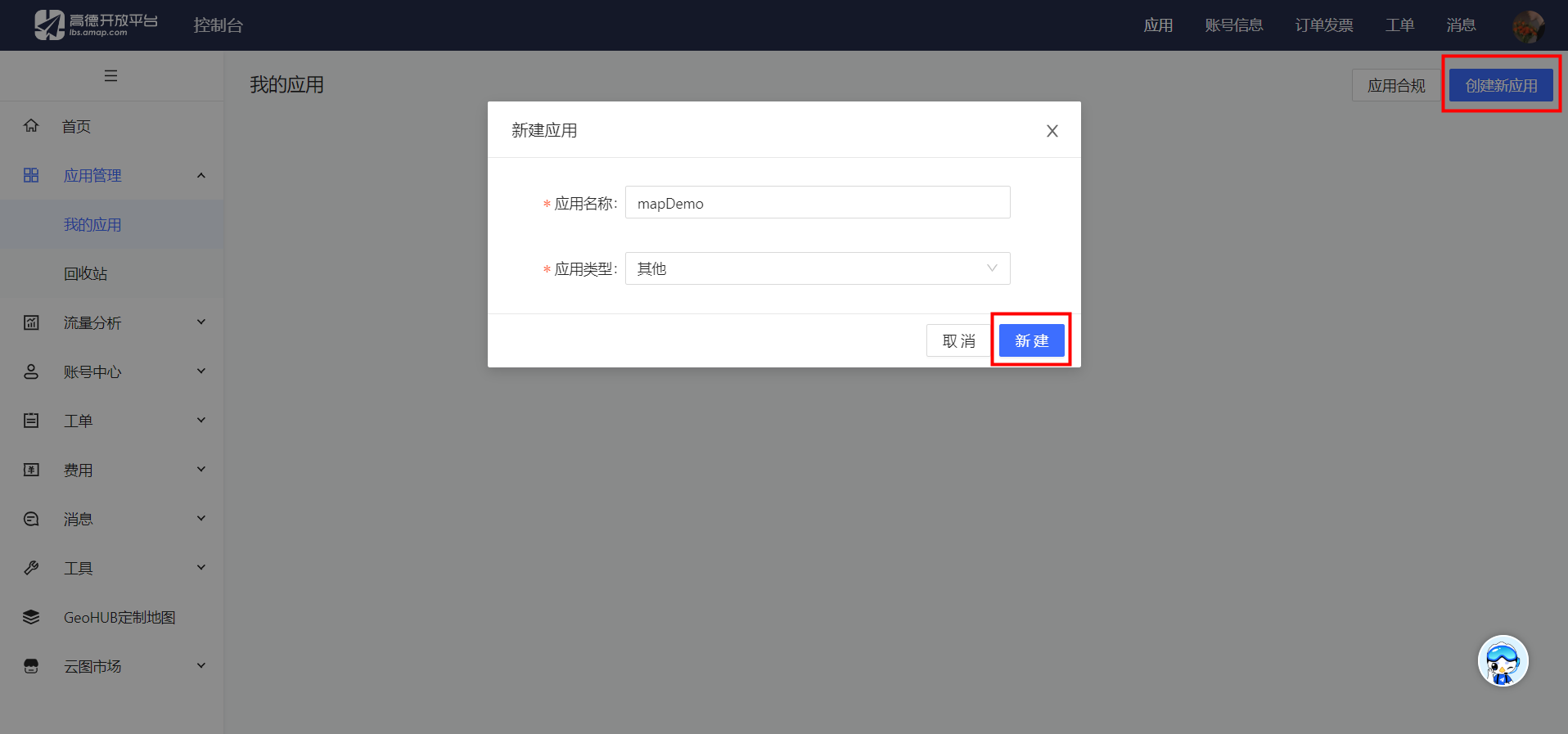
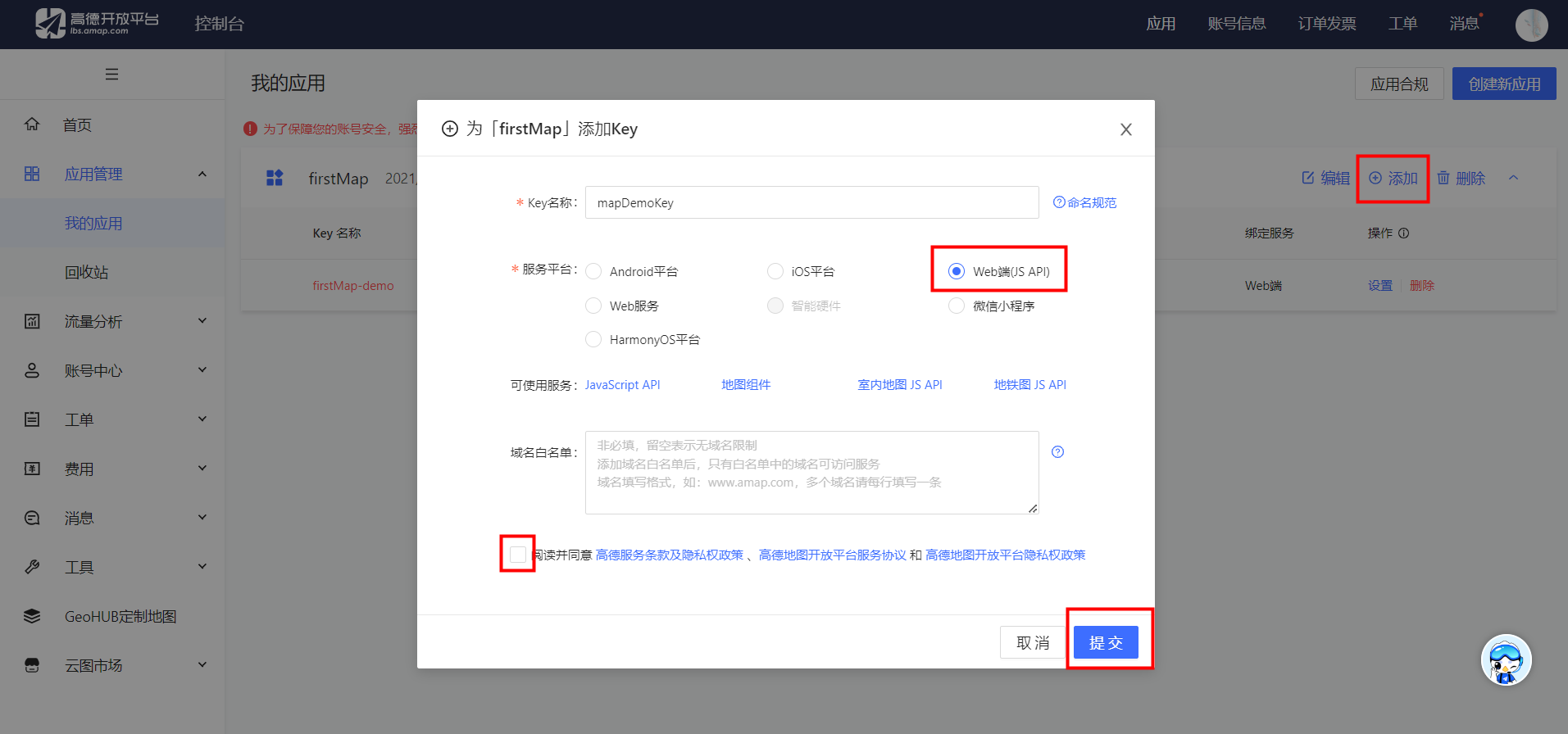
2.1.4. View created keys and security keys
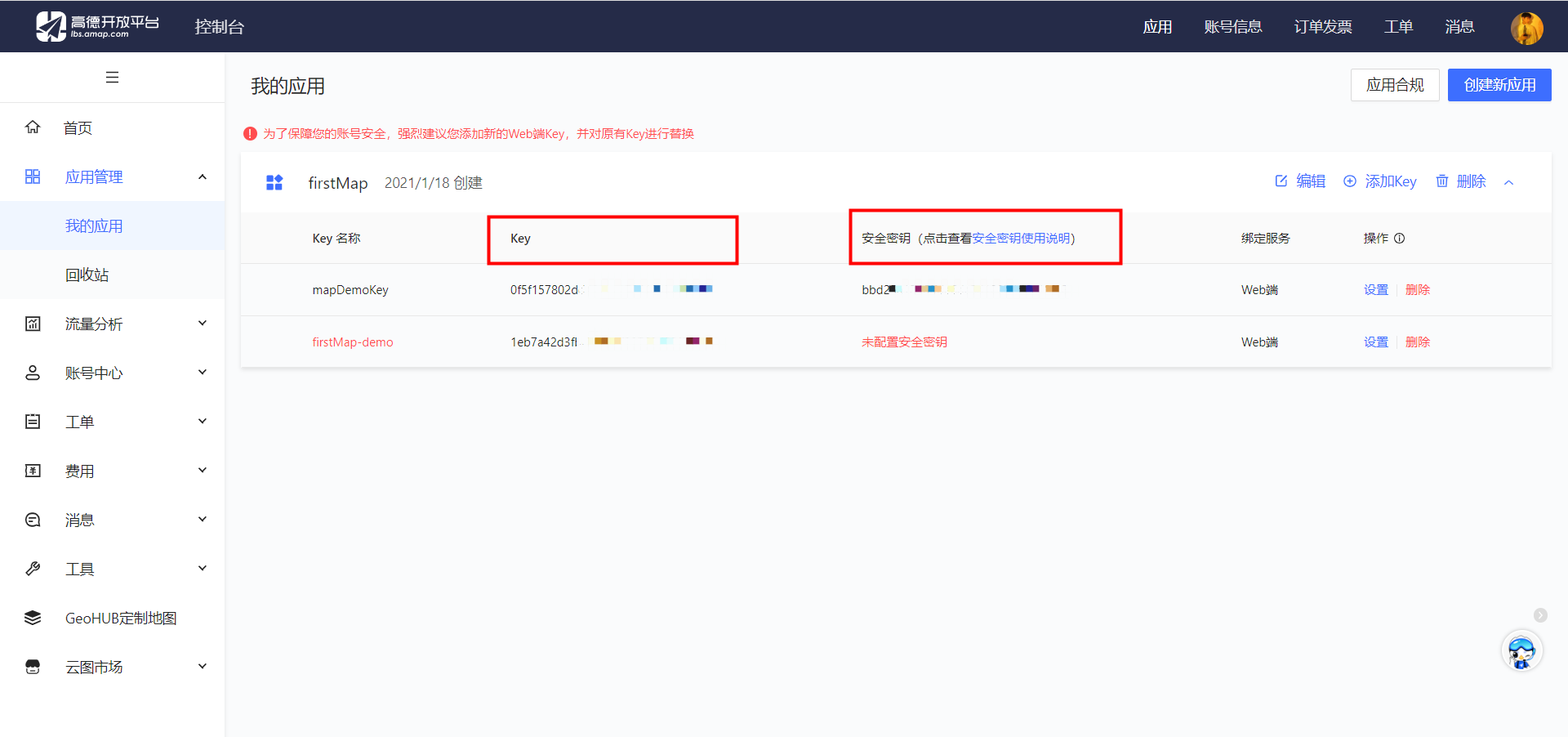
2.2. Configure custom pages

2.3. Configure JSX components

function render() {
return(
<div id='container'>
</div>
);
}
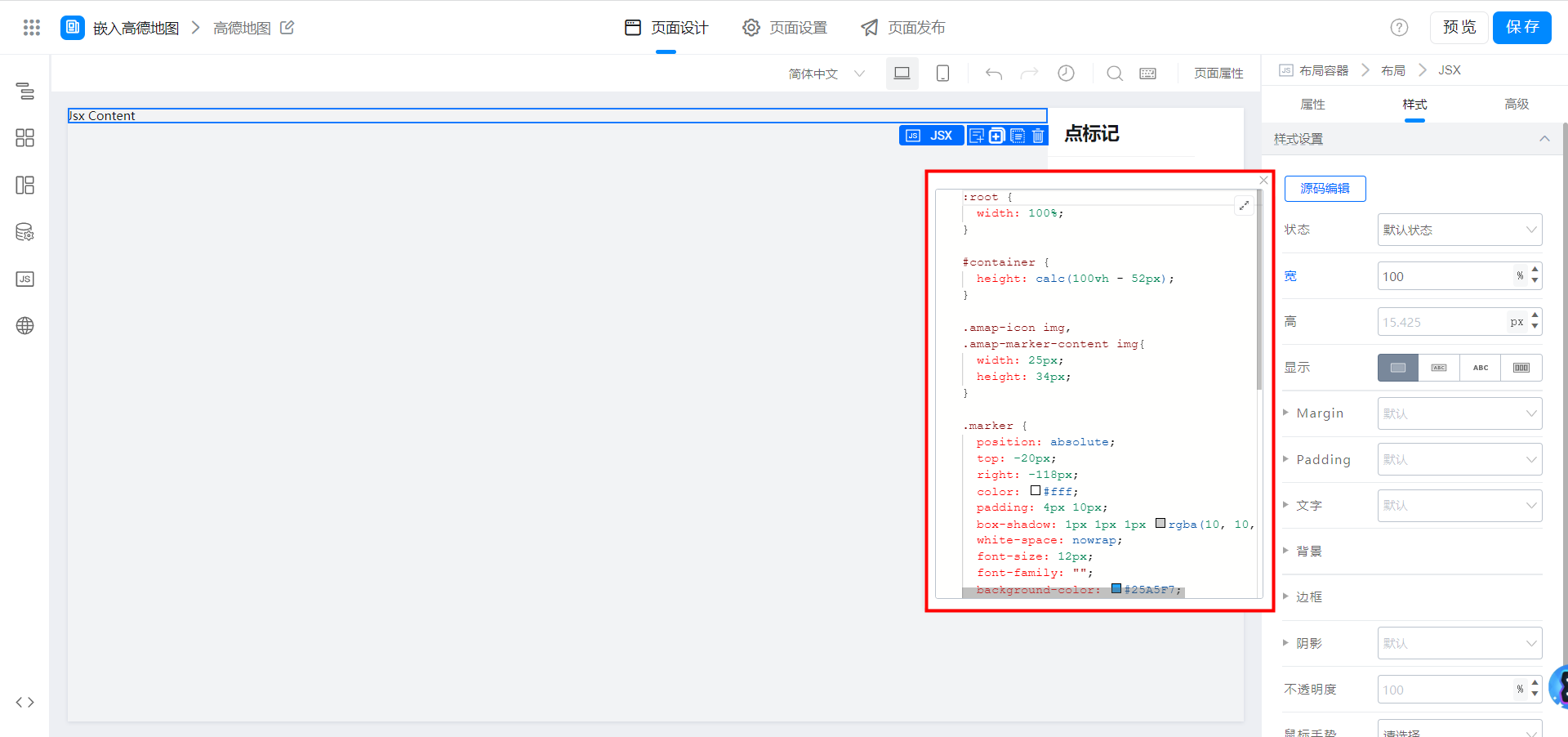
:root {
width: 100%;
}
#container {
height: calc(100vh - 52px);
}
.amap-icon img,
.amap-marker-content img{
width: 25px;
height: 34px;
}
.marker {
position: absolute;
top: -20px;
right: -118px;
color: #fff;
padding: 4px 10px;
box-shadow: 1px 1px 1px rgba(10, 10, 10, .2);
white-space: nowrap;
font-size: 12px;
font-family: "";
background-color: #25A5F7;
border-radius: 3px;
}
2.4. Configure variables
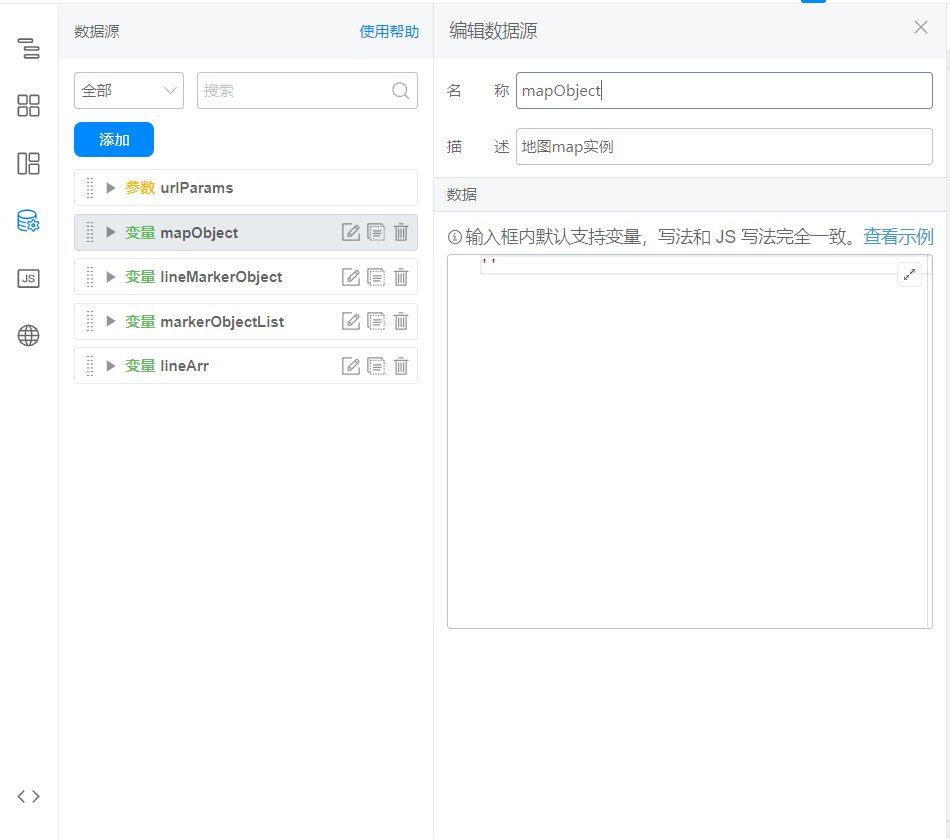
