Custom page drop-down load more
1. Usage scenarios
When a custom page displays data in a loop, we can normally view more data by using pagination. In this example, we discuss how to view more data without switching through listening elements.
2. Implement functions
2.1. Create a custom page
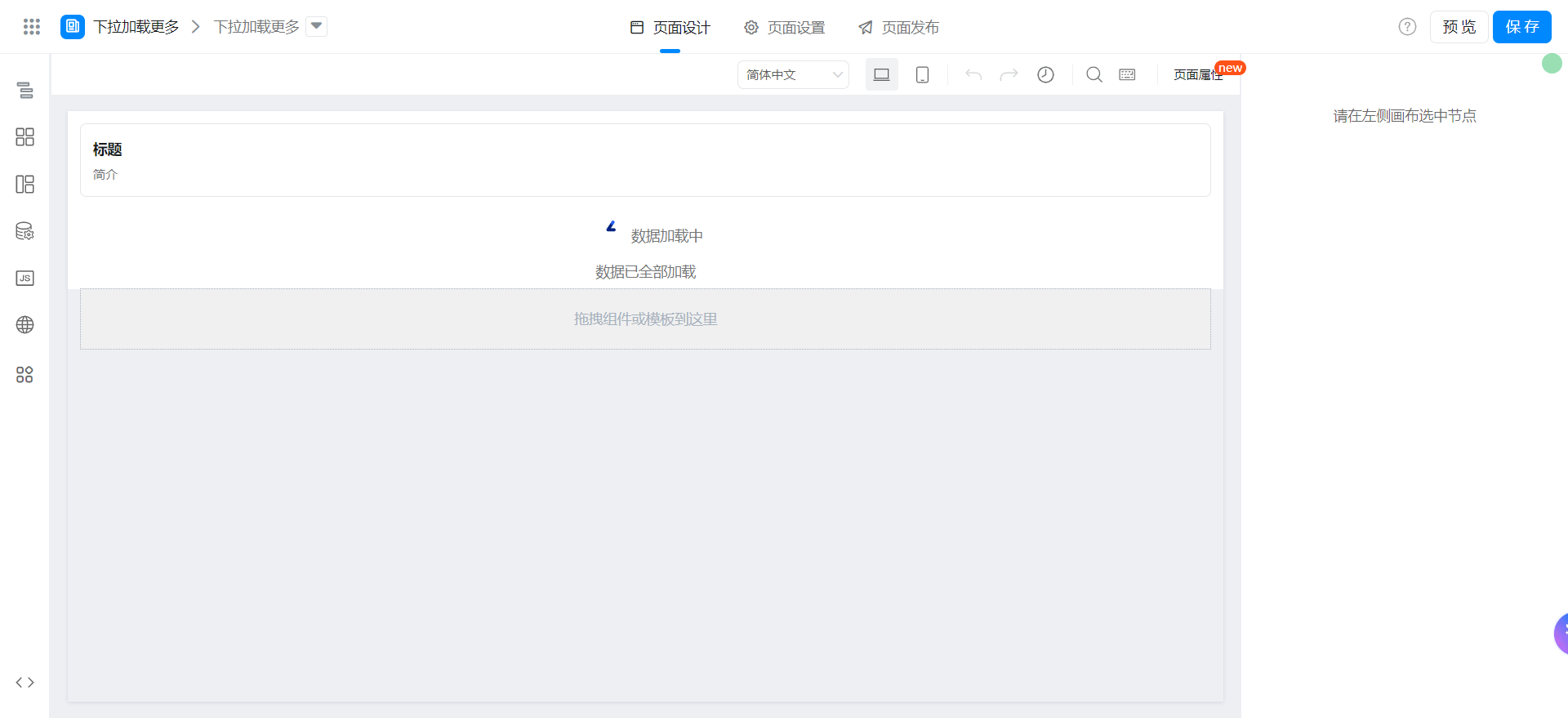
The outline tree is as follows:
