DingTalk protocol link generation
In the process of building YIDA applications, you may encounter the following problems.
- When configuring the link cool application, fill in the custom link. I hope it can be opened within DingTalk after clicking, instead of jumping to the browser.
- Applications built in YIDA are opened from the DingTalk Workbench by default. The custom link configured on the page also needs to be opened in DingTalk
Prerequisites
This tutorial uses the third-party text copy plug-in. You can first understandclipboard.js
, for more information, seeclipboard.js.
Effect
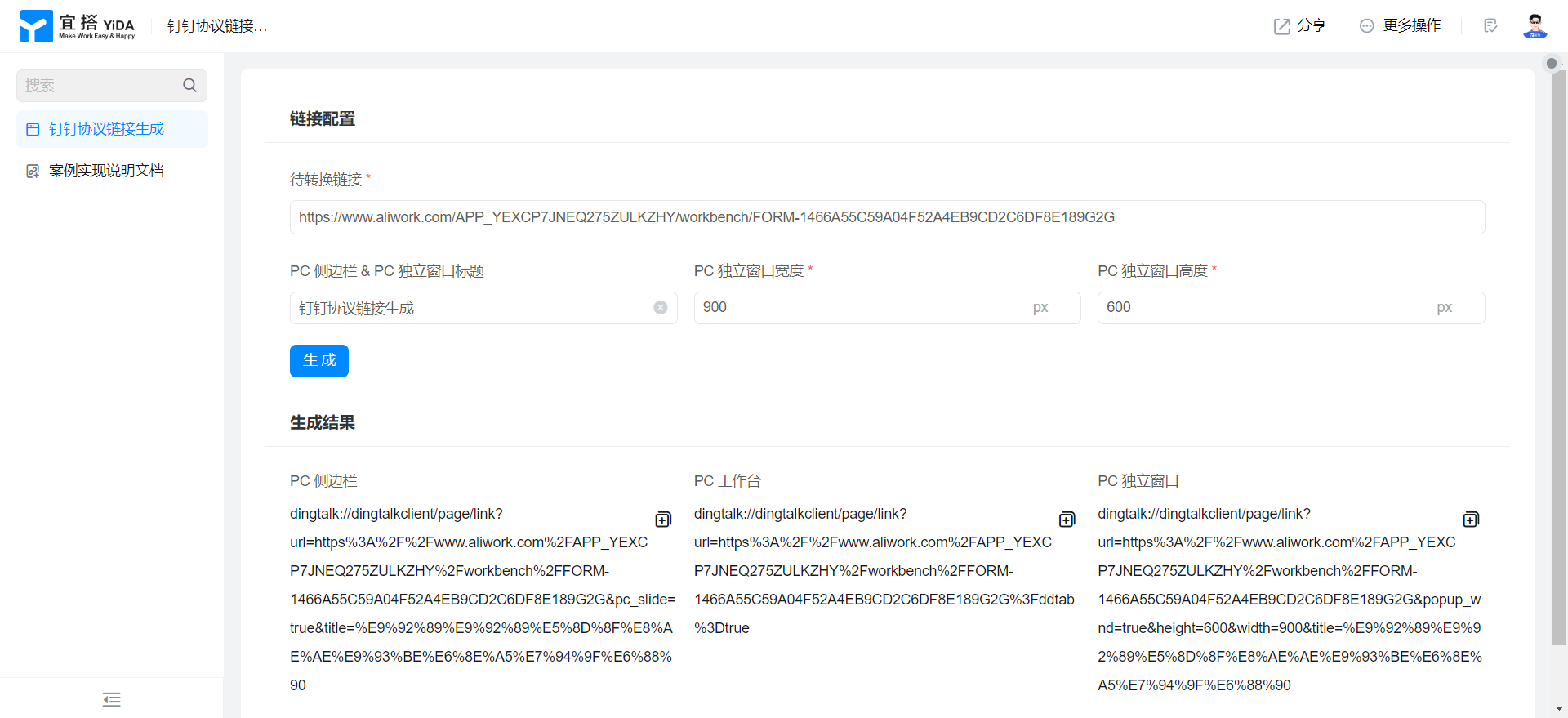

Implementation steps
Create a custom page
Create a custom page. For more information, seeCustom page.

Drag the following components into the canvas area.
- Multi-line input box: Named as the link to be converted
- Input box: Named PC sidebar & PC independent window title
- Digital input box: Named PC independent window width
- Digital input box: Named PC independent window height
- Input box: Named PC sidebar
- Input box: Named PC Workbench
- Input box: Named PC independent window
Introduce a third-party text replication plug-in
In the actions panel of the left-side ribbon, importclipboard.js
.