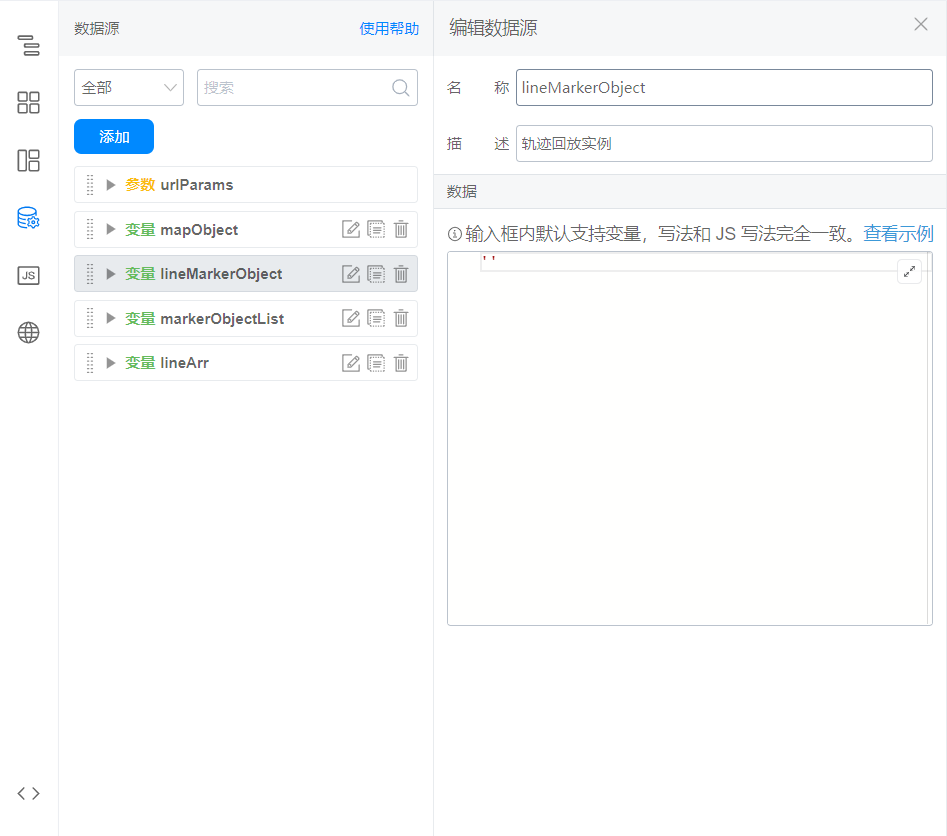
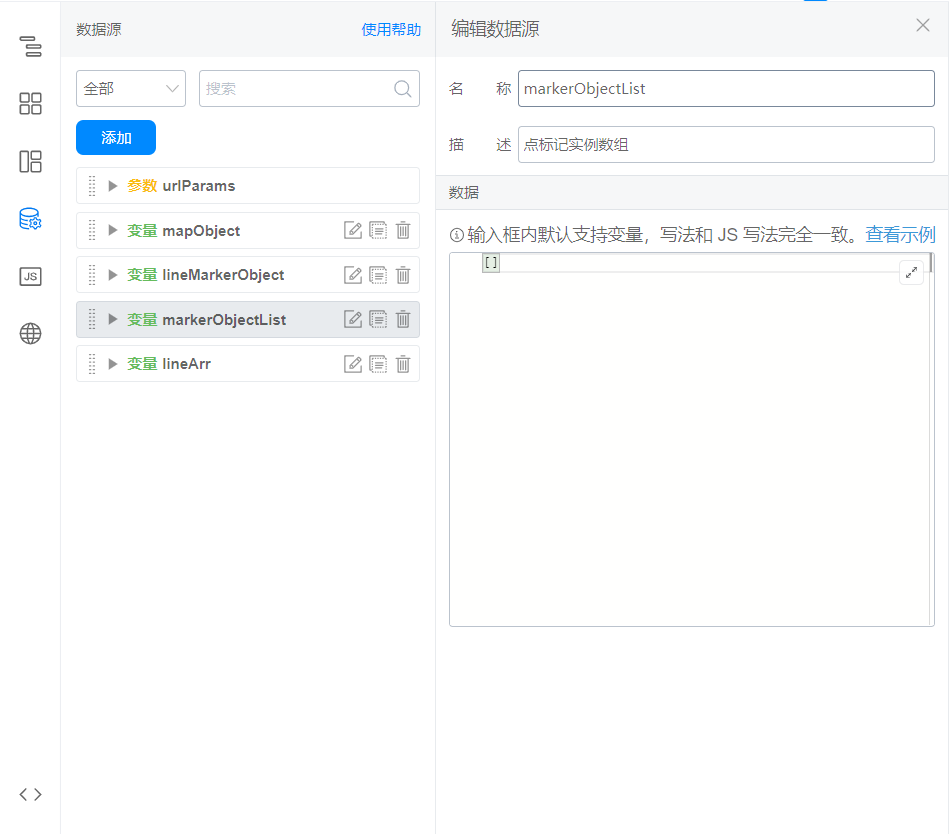
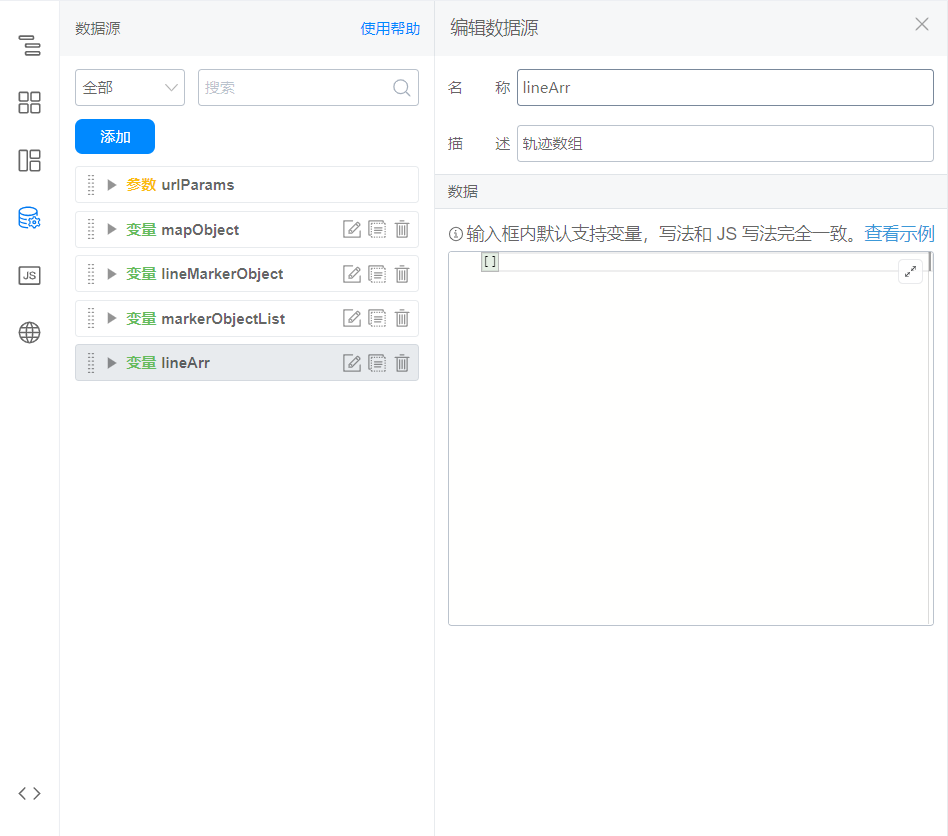
2.5. didMount introduced AMAP JSAPI

export async function didMount() {
const securityJsCode = '您申请的安全密钥'; // 「您申请的安全密钥」
const webKey = '您申请的Web开发者key'; // 「您申请的Web开发者key」
window._AMapSecurityConfig = {
securityJsCode,
};
await this.utils.loadScript(`https://webapi.amap.com/maps?v=2.0&key=${webKey}`)
.then(res => {
this.initMap();
});
}
2.6. Configure map initialization functions
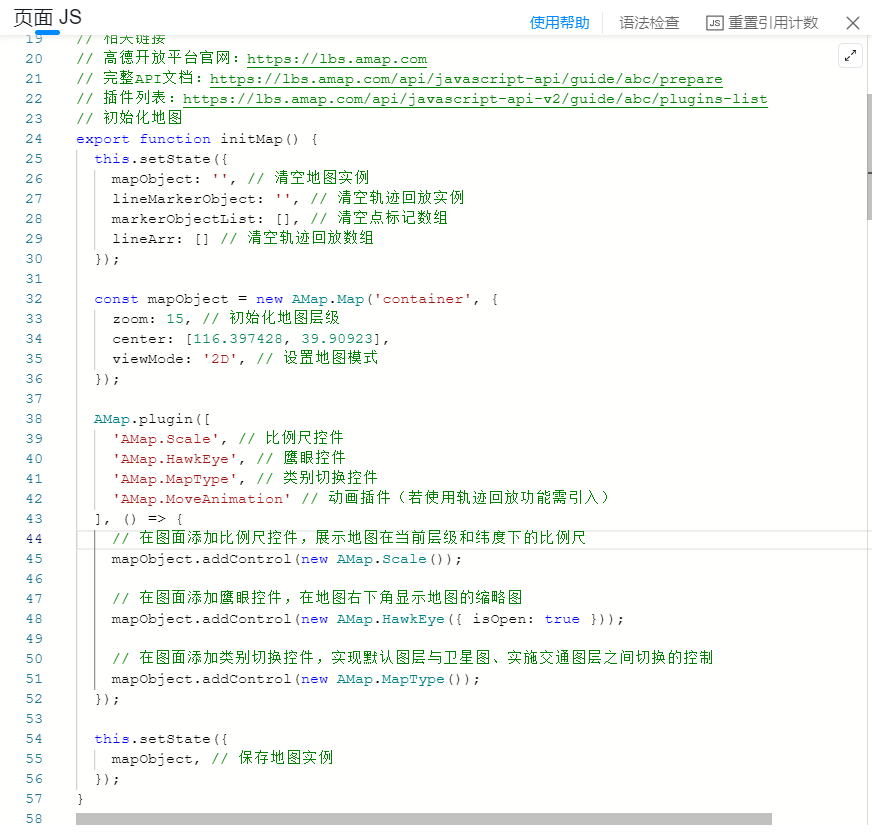
// 高德开放平台官网:https://lbs.amap.com
// 完整API文档:https://lbs.amap.com/api/javascript-api/guide/abc/prepare
// 插件列表:https://lbs.amap.com/api/javascript-api-v2/guide/abc/plugins-list
// 初始化地图
export function initMap() {
this.setState({
mapObject: '', // 清空地图实例
