Convert Chinese to pinyin
In the process of building YIDA applications, you may encounter the following problems.
- In the CRM system, when entering the company name, supplier name, and contact name, you need to obtain the full spelling and uppercase letters of the name for standardized processing.
- In the enterprise mailbox management system, you can convert employee names to pinyin to automatically assign enterprise mailbox accounts.
- In the learning system, pinyin annotations can meet the learning needs of people of all ages.
Prerequisites
This tutorial uses the third-party Chinese to pinyin plug-in. You can first understandpinyin-pro.js
, for more information, seepinyinPro.js.
Effect
Pinyin Conversion
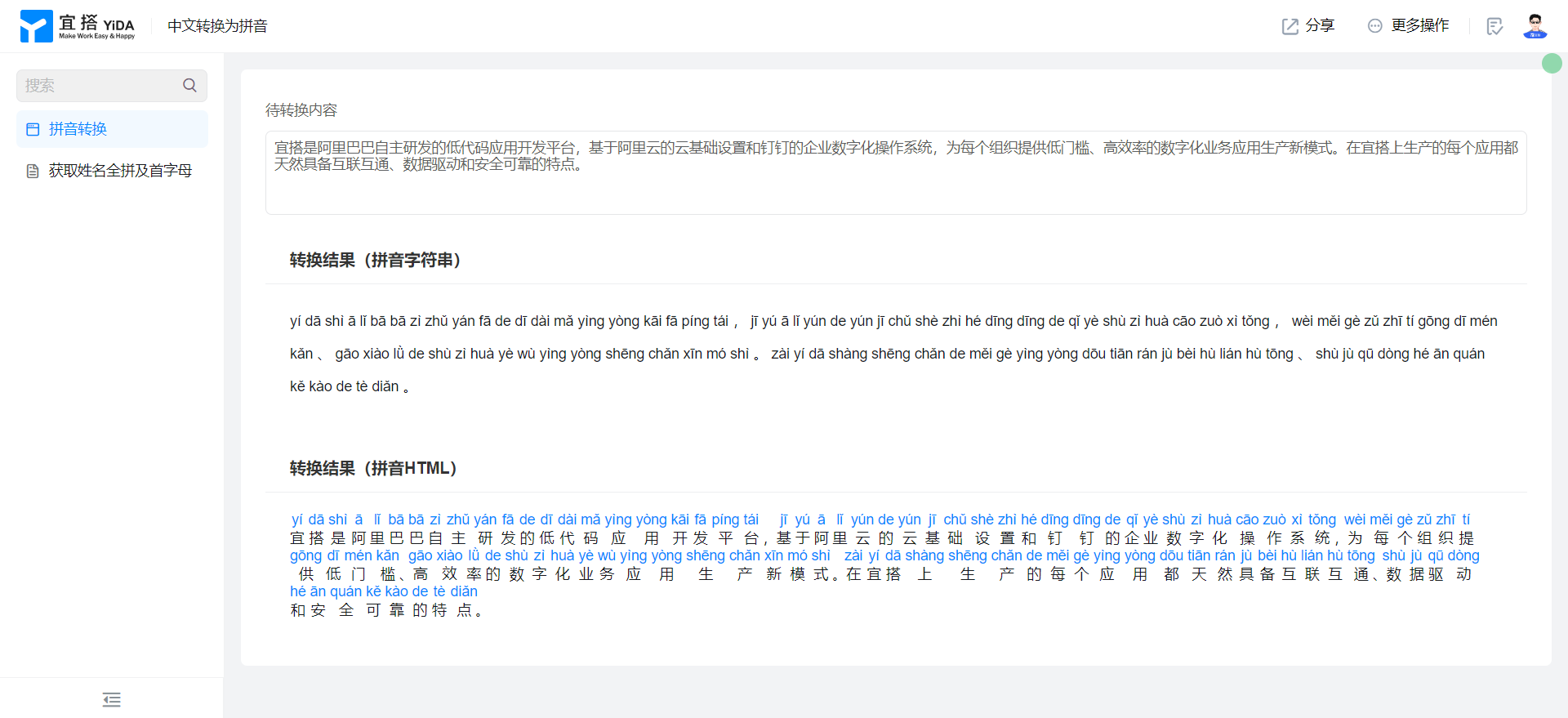
Get full name spelling and uppercase letter

Implementation steps
Pinyin Conversion
Create a custom page
Create a custom page. For more information, seeCustom page.

Drag the following components into the canvas area.
- Multi-line text: name the content to be converted
- Multi-line text: Named as the conversion result (pinyin string).
- HTML: The conversion result.

Introduce a third-party Chinese to pinyin plug-in
In the actions panel of the left-side ribbon, importpinyinPro.js
.