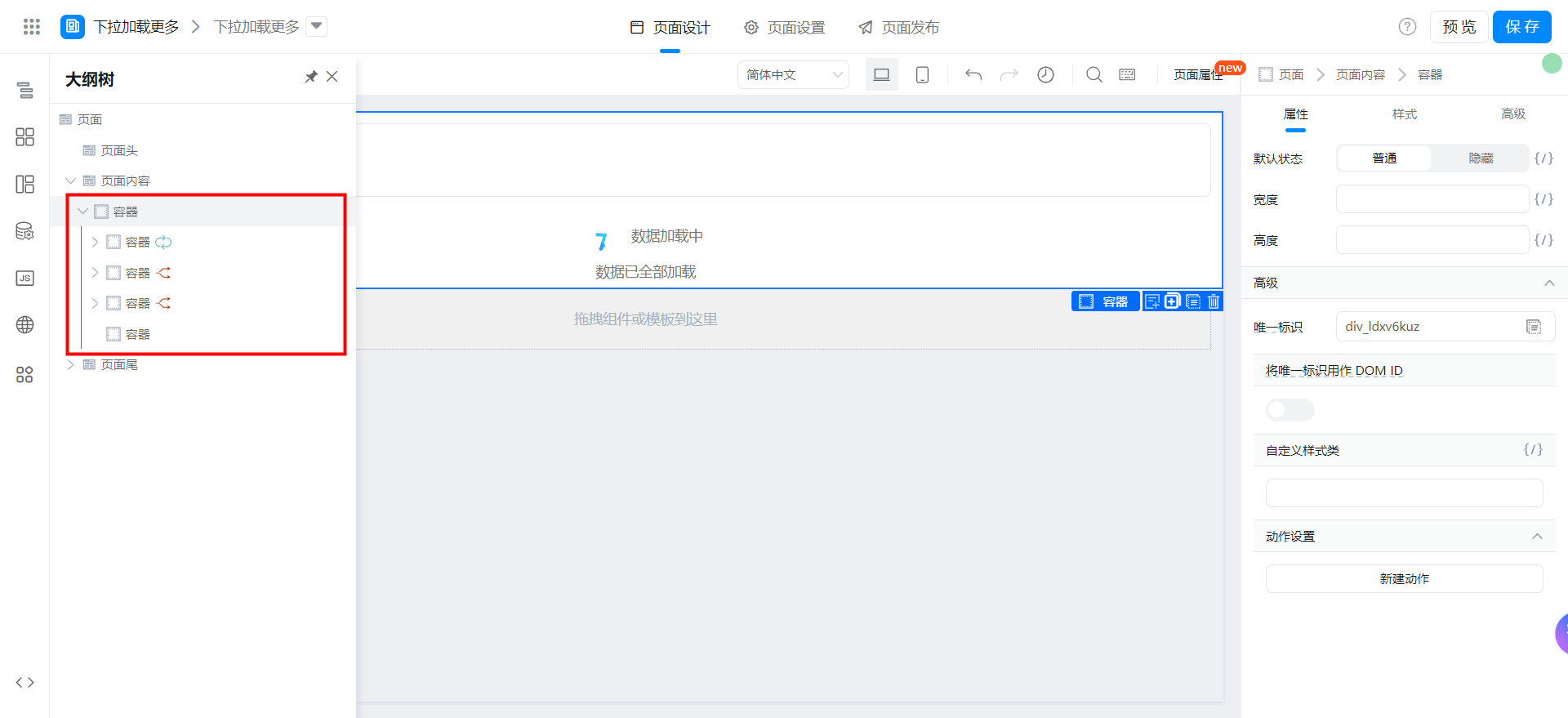
Loop container (used to loop the results of data requests):
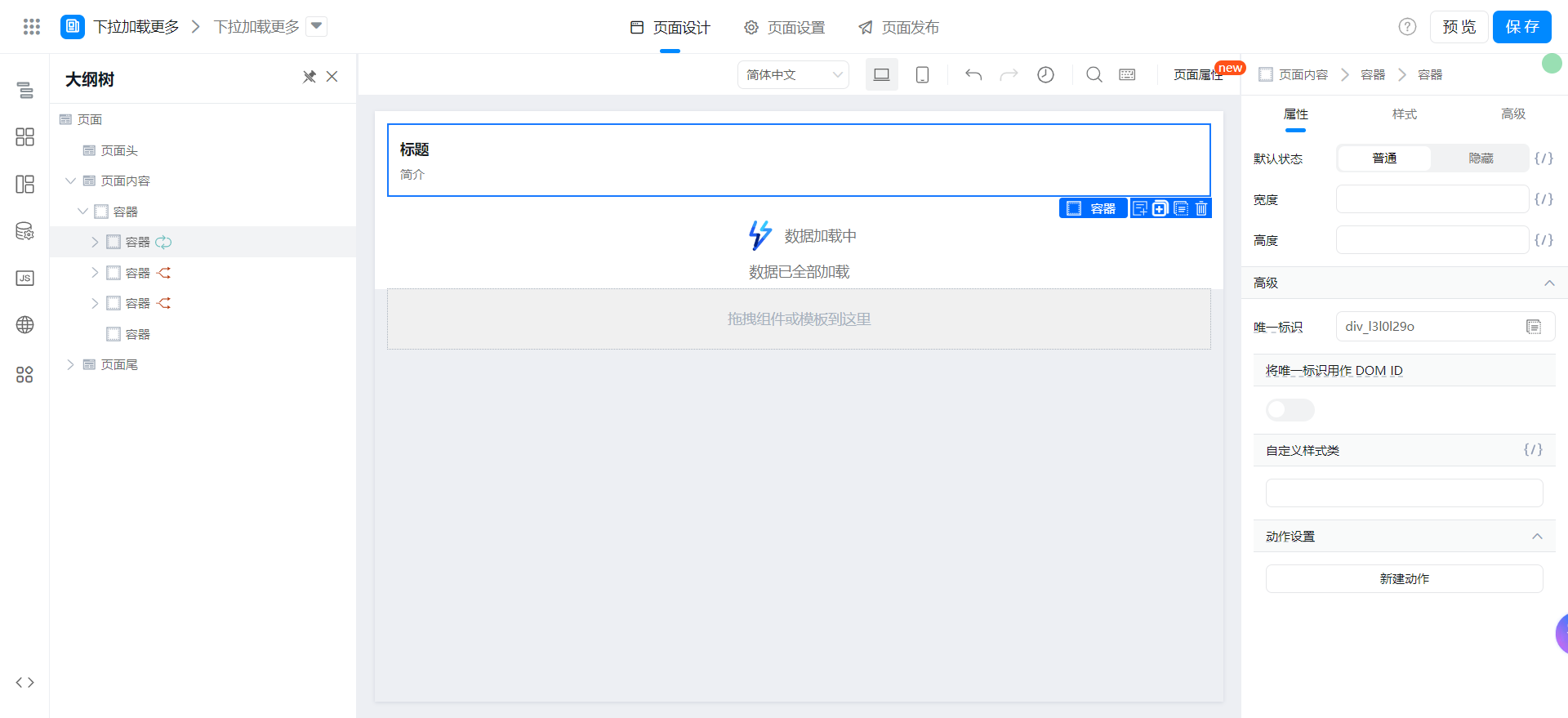
loading container (used to prompt the data loading process):
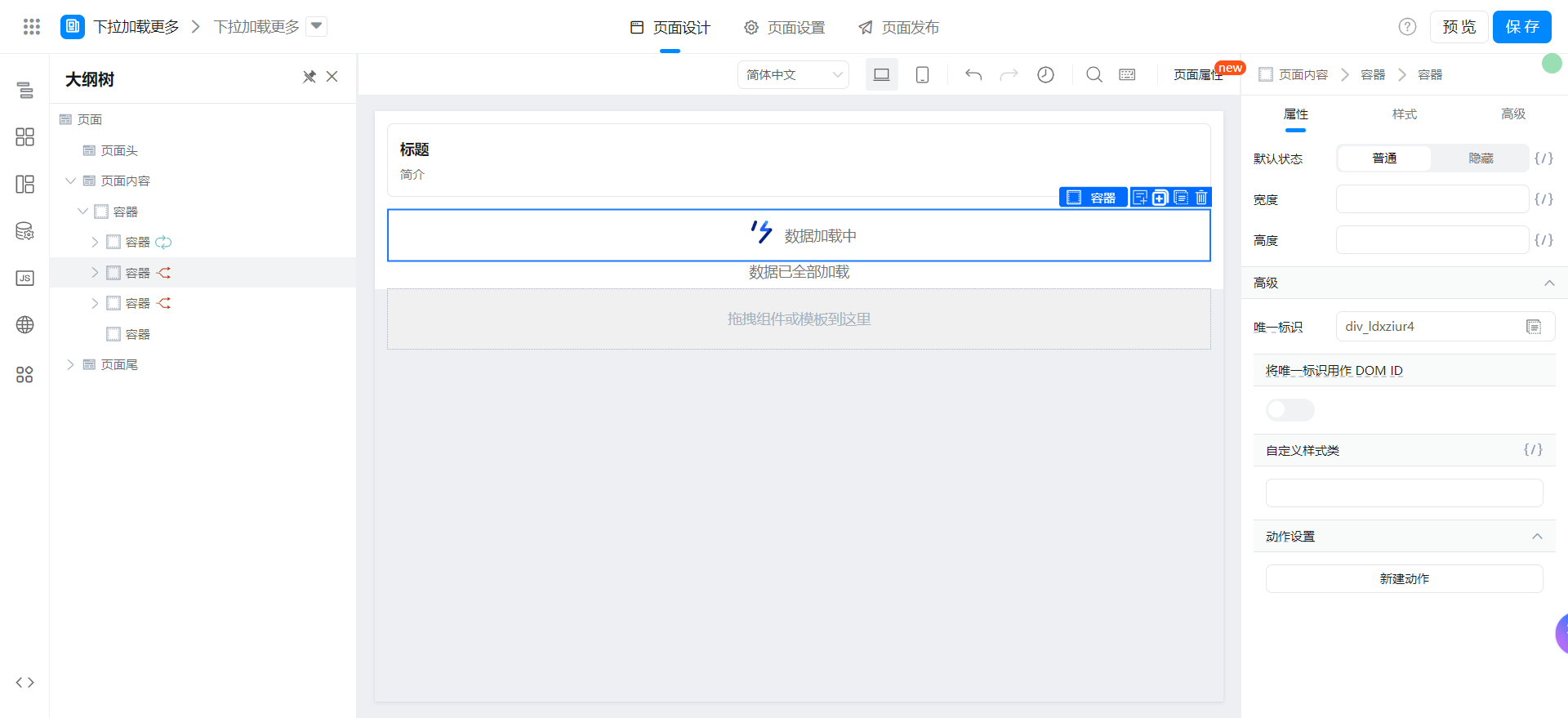
Container after data loading (used to indicate that all data has been loaded):

