Optional interval for implementing custom dates in the form
1. Usage scenarios
With the help of custom verification functions or YIDA JSAPI, we can implement some complex custom date optional intervals. This example shows how to implement custom date optional intervals in YIDA.
2. Implement functions
2.1. Form configuration
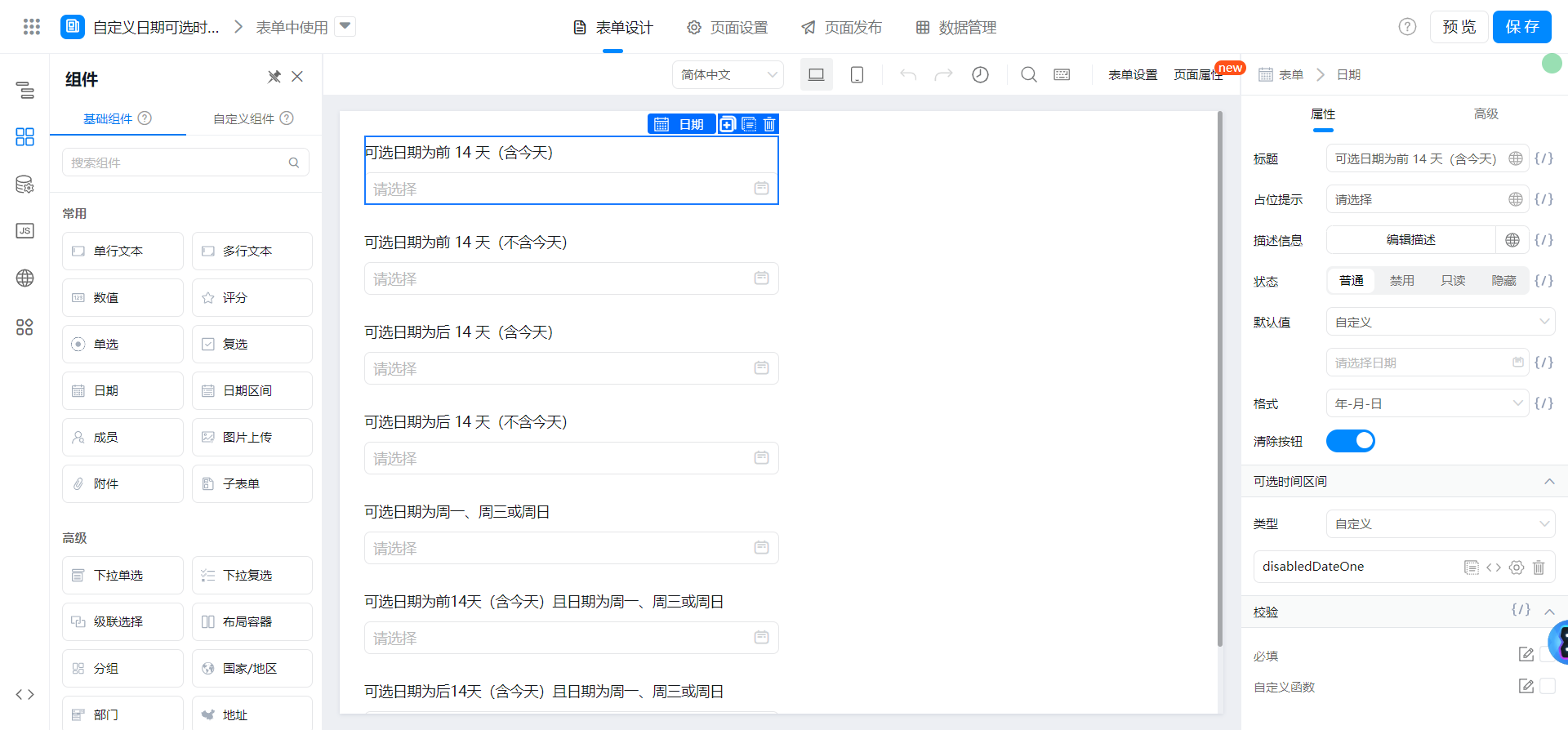
2.2. Optional interval function for configuring custom dates
Copy the following function to Page JS.