// 当页面渲染完毕后马上调用下面的函数,这个函数是在当前页面 - 设置 - 生命周期 - 页面加载完成时中被关联的。
export function didMount() {
this.utils.loadScript('https://g.alicdn.com/code/lib/pinyin-pro/3.19.6/index.min.js').then(() => {
console.log('pinyinPro.js load success');
this.$('textareaField_lye6ycnl').setValue('宜搭是阿里巴巴自主研发的低代码应用开发平台,基于阿里云的云基础设置和钉钉的企业数字化操作系统,为每个组织提供低门槛、高效率的数字化业务应用生产新模式。在宜搭上生产的每个应用都天然具备互联互通、数据驱动和安全可靠的特点。');
});
}
The setValue in the fifth line of the code can be used as needed.
Configure page functions
Copy the following function to Page JS
/**
* 将中文转换为拼音
* @param text 需要转换拼音的内容
* @param options 配置项,可以参考下述文档
* API文档:https://pinyin-pro.cn/use/pinyin.html
*/
export function chinese2Pinyin(text = '', options = {}) {
if (text) {
const { pinyin } = pinyinPro;
return pinyin(text, options);
} else {
return '';
}
}
/**
* 将中文转换为拼音 HTML 字符串
* @param text 需要转换拼音 HTML 字符串的内容
* @param options 配置项,可以参考下述文档
* API文档:https://pinyin-pro.cn/use/html.html
*/
export function chinese2PinyinHTML(text = '', options = {}) {
if (text) {
const { html } = pinyinPro;
return html(text, options);
} else {
return '';
}
}
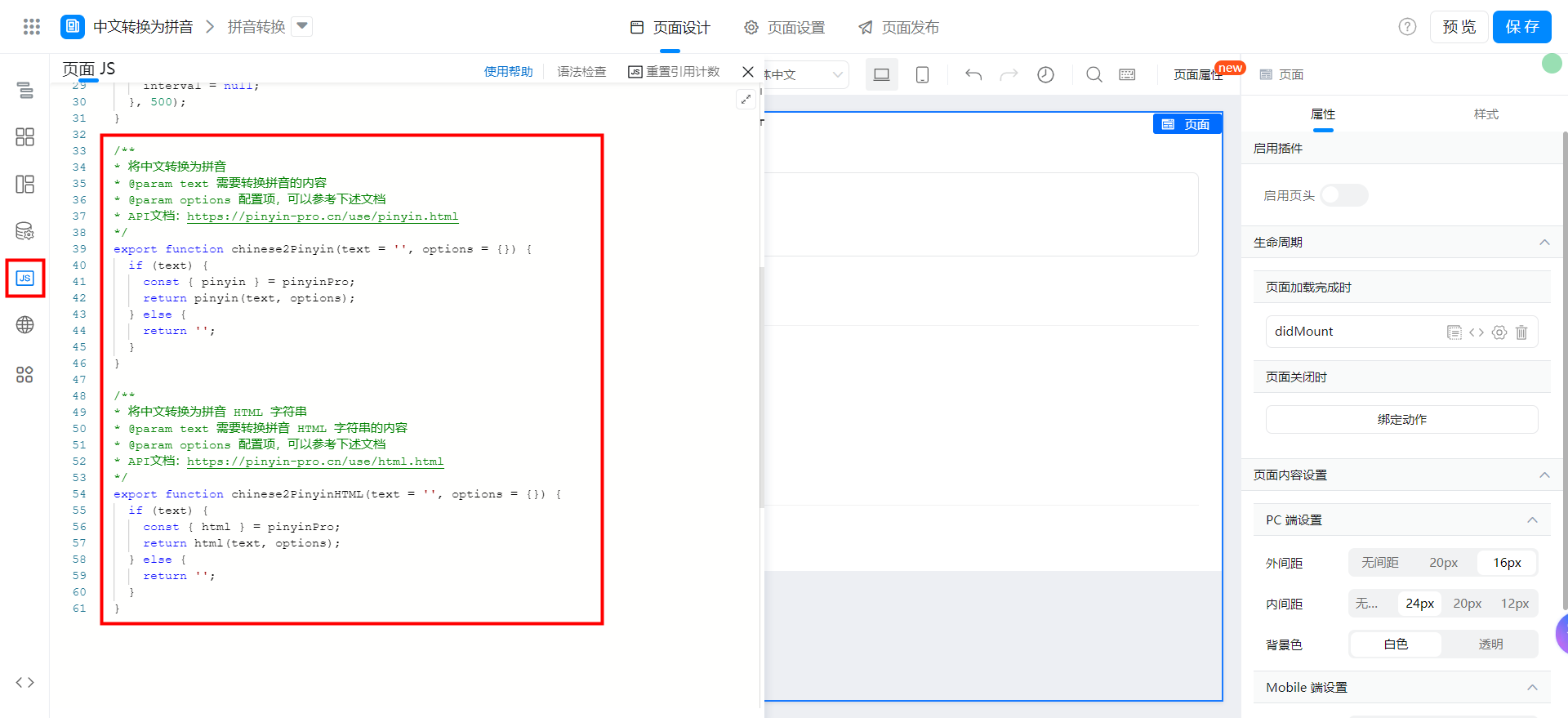