Drop-down load listener container (enable the unique identifier as DOM ID and set the height to 1px):
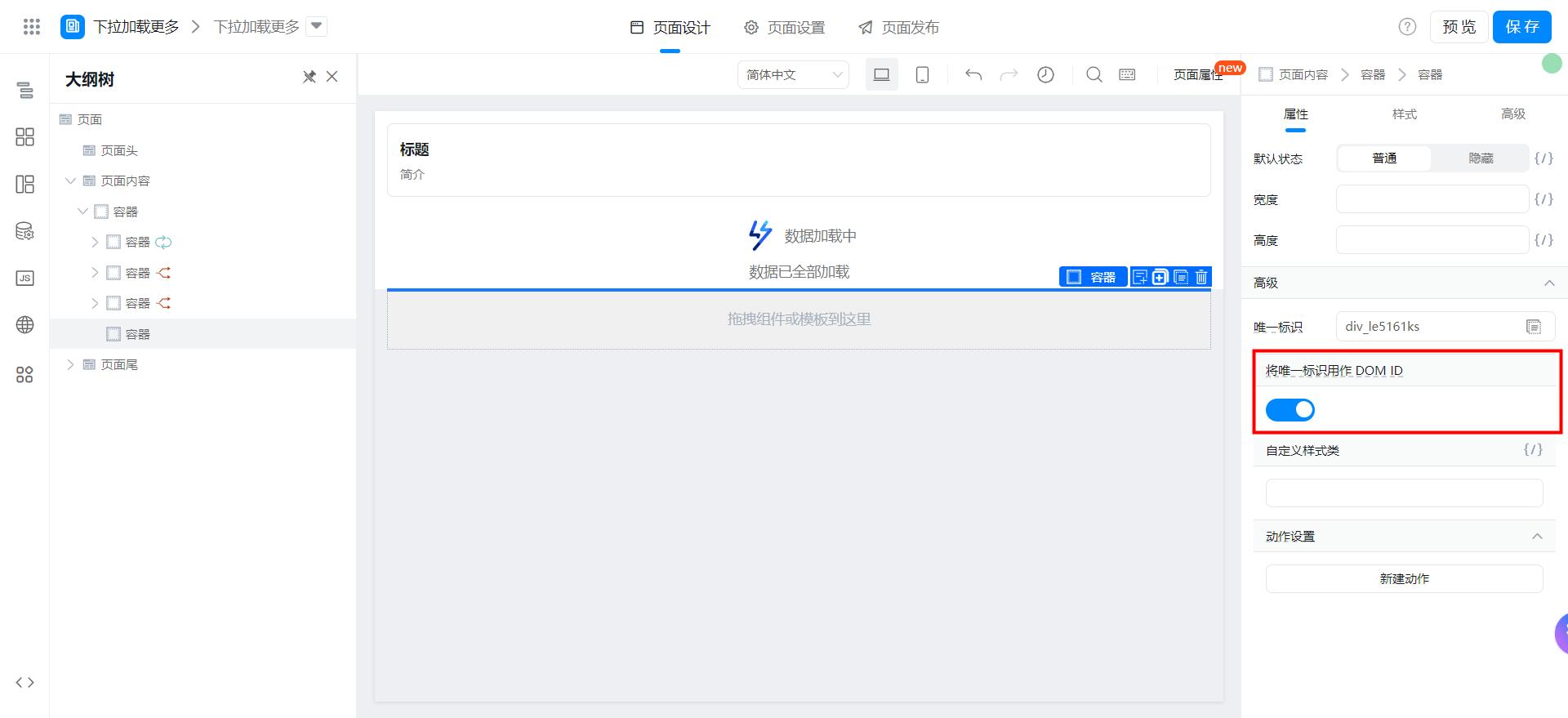
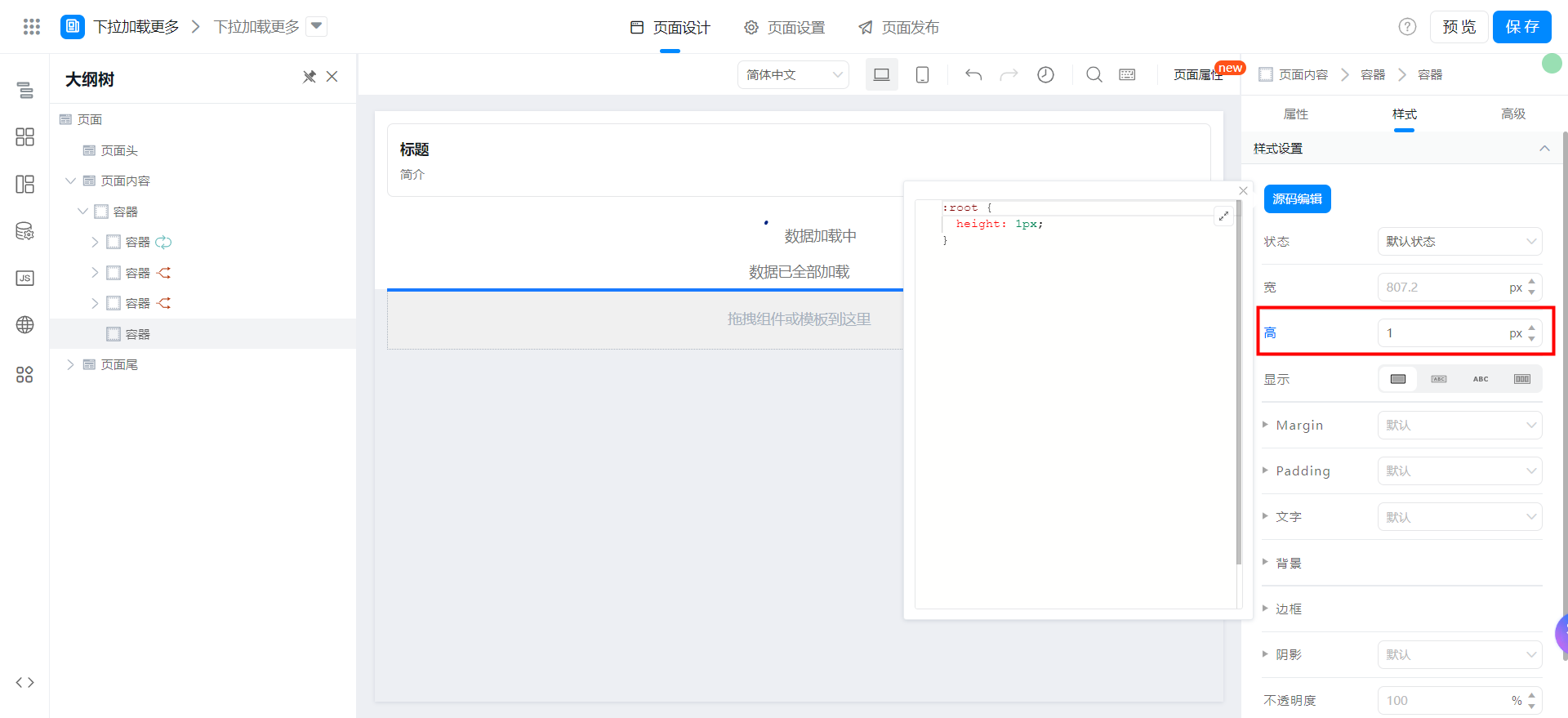
2.2. Configure a data source
Reference documents:Search form instance details by criteria
The interface configuration is as follows:
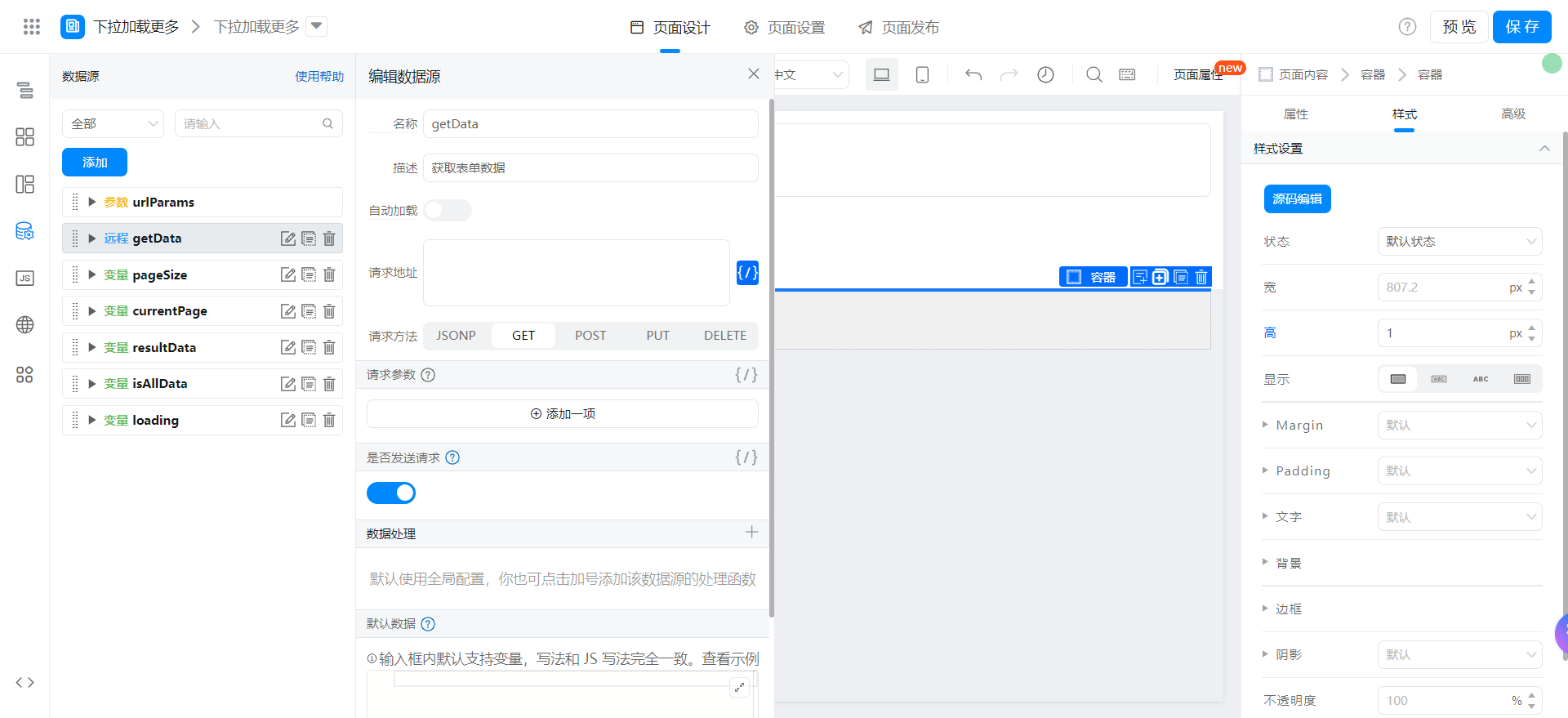
`/${window.pageConfig.appType || window.g_config.appKey}/v1/form/searchFormDatas.json`