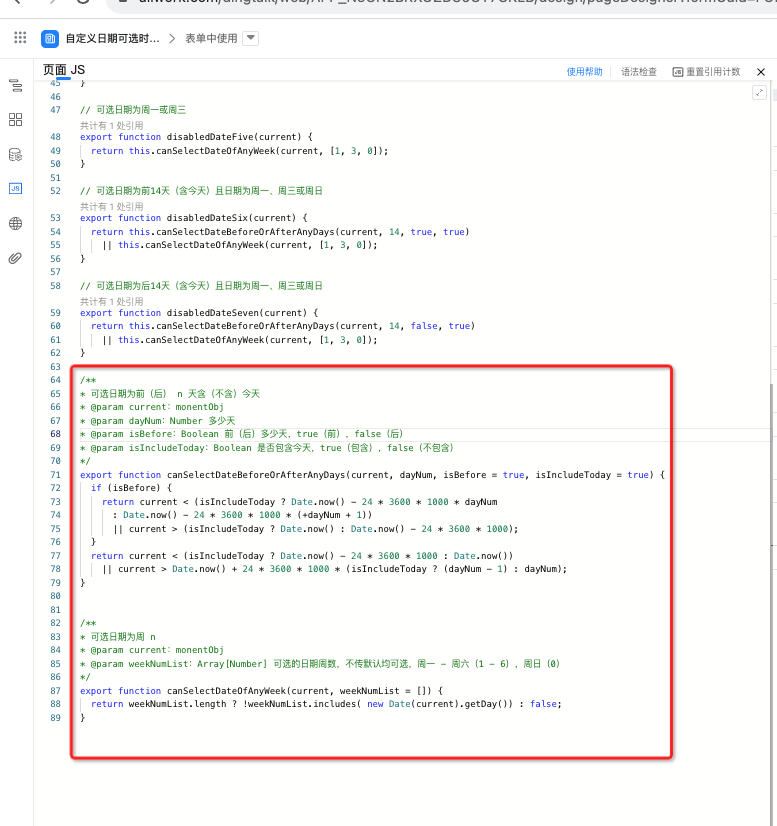
/**
* 可选日期为前(后) n 天含(不含)今天
* @param current:monentObj
* @param dayNum:Number 多少天
* @param isBefore:Boolean 前(后)多少天,true(前),false(后)
* @param isIncludeToday:Boolean 是否包含今天,true(包含),false(不包含)
*/
export function canSelectDateBeforeOrAfterAnyDays(current, dayNum, isBefore = true, isIncludeToday = true) {
if (isBefore) {
return current < (isIncludeToday ? Date.now() - 24 * 3600 * 1000 * dayNum
: Date.now() - 24 * 3600 * 1000 * (+dayNum + 1))
|| current > (isIncludeToday ? Date.now() : Date.now() - 24 * 3600 * 1000);
}
return current < (isIncludeToday ? Date.now() - 24 * 3600 * 1000 : Date.now())
|| current > Date.now() + 24 * 3600 * 1000 * (isIncludeToday ? (dayNum - 1) : dayNum);
}
/**
* 可选日期为周 n
* @param current:monentObj
* @param weekNumList:Array[Number] 可选的日期周数,不传默认均可选,周一 - 周六(1 - 6),周日(0)
*/
export function canSelectDateOfAnyWeek(current, weekNumList = []) {
return weekNumList.length ? !weekNumList.includes( new Date(current).getDay()) : false;
}
2.3. Used in the date component
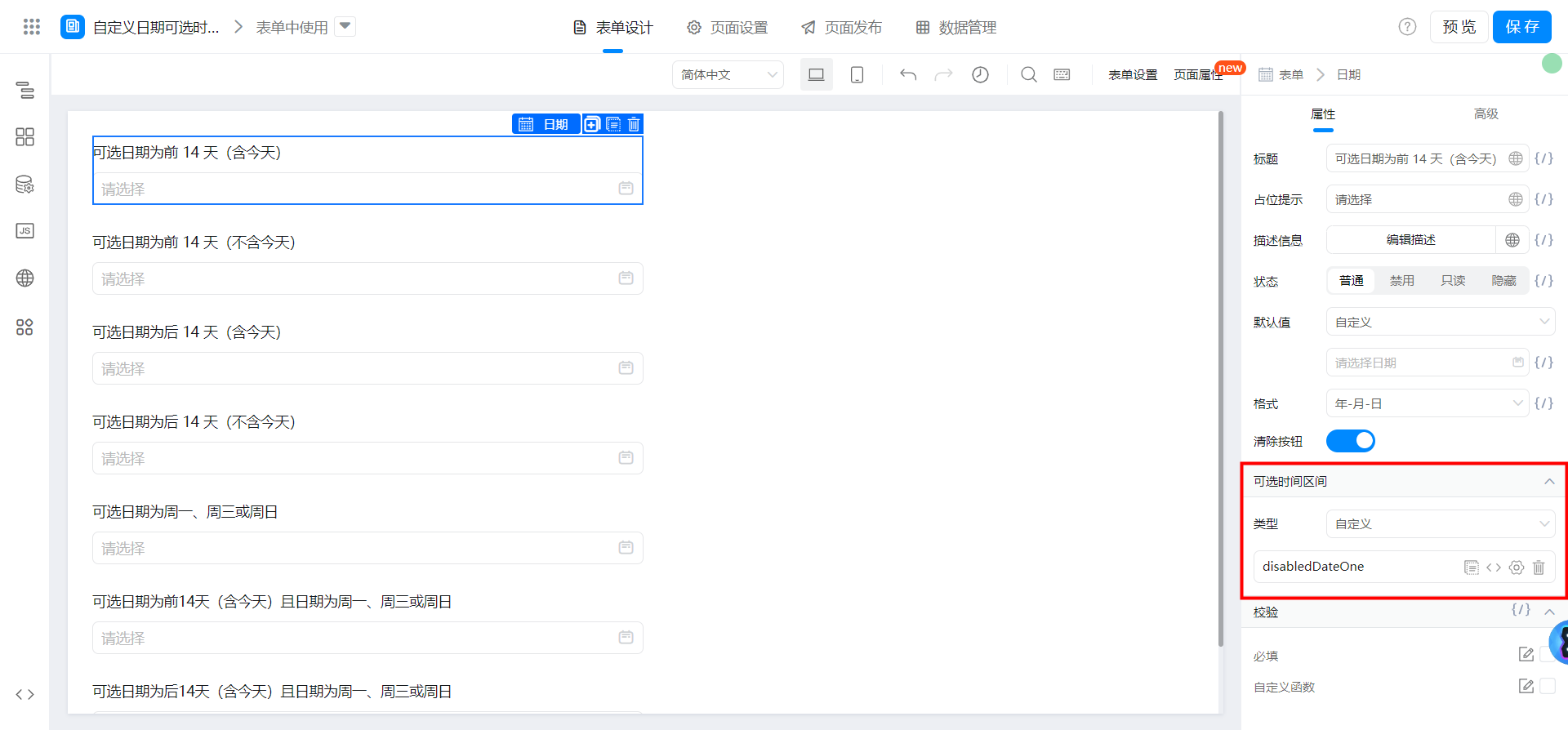