// 当页面渲染完毕后马上调用下面的函数,这个函数是在当前页面 - 设置 - 生命周期 - 页面加载完成时中被关联的。
export function didMount() {
// 初始化复制
this.utils.loadScript("https://g.alicdn.com/code/lib/clipboard.js/2.0.11/clipboard.min.js")
.then(() => {
const clipboard = new ClipboardJS('.btn');
clipboard.on('success', () => {
this.utils.toast({
title: `已复制到粘贴板`,
type: 'success'
});
});
})
}
Add tool functions
In the actions panel of the left-side ribbon, add the following functions.
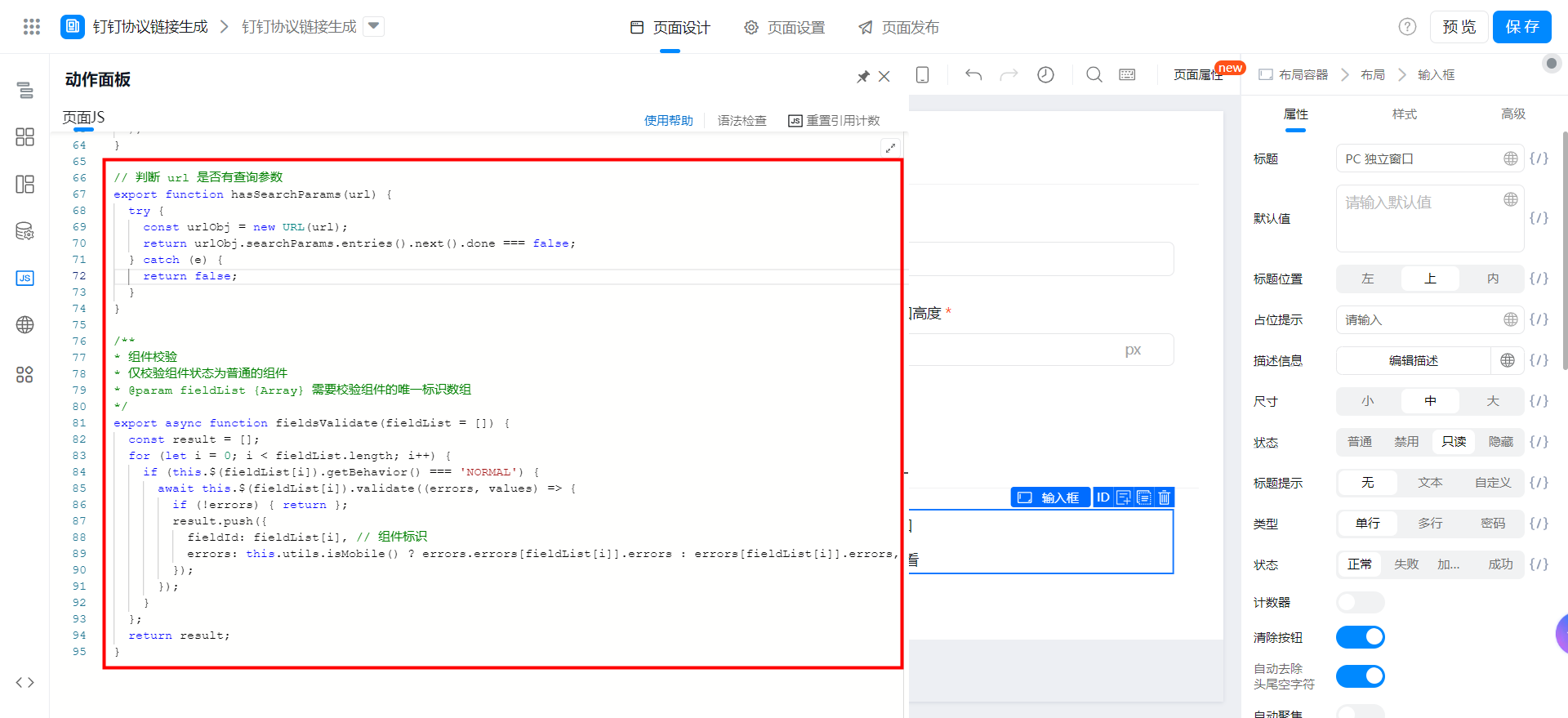
// 判断 url 是否有查询参数
export function hasSearchParams(url) {
try {
const urlObj = new URL(url);
return urlObj.searchParams.entries().next().done === false;
} catch (e) {
return false;
}
}
/**
* 组件校验
* 仅校验组件状态为普通的组件
* @param fieldList {Array} 需要校验组件的唯一标识数组
*/
export async function fieldsValidate(fieldList = []) {
const result = [];
for (let i = 0; i < fieldList.length; i++) {
if (this.$(fieldList[i]).getBehavior() === 'NORMAL') {
await this.$(fieldList[i]).validate((errors, values) => {
if (!errors) { return };
result.push({
fieldId: fieldList[i], // 组件标识
errors: this.utils.isMobile() ? errors.errors[fieldList[i]].errors : errors[fieldList[i]].errors, // 校验错误信息
});
});
}
};
return result;
}
Configure page functions
Bind an event to the link to be converted component.