2.3. Configure page variables
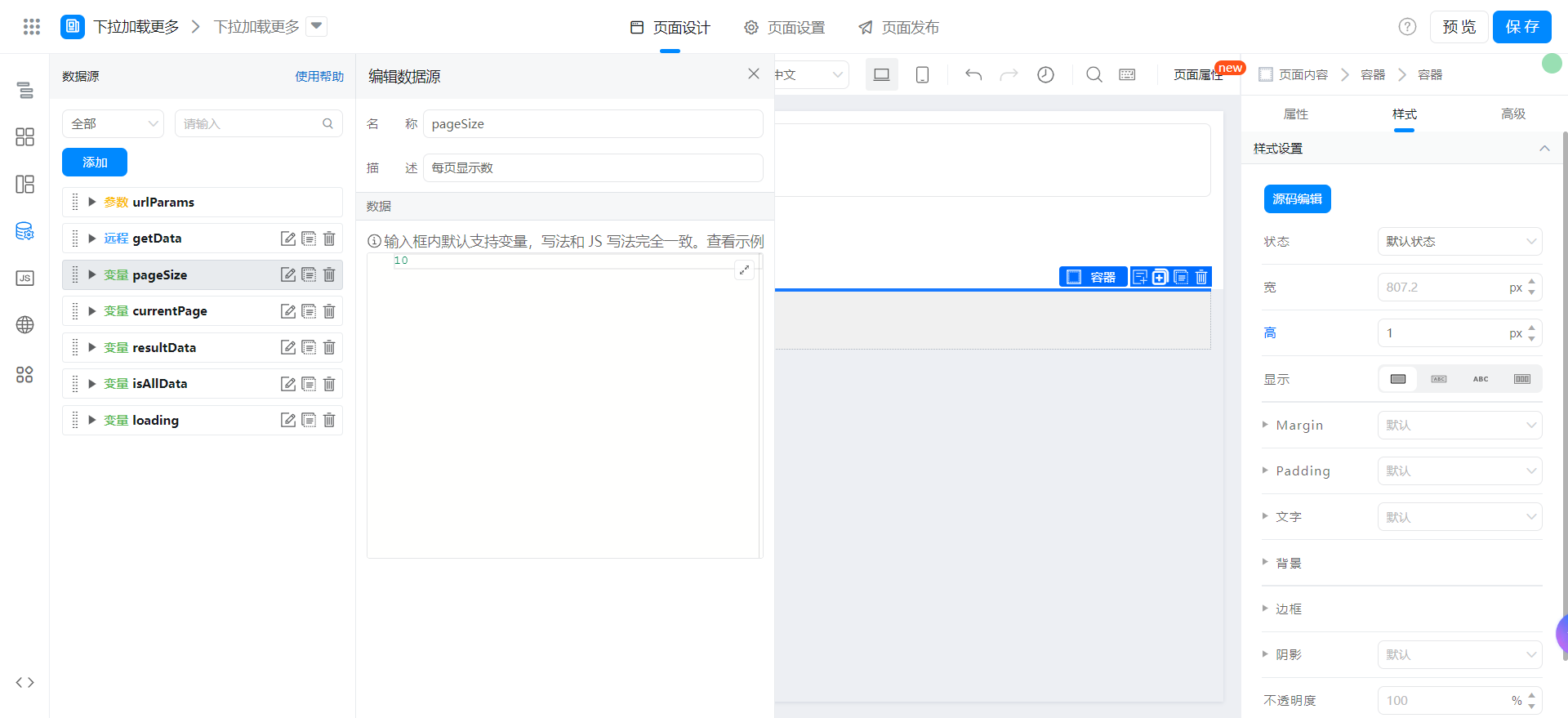
- pageSize: number of displays per page. Default value: 10
- currentPage: the current page number. Default value: 1
- resultData: request result, default value []
- isAllData: whether all data is loaded. Default value: false
- loading: data loading status. Default value: false