Bind events to the [content to be converted] component

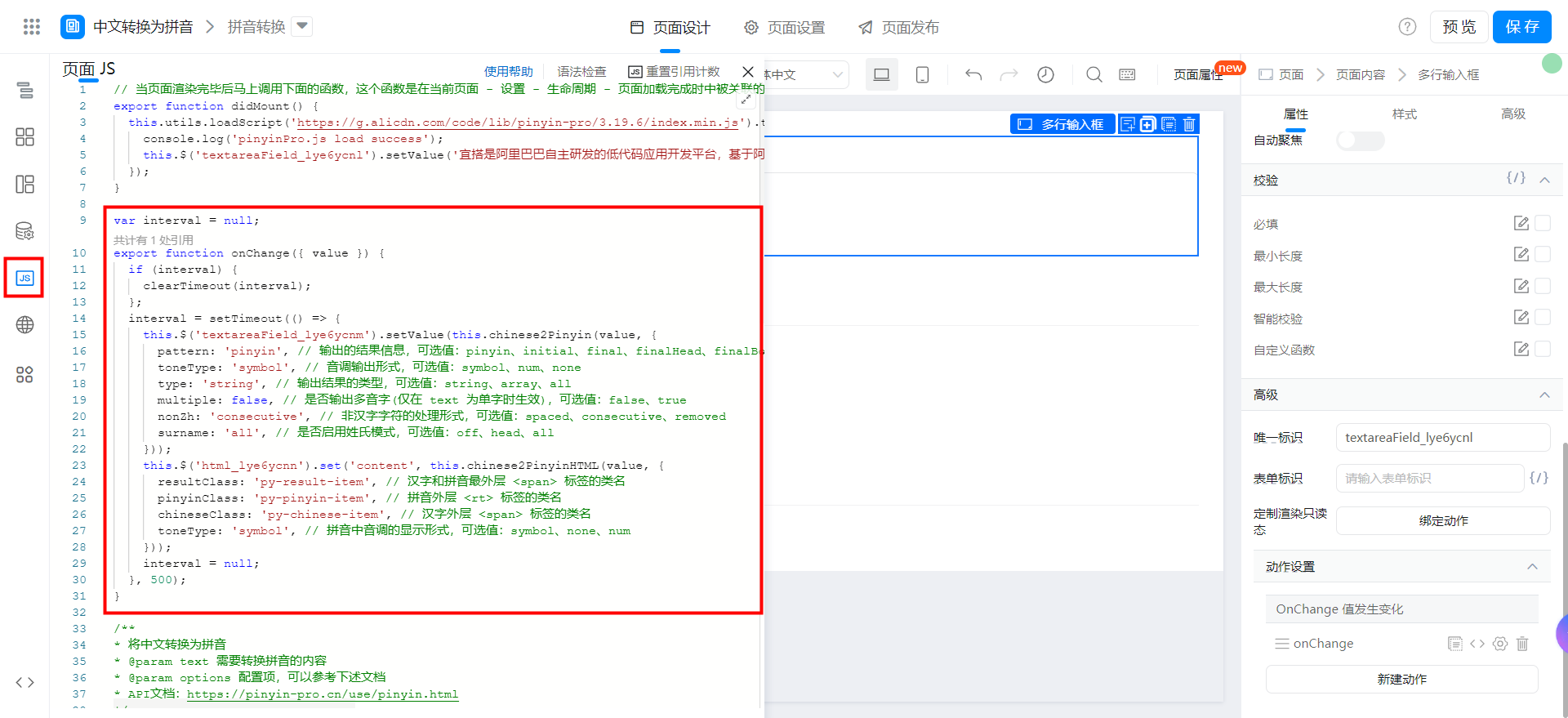
Note that the unique identifier of the component to be setValue is modified.
var interval = null;
export function onChange({ value }) {
if (interval) {
clearTimeout(interval);
};
interval = setTimeout(() => {
this.$('textareaField_lye6ycnm').setValue(this.chinese2Pinyin(value, {
pattern: 'pinyin', // 输出的结果信息,可选值:pinyin、initial、final、finalHead、finalBody、finalTail、num、first
toneType: 'symbol', // 音调输出形式,可选值:symbol、num、none
type: 'string', // 输出结果的类型,可选值:string、array、all
multiple: false, // 是否输出多音字(仅在 text 为单字时生效),可选值:false、true
nonZh: 'consecutive', // 非汉字字符的处理形式,可选值:spaced、consecutive、removed
surname: 'all', // 是否启用姓氏模式,可选值:off、head、all
}));
this.$('html_lye6ycnn').set('content', this.chinese2PinyinHTML(value, {
resultClass: 'py-result-item', // 汉字和拼音最外层 <span> 标签的类名
pinyinClass: 'py-pinyin-item', // 拼音外层 <rt> 标签的类名
chineseClass: 'py-chinese-item', // 汉字外层 <span> 标签的类名
toneType: 'symbol', // 拼音中音调的显示形式,可选值:symbol、none、num
}));
interval = null;
}, 500);
}
Configure the style of pinyin annotations (ON DEMAND)
- Locate the HTML component.