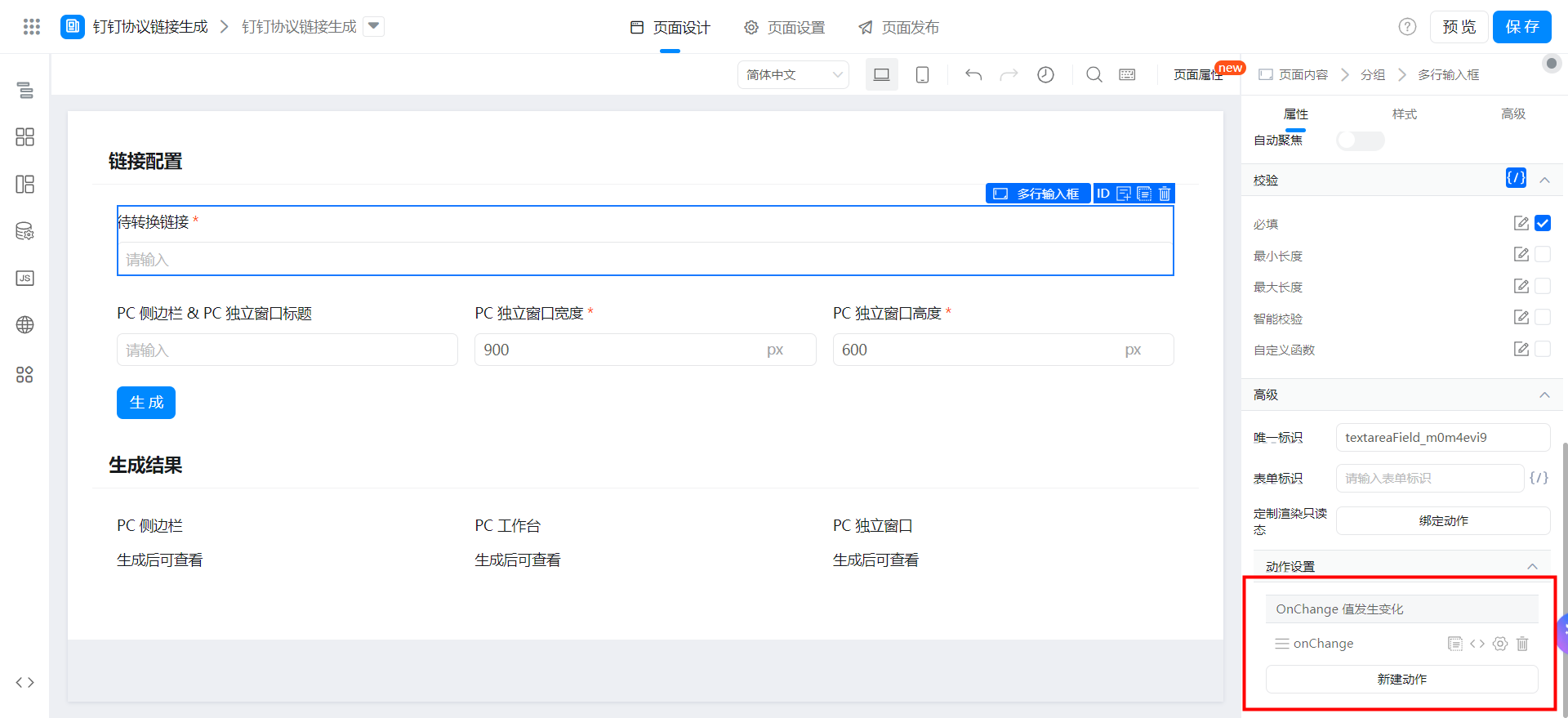

Note that the unique identifier of the component is modified.
export function onChange({ value }) {
if (!value) {
this.$('textField_lahvtneg').reset();
this.$('textField_lahvtneh').reset();
this.$('textField_lahvtnei').reset();
};
}
Bind events to the Generate button component.