2.4. Configuration page display effect
The loop container binds the loop data:
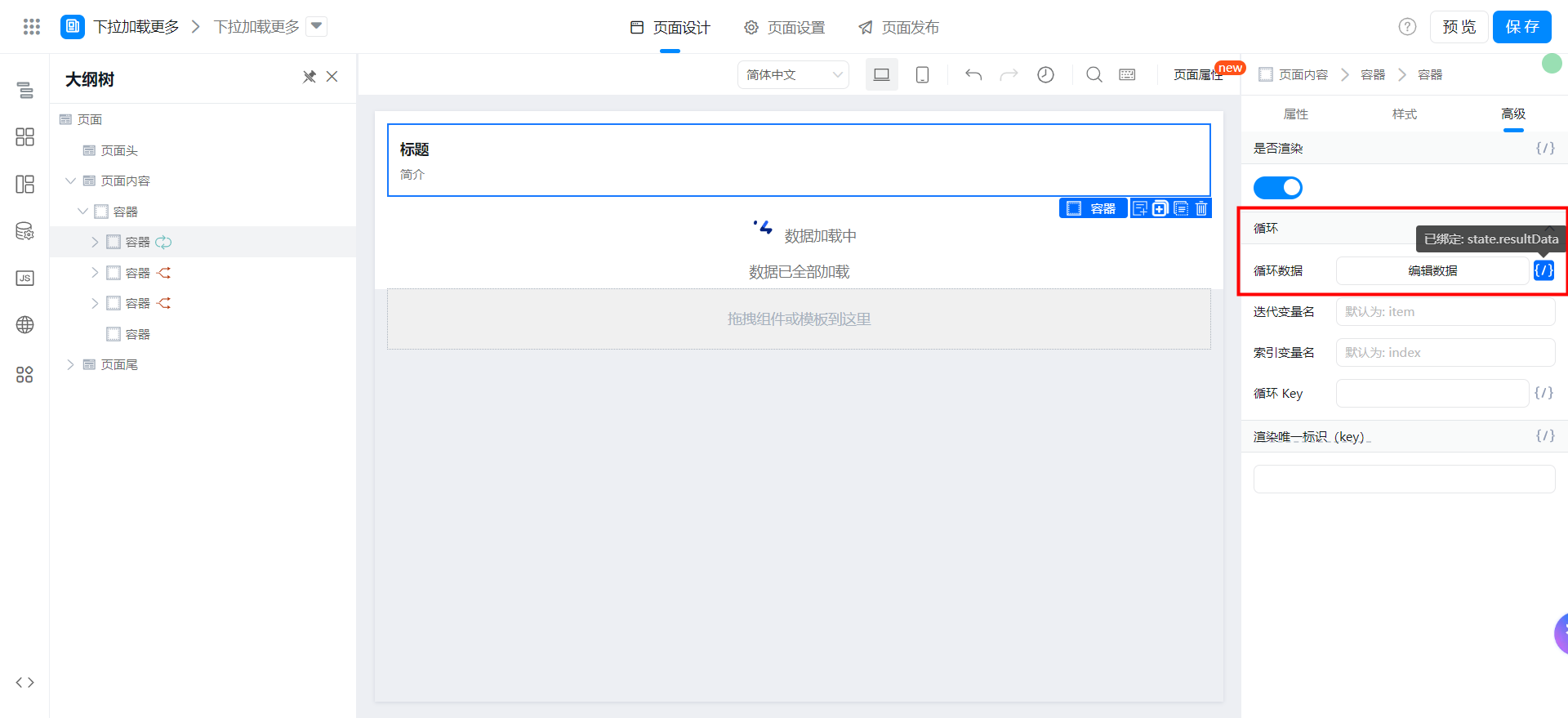
Title binding variable:
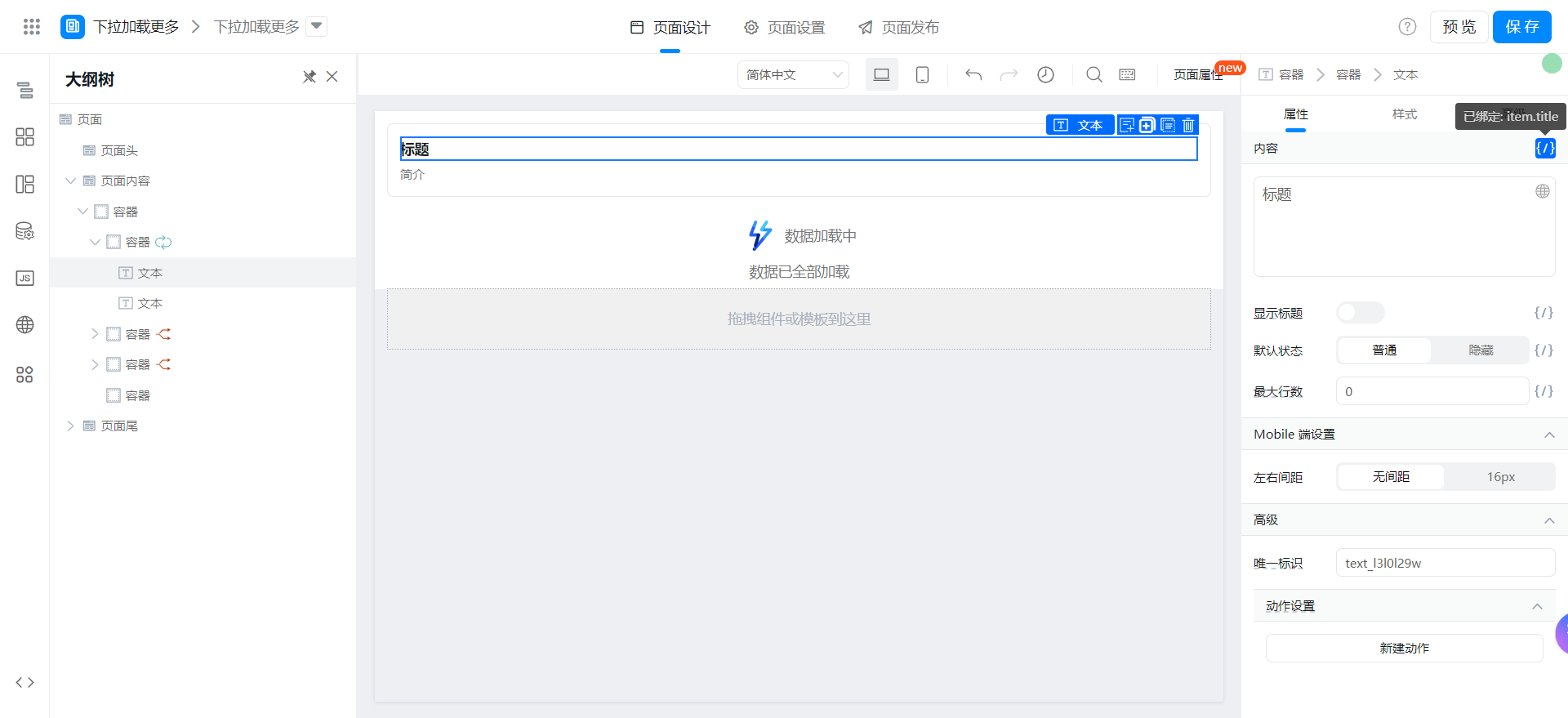
Introduction to binding variables:

Data loading container binding variables:
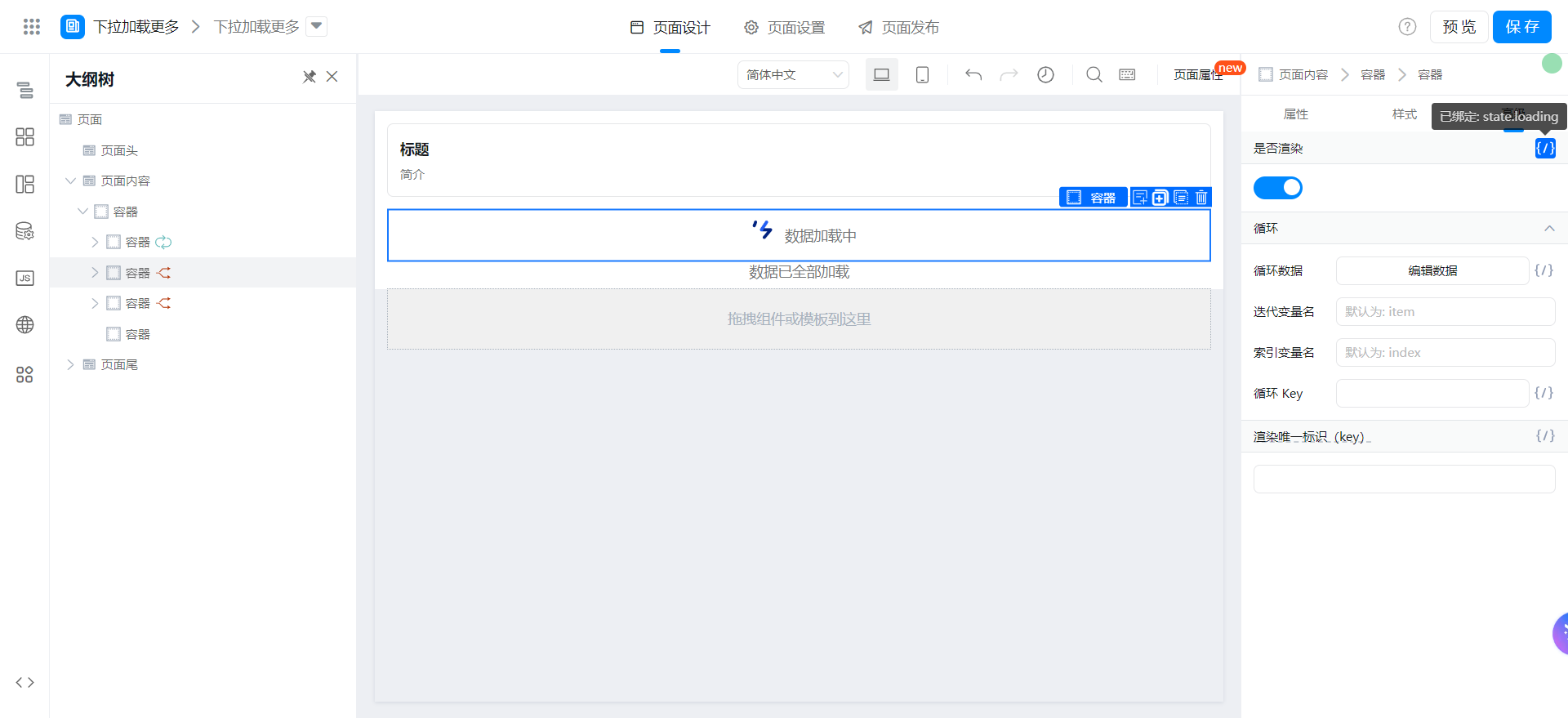
Container binding variables are loaded with all data:
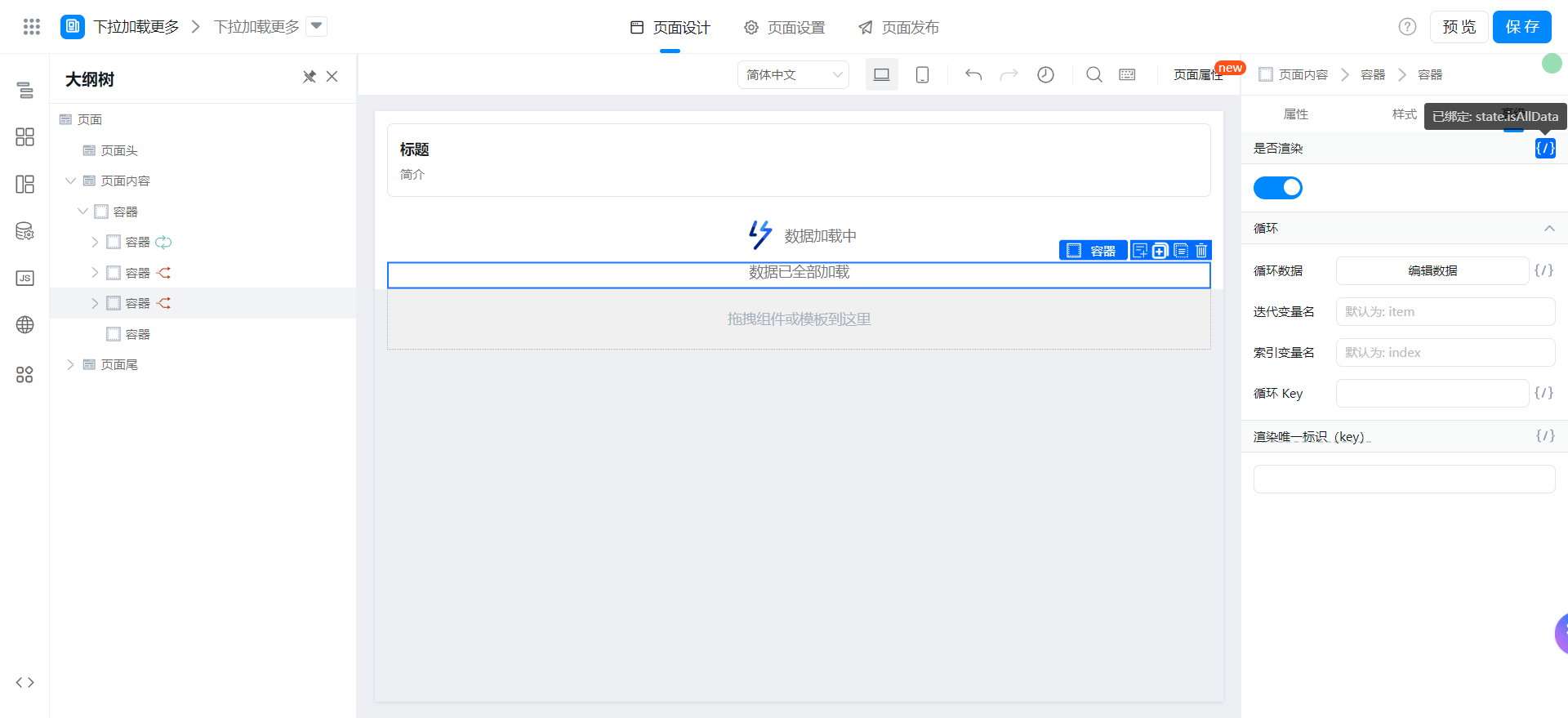
2.5. Configure page functions
Copy the following code to Page JS, call didMount function in scrollDownObserver, and modify the input parameter of the function according to the actual situation. The unique identifier in the input parameter of the function is the unique identifier of the monitored container.