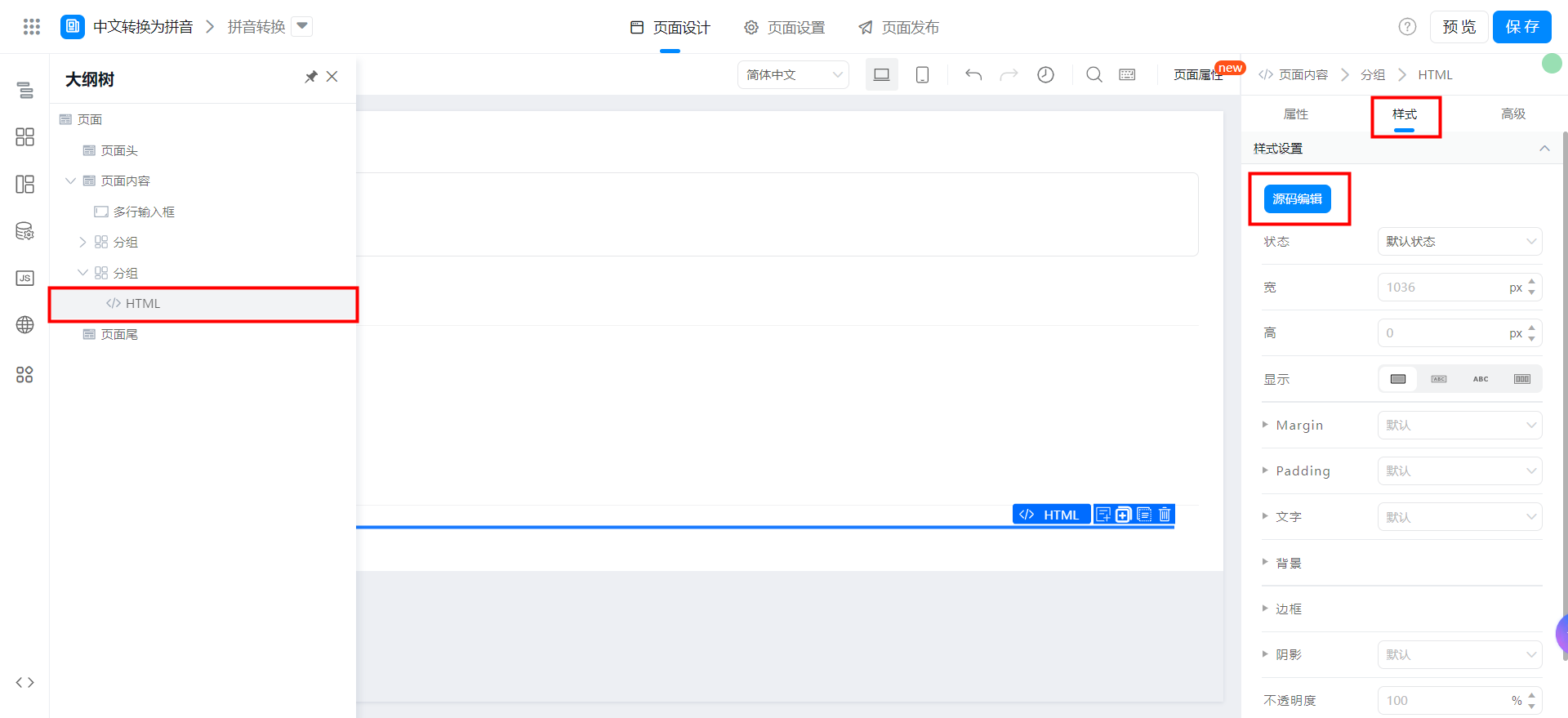
- Add the following style:
.py-result-item {
margin-right: 4px;
}
.py-chinese-item {
display: block;
font-size: 14px;
line-height: 1.5;
}
.py-pinyin-item {
font-size: 14px;
color: #0089FF;
}
The class name corresponds to the following:
- Overall (Chinese + pinyin)
- Chinese
- Pinyin
Get full name spelling and uppercase letter
Create a normal form page
Create a common form page. For more information, seeCommon form.