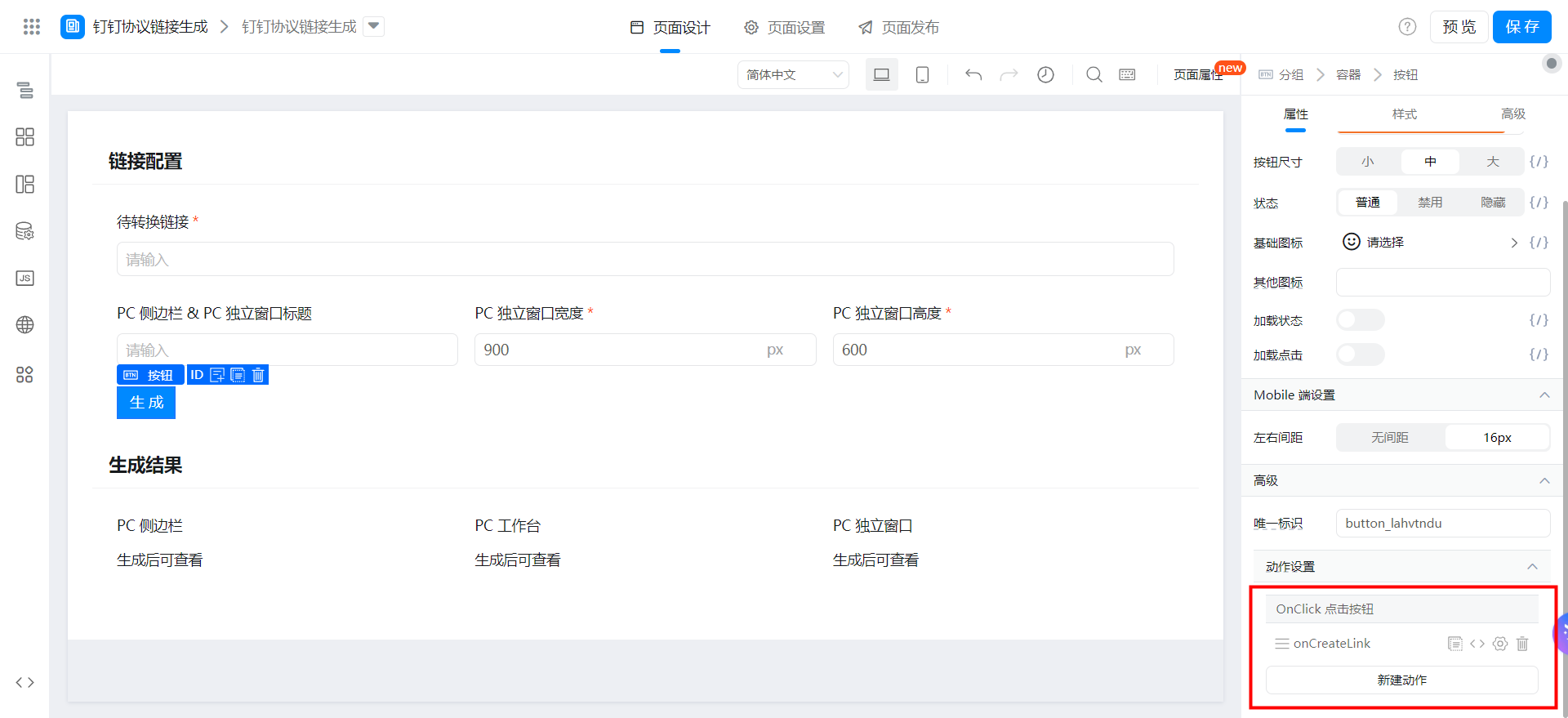

Note that the unique identifier of the component is modified.
// 链接生成
export function onCreateLink() {
const fieldList = [
'textareaField_m0m4evi9',
'numberField_lahvtnej',
'numberField_lahvtneo',
];
this.fieldsValidate(fieldList).then((errorList) => {
setTimeout(() => {
if (errorList.length > 0) {
// 表单校验未通过,可做一些数据错误提示
console.log(errorList);
return;
};
// 表单校验通过,你的后续业务逻辑由此往下写
const link = this.$('textareaField_m0m4evi9').getValue();
const width = this.$('numberField_lahvtnej').getValue();
const height = this.$('numberField_lahvtneo').getValue();
const title = this.$('textField_m0m4evhz').getValue();
const hasSearchParams = this.hasSearchParams(link);
this.$('textField_lahvtneg').setValue(`dingtalk://dingtalkclient/page/link?url=${encodeURIComponent(link)}&pc_slide=true&title=${encodeURIComponent(title)}`);
this.$('textField_lahvtneh').setValue(`dingtalk://dingtalkclient/page/link?url=${encodeURIComponent(link + `${hasSearchParams ? '&' : '?'}ddtab=true`)}`);
this.$('textField_lahvtnei').setValue(`dingtalk://dingtalkclient/page/link?url=${encodeURIComponent(link)}&popup_wnd=true&height=${height}&width=${width}&title=${encodeURIComponent(title)}`);
});
}, 0);
}
Configure a custom rendering read-only state for the PC sidebar, PC workbench, and PC independent window components. The three components are bound to the same event.