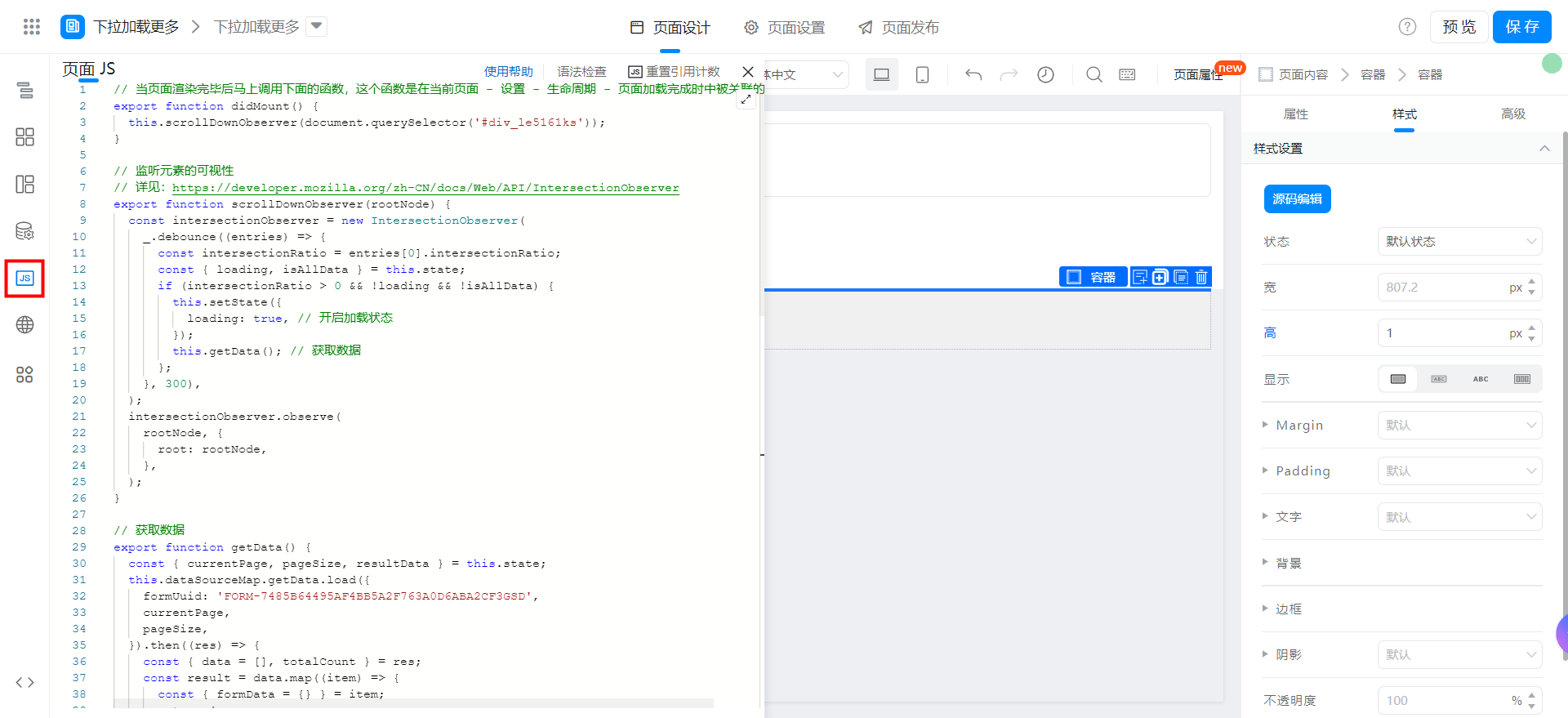
// 监听元素的可视性
// 详见:https://developer.mozilla.org/zh-CN/docs/Web/API/IntersectionObserver
export function scrollDownObserver(rootNode) {
const intersectionObserver = new IntersectionObserver(
_.debounce((entries) => {
const intersectionRatio = entries[0].intersectionRatio;
const { loading, isAllData } = this.state;
if (intersectionRatio > 0 && !loading && !isAllData) {
this.setState({
loading: true, // 开启加载状态
});
this.getData(); // 获取数据
};
}, 300),
);
intersectionObserver.observe(
rootNode, {
root: rootNode,
},
);
}
// 获取数据
export function getData() {
const { currentPage, pageSize, resultData } = this.state;
this.dataSourceMap.getData.load({
formUuid: 'FORM-7485B64495AF4BB5A2F763A0D6ABA2CF3GSD',
currentPage,
pageSize,
}).then((res) => {