lineMarkerObject: '', // 清空轨迹回放实例
markerObjectList: [], // 清空点标记数组
lineArr: [] // 清空轨迹回放数组
});
const mapObject = new AMap.Map('container', {
zoom: 15, // 初始化地图层级
center: [116.397428, 39.90923],
viewMode: '2D', // 设置地图模式
});
AMap.plugin([
'AMap.Scale', // 比例尺控件
'AMap.HawkEye', // 鹰眼控件
'AMap.MapType', // 类别切换控件
'AMap.MoveAnimation' // 动画插件(若使用轨迹回放功能需引入)
], () => {
// 在图面添加比例尺控件,展示地图在当前层级和纬度下的比例尺
mapObject.addControl(new AMap.Scale());
// 在图面添加鹰眼控件,在地图右下角显示地图的缩略图
mapObject.addControl(new AMap.HawkEye({ isOpen: true }));
// 在图面添加类别切换控件,实现默认图层与卫星图、实施交通图层之间切换的控制
mapObject.addControl(new AMap.MapType());
});
this.setState({
mapObject, // 保存地图实例
});
}
2.7. Configure the add or remove point marker function
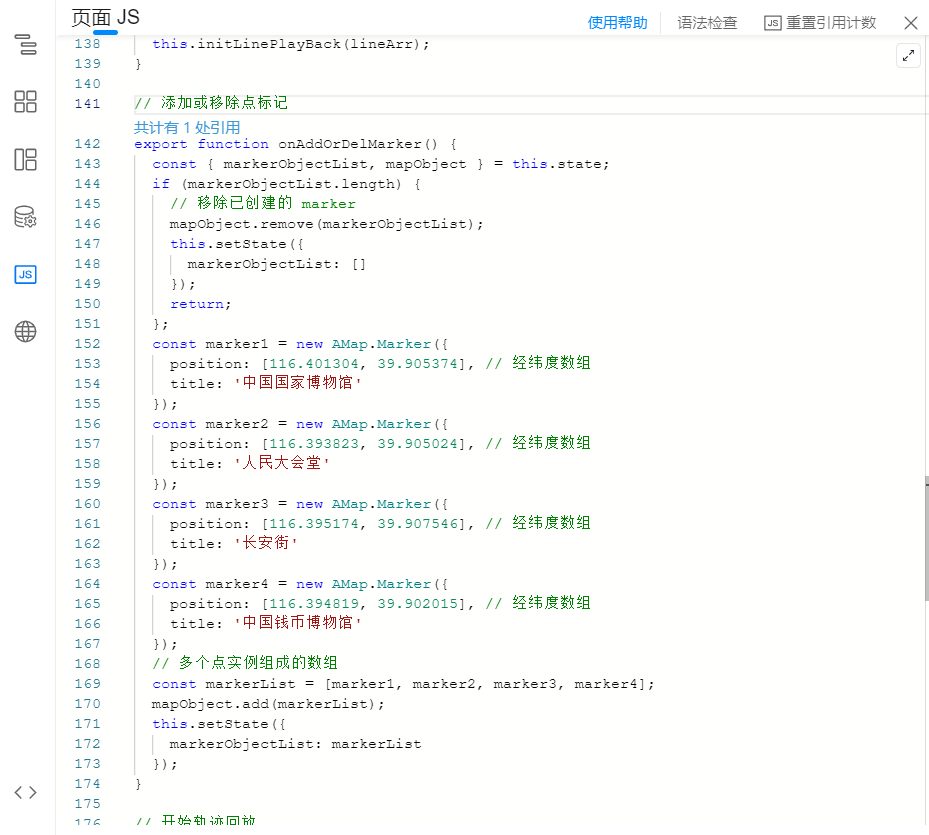
// 添加或移除点标记
export function onAddOrDelMarker() {