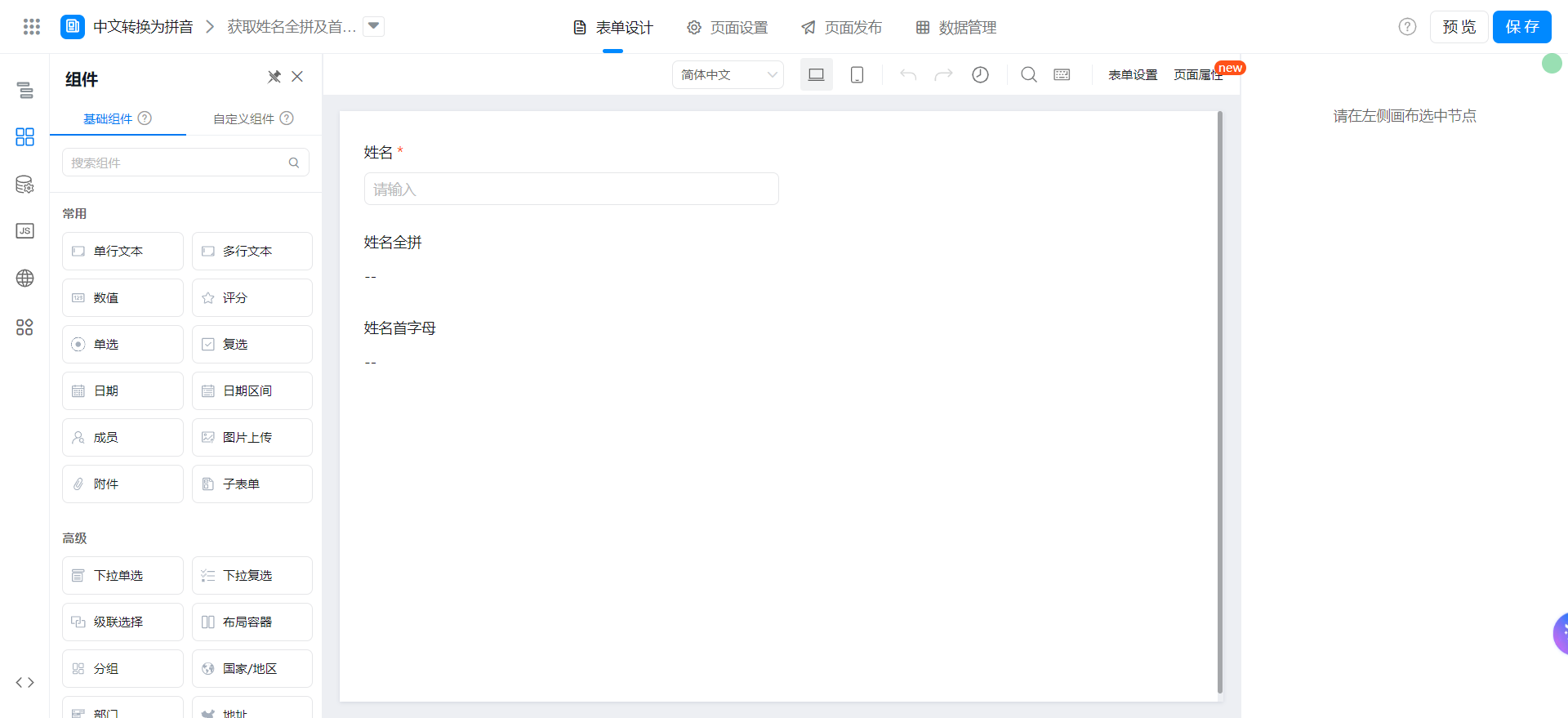
Drag the following components into the canvas area.
- Single line text: Named as name
- Single line text: name it full name
- Single line text: Named as the first letter of the name

Introduce a third-party Chinese to pinyin plug-in
In the actions panel of the left-side ribbon, importpinyinPro.js
.
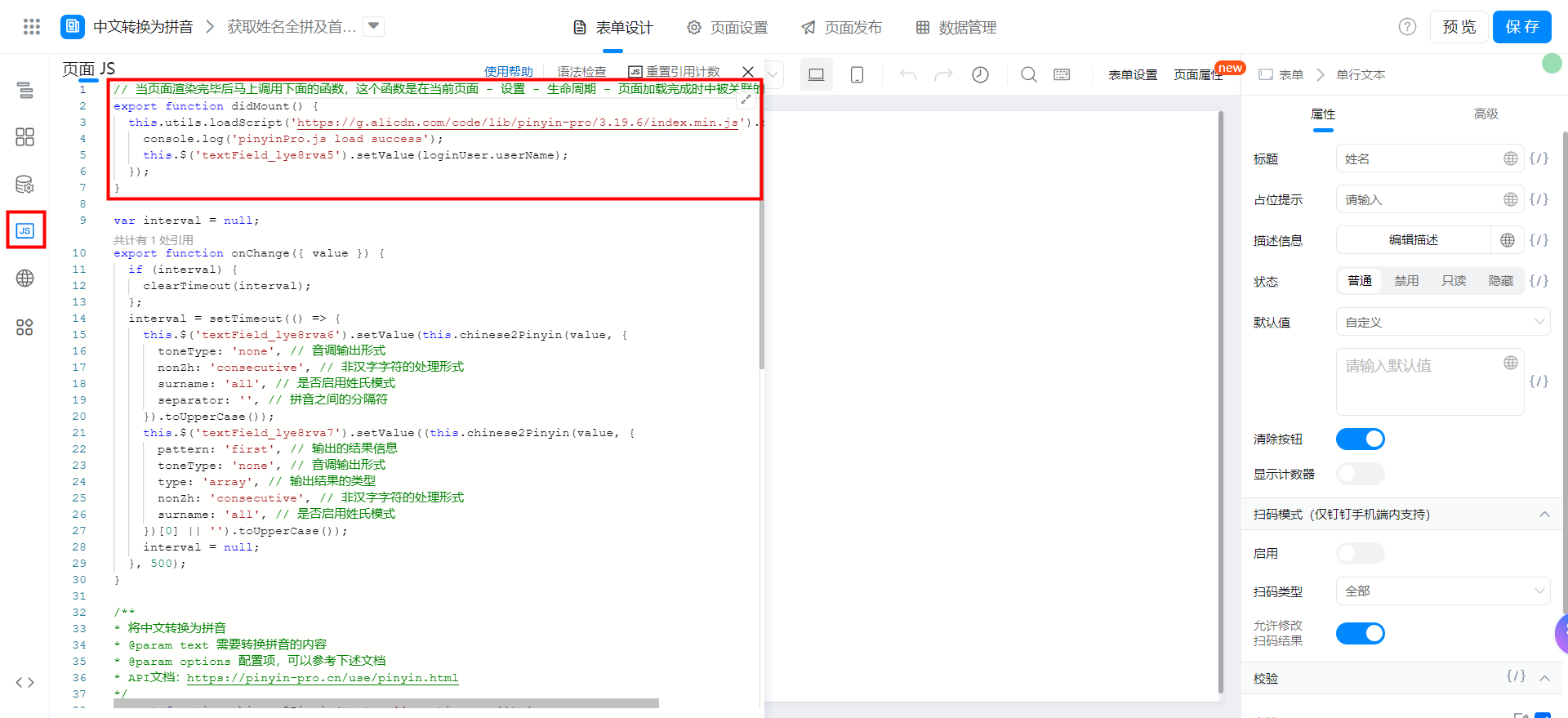
// 当页面渲染完毕后马上调用下面的函数,这个函数是在当前页面 - 设置 - 生命周期 - 页面加载完成时中被关联的。
export function didMount() {