const { data = [], totalCount } = res;
const result = data.map((item) => {
const { formData = {} } = item;
return {
title: formData.textField_ldxu659y,
desc: formData.textareaField_ldxuoi6d,
};
});
resultData.push(...result);
if (resultData.length === totalCount) {
// 数据已全部加载完毕
this.setState({
resultData,
isAllData: true,
loading: false,
});
} else {
this.setState({
currentPage: currentPage + 1,
resultData,
loading: false,
});
}
}).catch((error) => {
this.utils.toast({
title: error.message,
type: 'error',
});
});
}
Called in didMount:
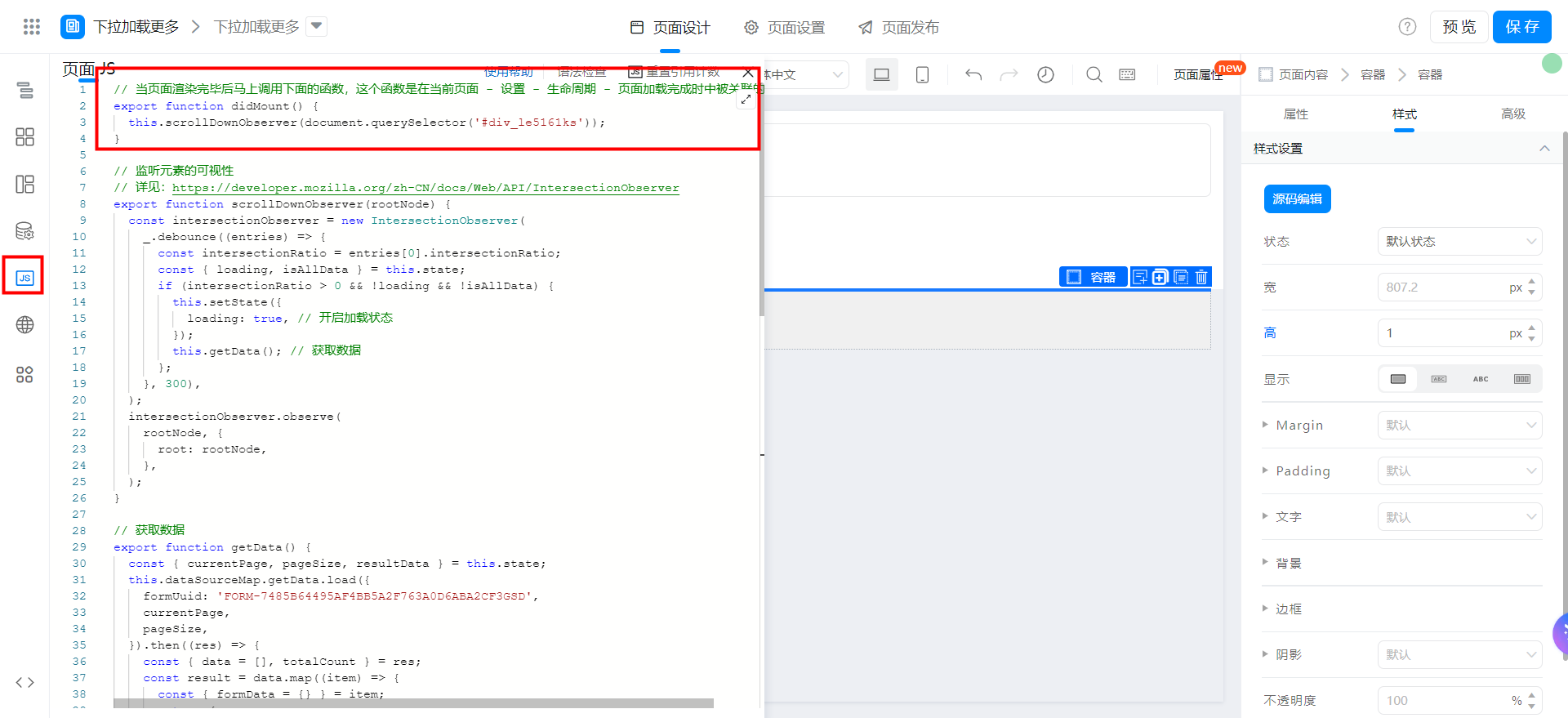
// 当页面渲染完毕后马上调用下面的函数,这个函数是在当前页面 - 设置 - 生命周期 - 页面加载完成时中被关联的。
export function didMount() {
this.scrollDownObserver(document.querySelector('#div_le5161ks'));
}
3. Effect
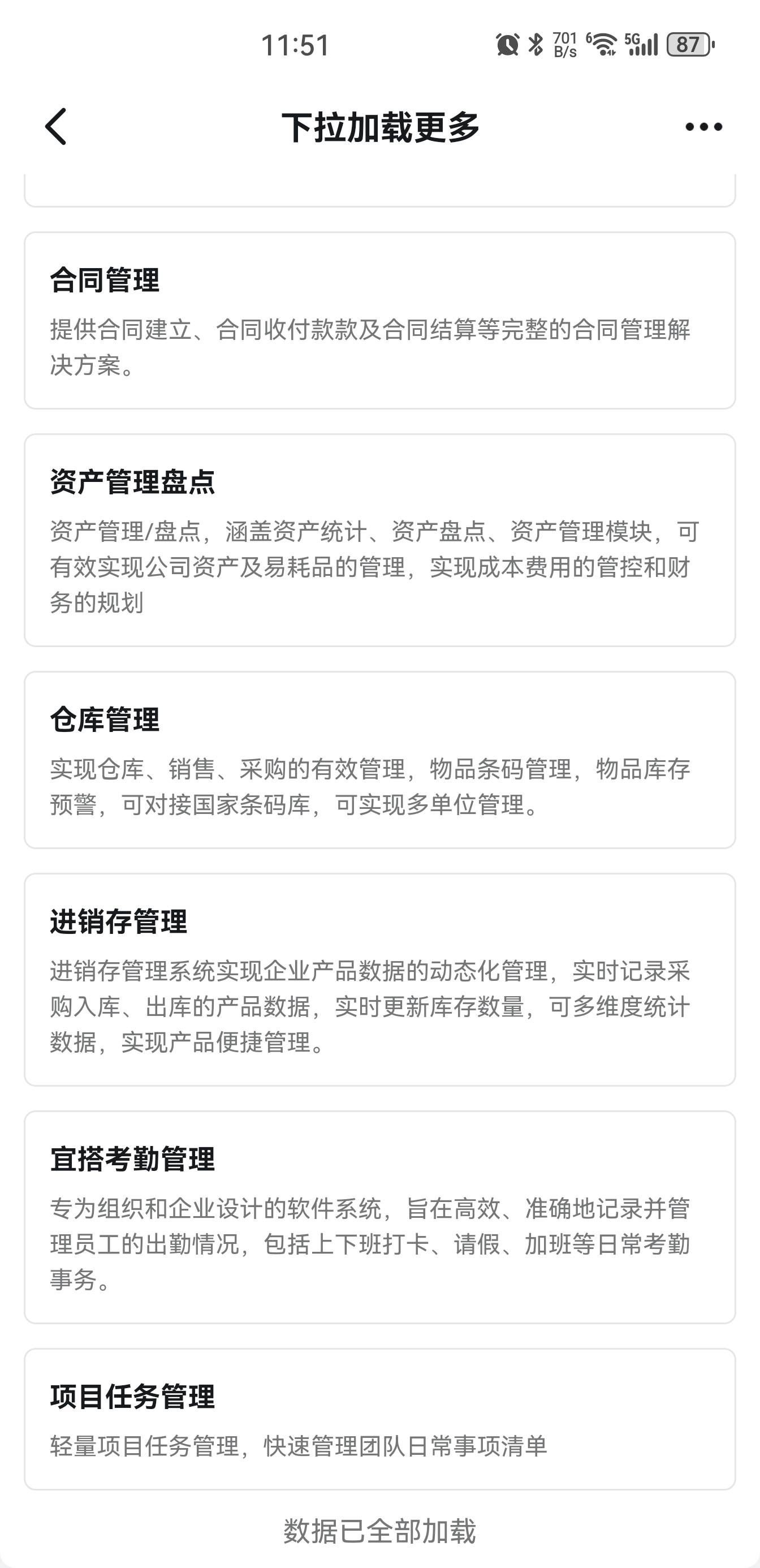