const { markerObjectList, mapObject } = this.state;
if (markerObjectList.length) {
// 移除已创建的 marker
mapObject.remove(markerObjectList);
this.setState({
markerObjectList: []
});
return;
};
const marker1 = new AMap.Marker({
position: [116.401304, 39.905374], // 经纬度数组
title: '中国国家博物馆'
});
const marker2 = new AMap.Marker({
position: [116.393823, 39.905024], // 经纬度数组
title: '人民大会堂'
});
const marker3 = new AMap.Marker({
position: [116.395174, 39.907546], // 经纬度数组
title: '长安街'
});
const marker4 = new AMap.Marker({
position: [116.394819, 39.902015], // 经纬度数组
title: '中国钱币博物馆'
});
// 多个点实例组成的数组
const markerList = [marker1, marker2, marker3, marker4];
mapObject.add(markerList);
this.setState({
markerObjectList: markerList
});
}
2.8. Configure trajectory playback initialization function
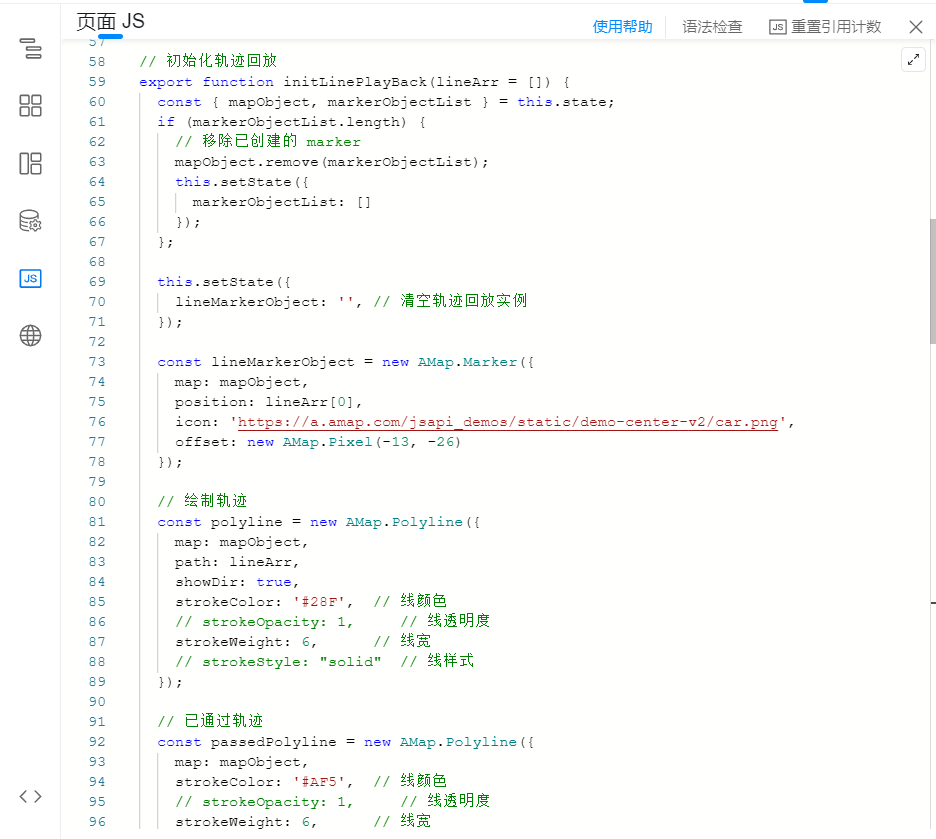
// 初始化轨迹回放
export function initLinePlayBack(lineArr = []) {
const { mapObject, markerObjectList } = this.state;
if (markerObjectList.length) {
// 移除已创建的 marker
mapObject.remove(markerObjectList);
this.setState({
markerObjectList: []
});
};
this.setState({
lineMarkerObject: '', // 清空轨迹回放实例
});
const lineMarkerObject = new AMap.Marker({
map: mapObject,
position: lineArr[0],
icon: 'https://a.amap.com/jsapi_demos/static/demo-center-v2/car.png',
offset: new AMap.Pixel(-13, -26)
});
// 绘制轨迹
const polyline = new AMap.Polyline({
map: mapObject,
path: lineArr,
showDir: true,
strokeColor: '#28F', // 线颜色
// strokeOpacity: 1, // 线透明度
strokeWeight: 6, // 线宽
// strokeStyle: "solid" // 线样式
});
// 已通过轨迹
const passedPolyline = new AMap.Polyline({