export function renderView(value, props) {
return (
<div>
{
value ?
<span className="btn" data-clipboard-text={value}>
<i className="next-icon next-icon-copy next-medium vc-icon" style={{ "float": "right", "fontSize": "10px", "padding": "4px", "verticalAlign": "top", "cursor": "pointer" }}></i>
</span> :
<div></div>
}
{value || '生成后可查看'}
</div>
);
}
Save page
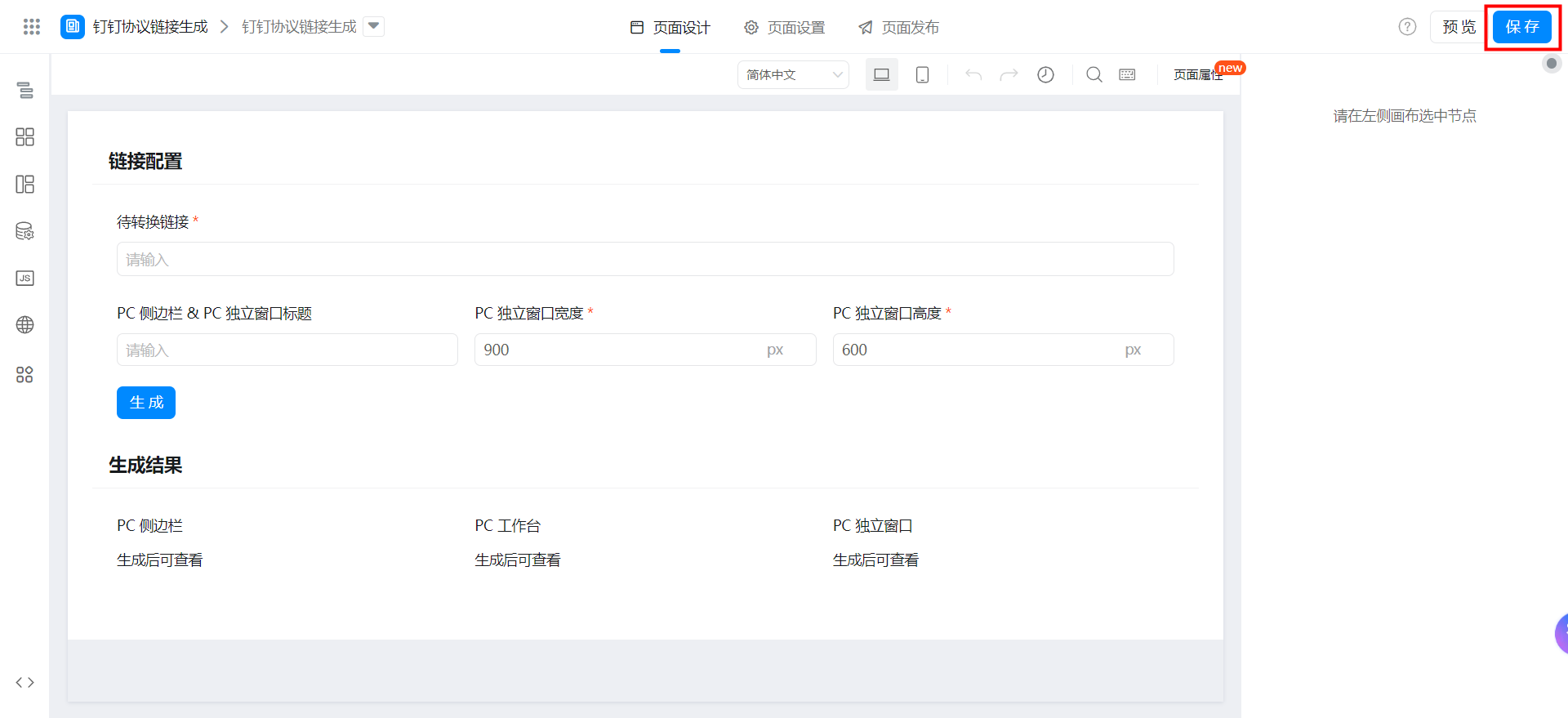