this.utils.loadScript('https://g.alicdn.com/code/lib/pinyin-pro/3.19.6/index.min.js').then(() => {
console.log('pinyinPro.js load success');
this.$('textField_lye8rva5').setValue(loginUser.userName);
});
}
The setValue in the fifth line of the code can be used as needed.
Configure page functions
Copy the following function to Page JS
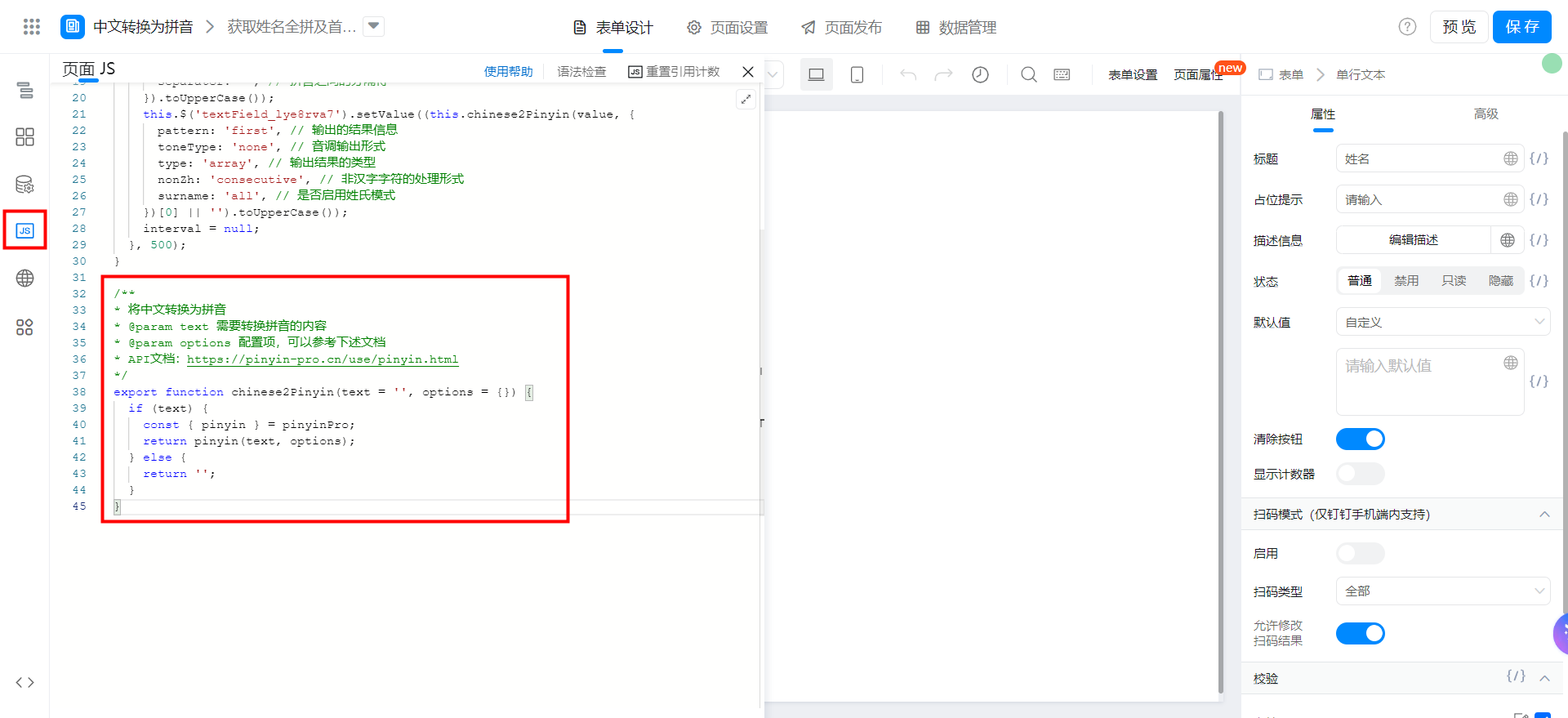
/**
* 将中文转换为拼音
* @param text 需要转换拼音的内容
* @param options 配置项,可以参考下述文档
* API文档:https://pinyin-pro.cn/use/pinyin.html