map: mapObject,
strokeColor: '#AF5', // 线颜色
// strokeOpacity: 1, // 线透明度
strokeWeight: 6, // 线宽
// strokeStyle: "solid" // 线样式
});
lineMarkerObject.on('moving', (e) => {
passedPolyline.setPath(e.passedPath);
mapObject.setCenter(e.target.getPosition(), true);
});
mapObject.setFitView();
this.setState({
lineMarkerObject, // 保存轨迹回放实例
});
}
2.9. Configure the create trajectory playback instance function
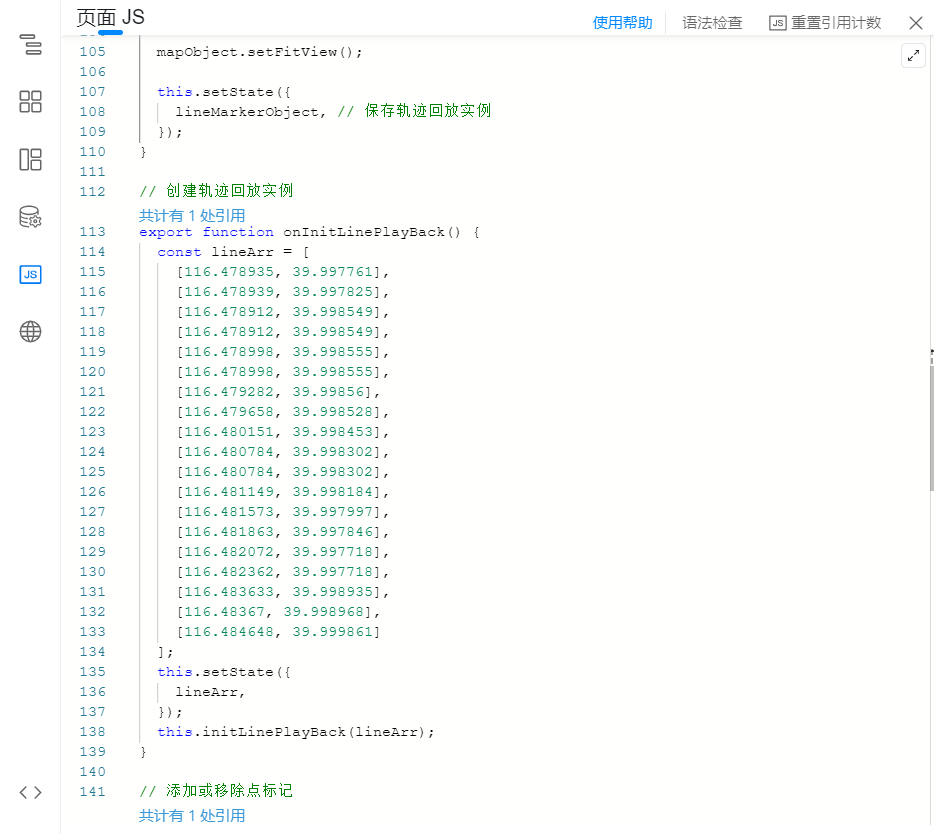
// 创建轨迹回放实例
export function onInitLinePlayBack() {
const lineArr = [
[116.478935, 39.997761],
[116.478939, 39.997825],
[116.478912, 39.998549],
[116.478912, 39.998549],
[116.478998, 39.998555],
[116.478998, 39.998555],
[116.479282, 39.99856],
[116.479658, 39.998528],
[116.480151, 39.998453],
[116.480784, 39.998302],
[116.480784, 39.998302],
[116.481149, 39.998184],
[116.481573, 39.997997],
[116.481863, 39.997846],
[116.482072, 39.997718],
[116.482362, 39.997718],
[116.483633, 39.998935],
[116.48367, 39.998968],
[116.484648, 39.999861]
];
this.setState({
lineArr,
});
this.initLinePlayBack(lineArr);
}
2.10. Configure trajectory playback control functions
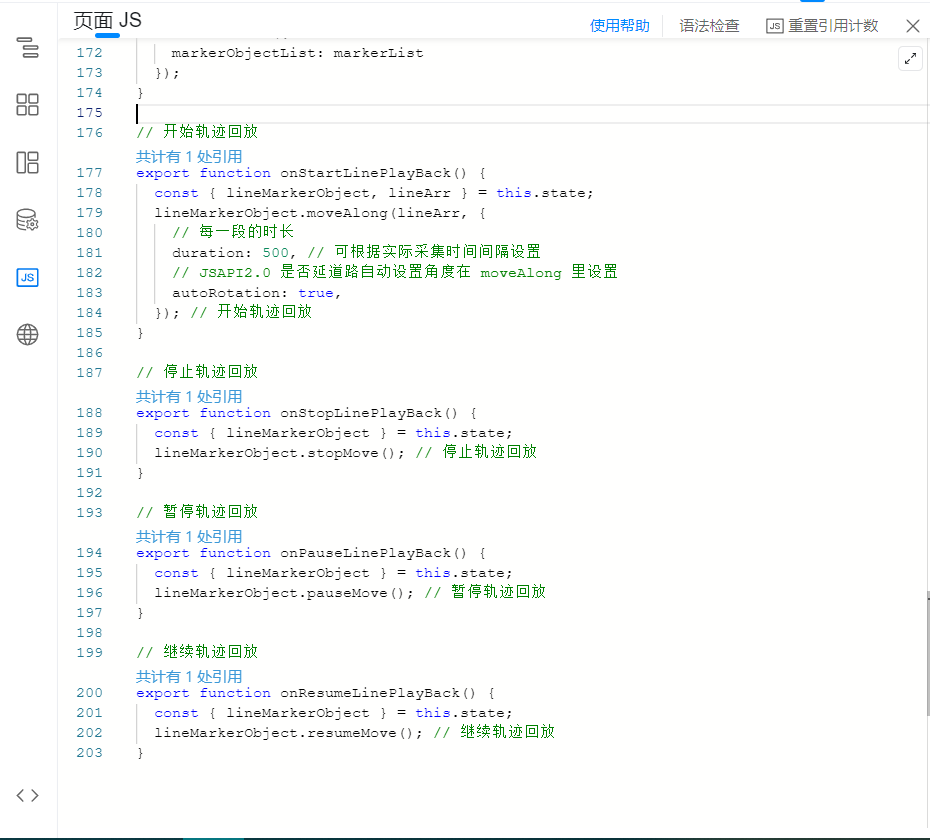
// 开始轨迹回放
export function onStartLinePlayBack() {
const { lineMarkerObject, lineArr } = this.state;
lineMarkerObject.moveAlong(lineArr, {
// 每一段的时长
duration: 500, // 可根据实际采集时间间隔设置
// JSAPI2.0 是否延道路自动设置角度在 moveAlong 里设置
autoRotation: true,
}); // 开始轨迹回放
}
// 停止轨迹回放
export function onStopLinePlayBack() {
const { lineMarkerObject } = this.state;
lineMarkerObject.stopMove(); // 停止轨迹回放
}
// 暂停轨迹回放
export function onPauseLinePlayBack() {