*/
export function chinese2Pinyin(text = '', options = {}) {
if (text) {
const { pinyin } = pinyinPro;
return pinyin(text, options);
} else {
return '';
}
}
Bind events to the [name] component
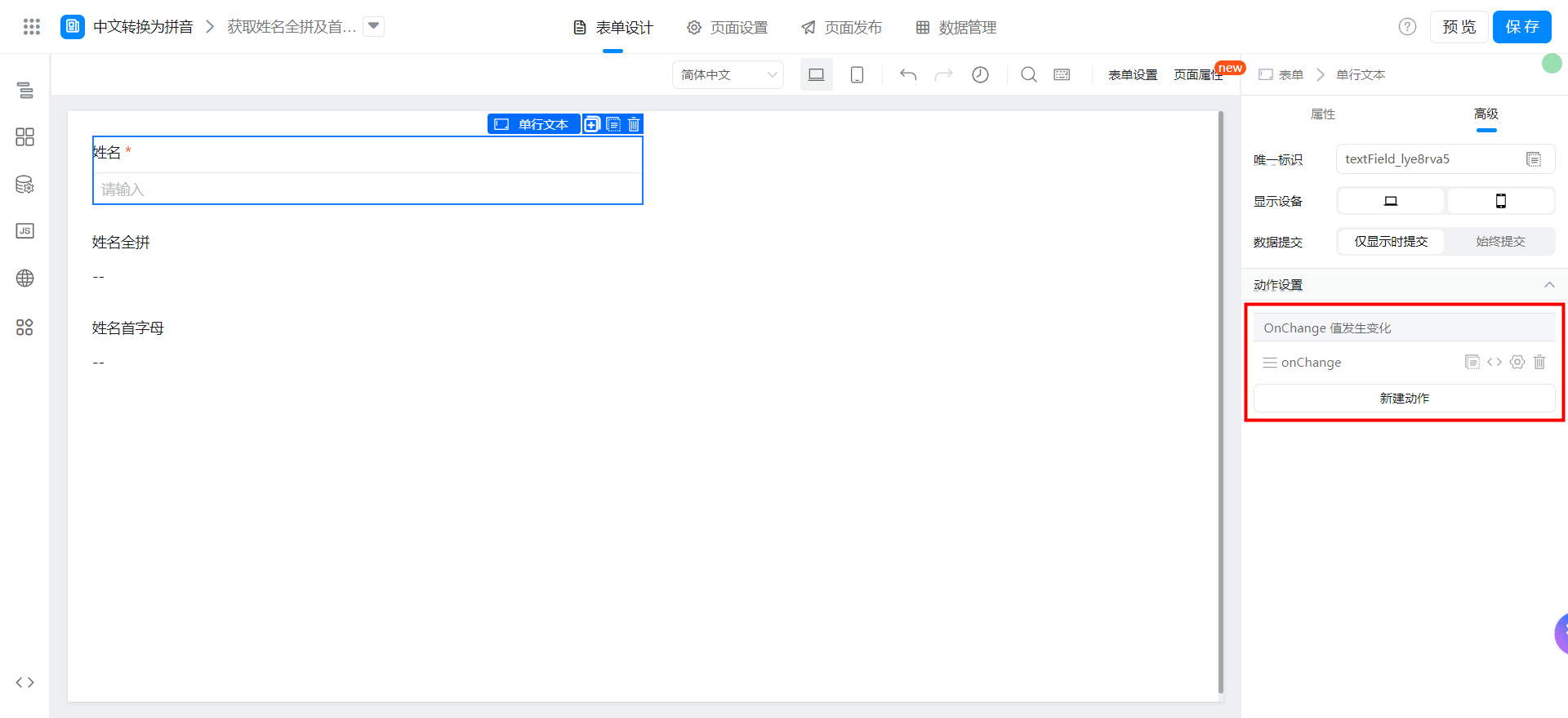
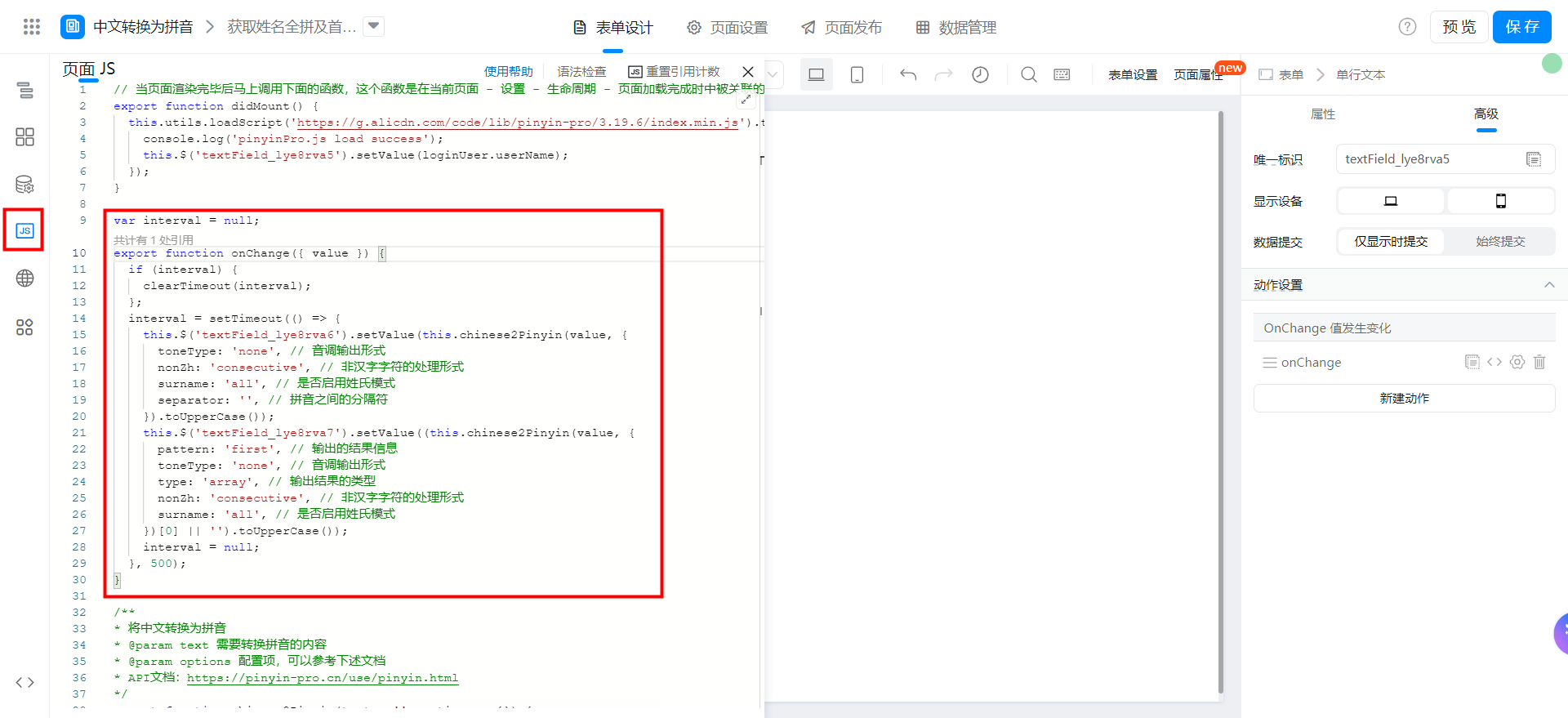
var interval = null;
export function onChange({ value }) {
if (interval) {
clearTimeout(interval);
};
interval = setTimeout(() => {
this.$('textField_lye8rva6').setValue(this.chinese2Pinyin(value, {
toneType: 'none', // 音调输出形式
nonZh: 'consecutive', // 非汉字字符的处理形式
surname: 'all', // 是否启用姓氏模式
separator: '', // 拼音之间的分隔符
}).toUpperCase());
this.$('textField_lye8rva7').setValue((this.chinese2Pinyin(value, {
pattern: 'first', // 输出的结果信息
toneType: 'none', // 音调输出形式
type: 'array', // 输出结果的类型
nonZh: 'consecutive', // 非汉字字符的处理形式
surname: 'all', // 是否启用姓氏模式
})[0] || '').toUpperCase());
interval = null;
}, 500);
}
Note that the unique identifier of the component to be setValue is modified.