const { lineMarkerObject } = this.state;
lineMarkerObject.pauseMove(); // 暂停轨迹回放
}
// 继续轨迹回放
export function onResumeLinePlayBack() {
const { lineMarkerObject } = this.state;
lineMarkerObject.resumeMove(); // 继续轨迹回放
}
2.11. Event binding
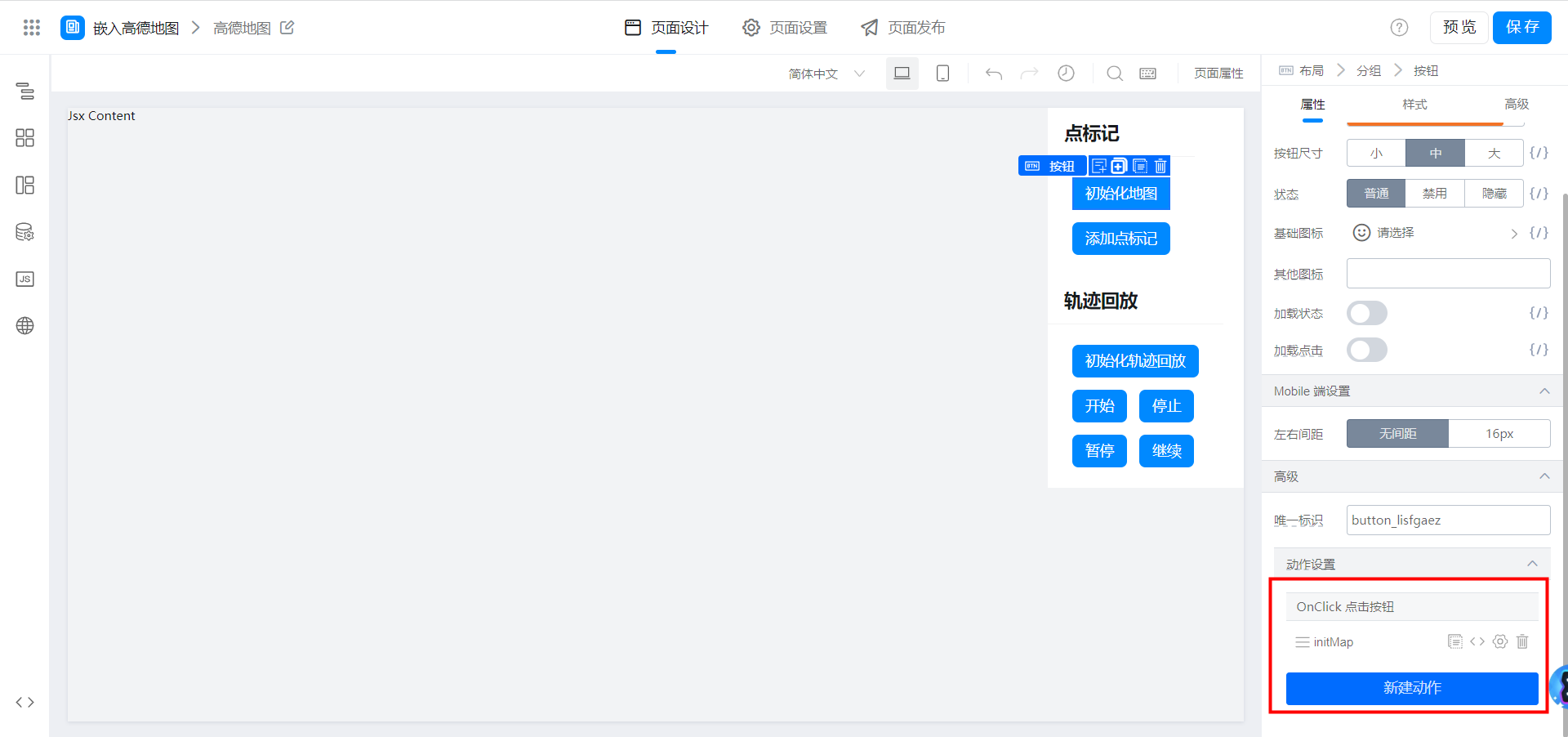
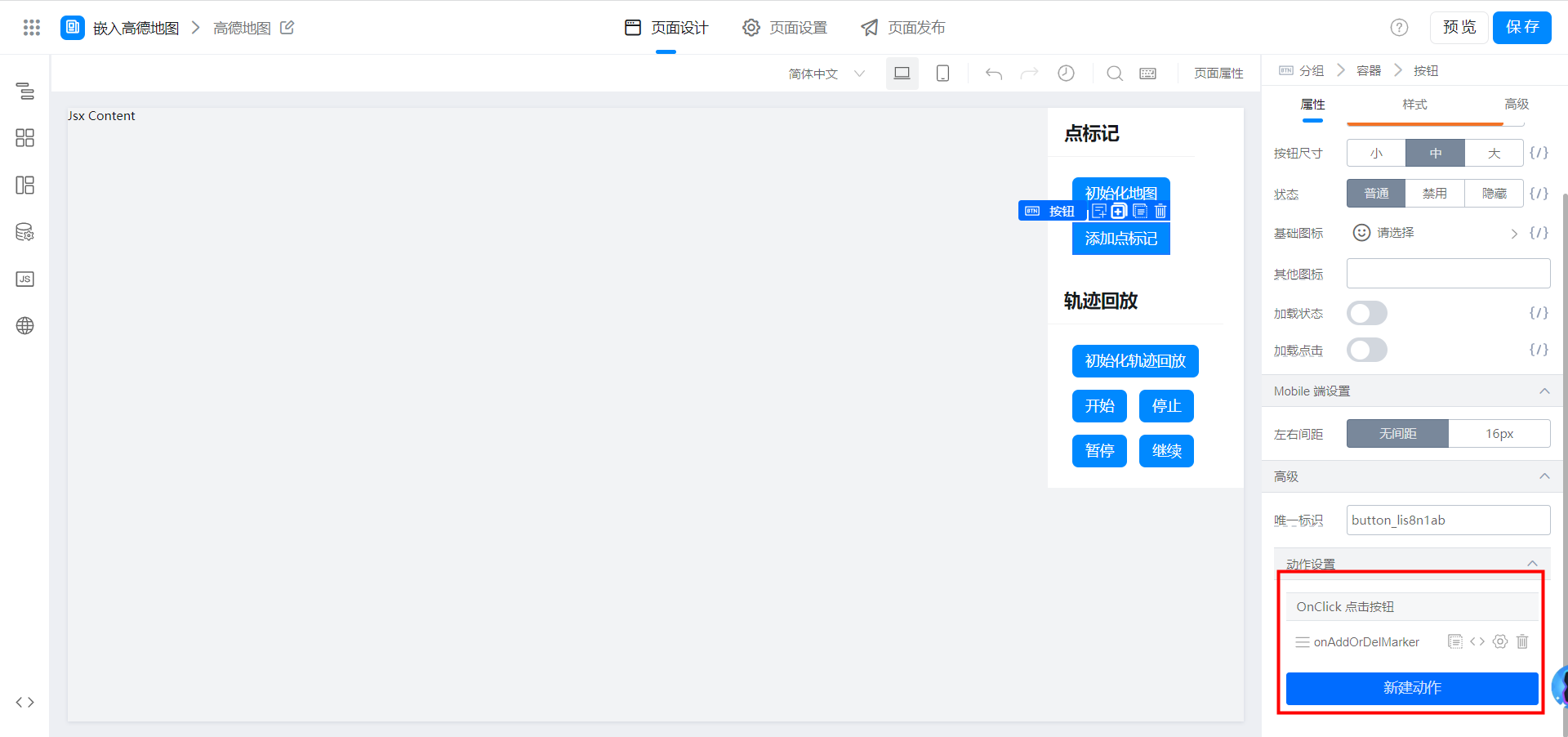

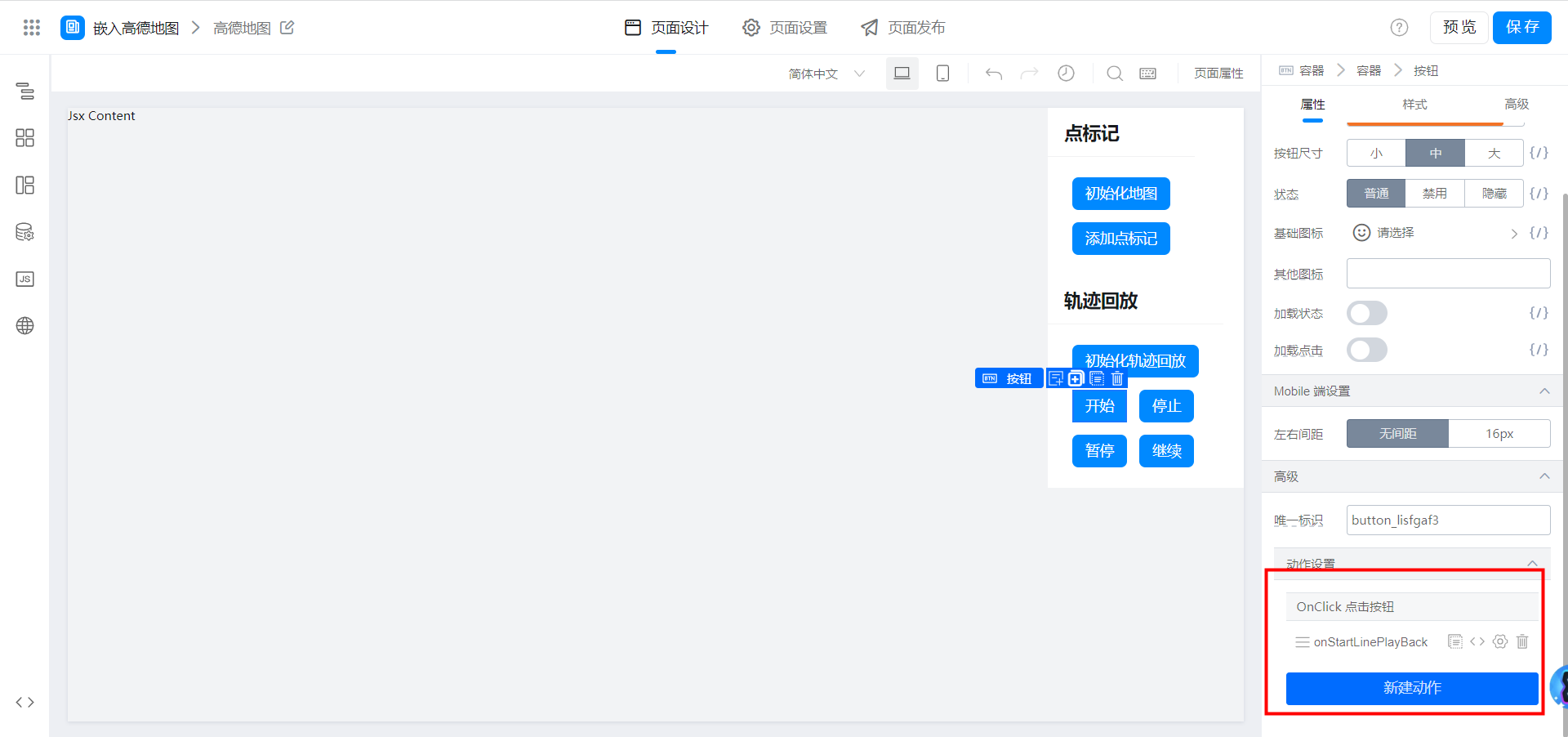
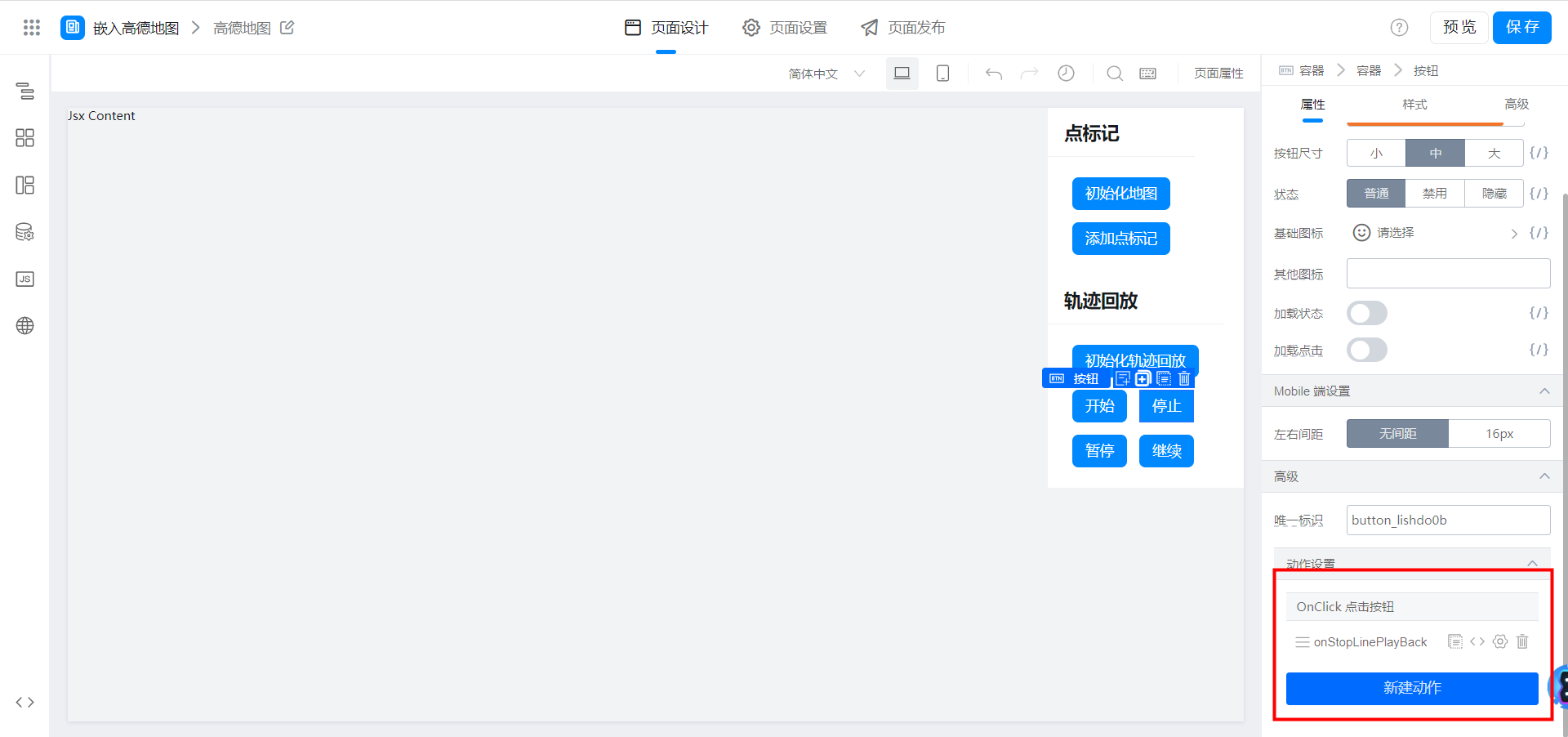
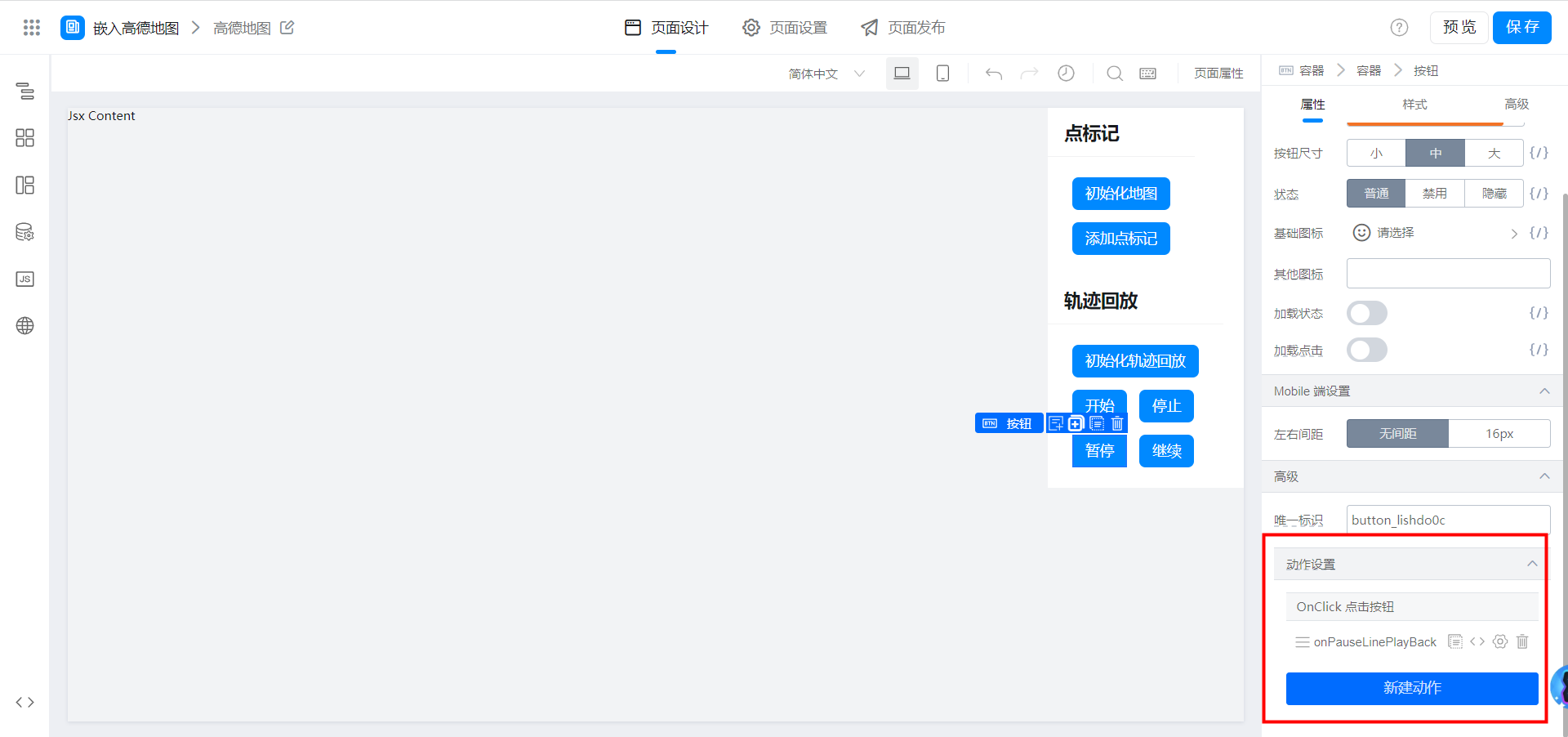
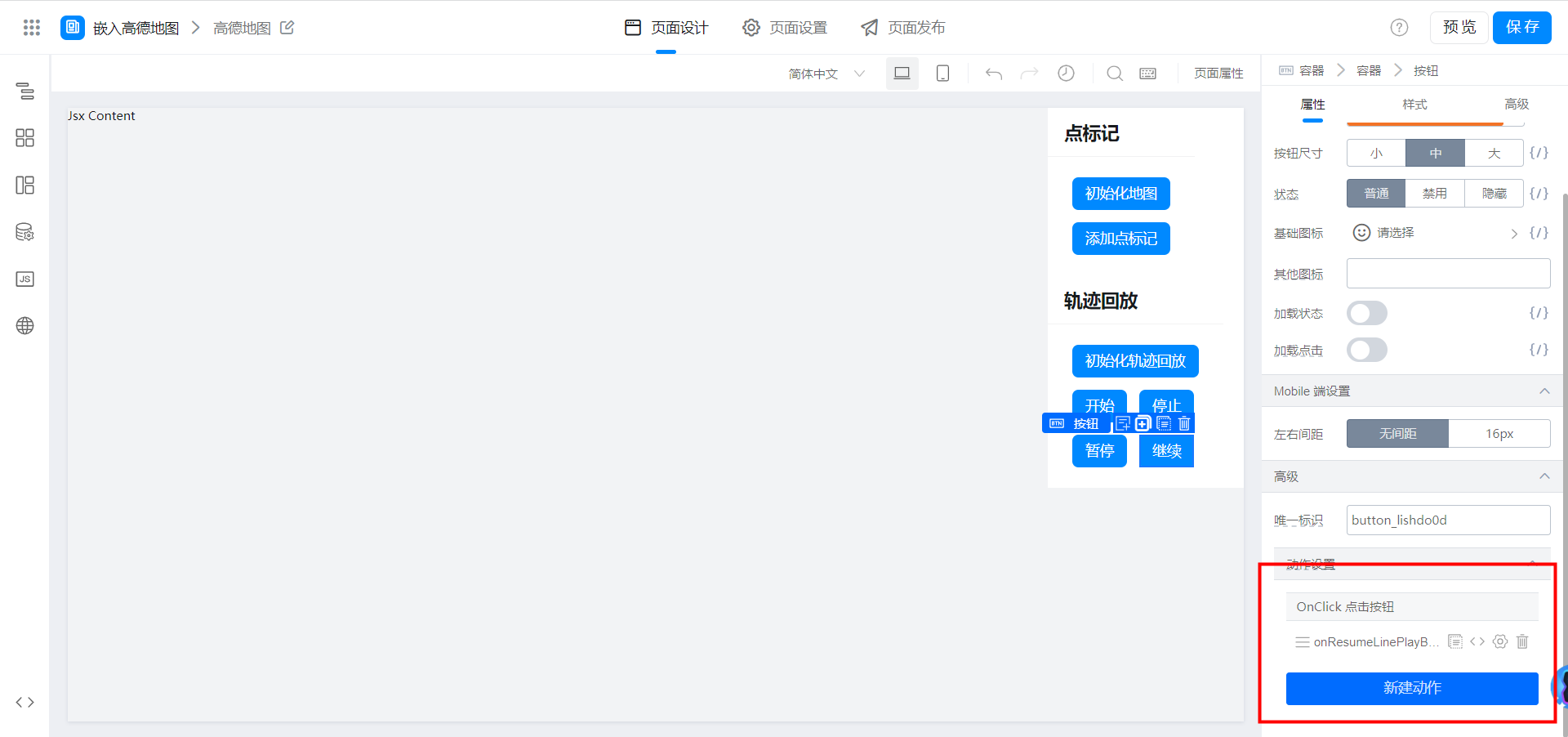
3. Effect
3.1. Point mark
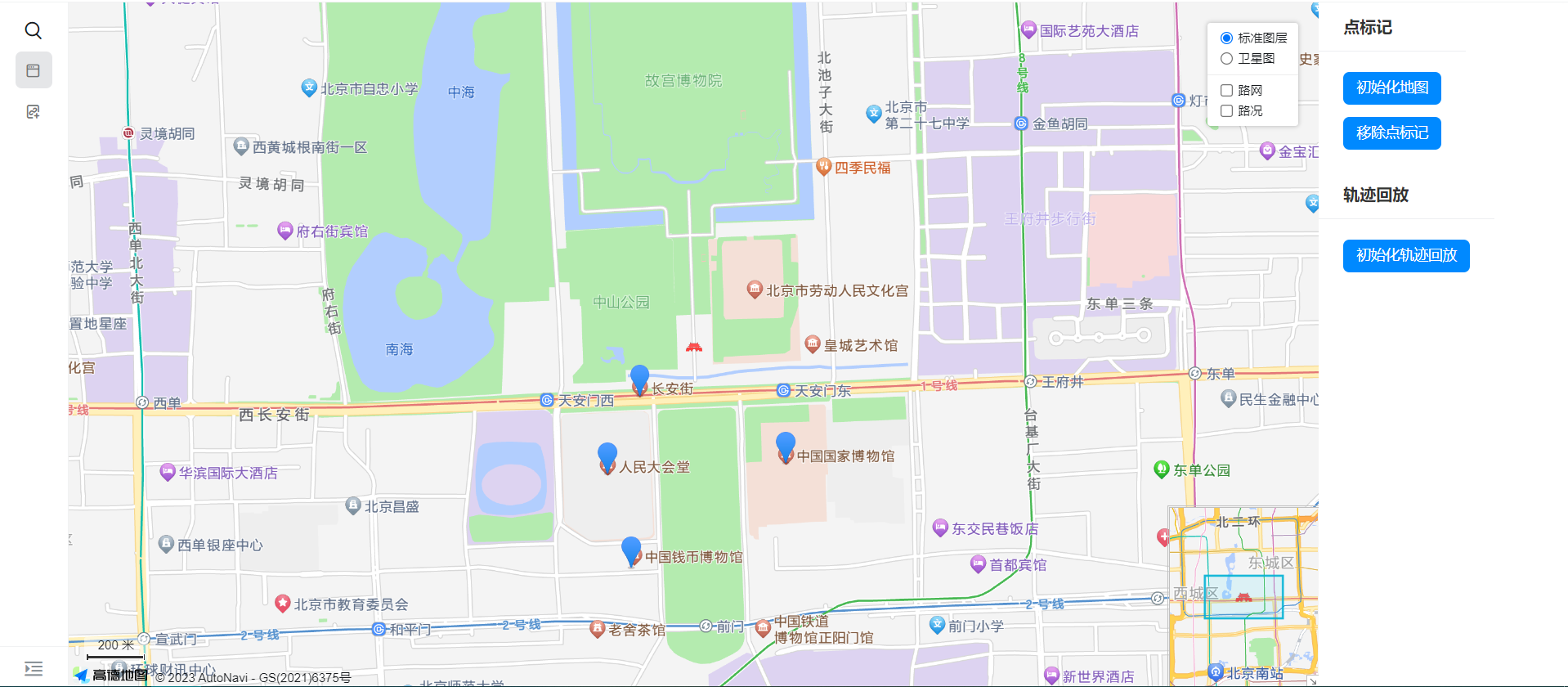
3.2. Track Playback

4. Try it online
This doc is generated using machine translation. Any discrepancies or differences created in the translation are not binding and have no legal effect for compliance or enforcement purposes.
本文档对您是否有帮助?