// 可选日期为前14天(含今天)
export function disabledDateOne(current) {
return this.canSelectDateBeforeOrAfterAnyDays(current, 14, true, true);
}
// 可选日期为前14天(不含今天)
export function disabledDateTwo(current) {
return this.canSelectDateBeforeOrAfterAnyDays(current, 14, true, false);
}
// 可选日期为后14天(含今天)
export function disabledDateThree(current) {
return this.canSelectDateBeforeOrAfterAnyDays(current, 14, false, true);
}
// 可选日期为后14天(不含今天)
export function disabledDateFour(current) {
return this.canSelectDateBeforeOrAfterAnyDays(current, 14, false, false);
}
// 可选日期为周一、周三或周日
export function disabledDateFive(current) {
return this.canSelectDateOfAnyWeek(current, [1, 3, 0]);
}
// 可选日期为前14天(含今天)且日期为周一、周三或周日
export function disabledDateSix(current) {
return this.canSelectDateBeforeOrAfterAnyDays(current, 14, true, true)
|| this.canSelectDateOfAnyWeek(current, [1, 3, 0]);
}
// 可选日期为后14天(含今天)且日期为周一、周三或周日
export function disabledDateSeven(current) {
return this.canSelectDateBeforeOrAfterAnyDays(current, 14, false, true)
|| this.canSelectDateOfAnyWeek(current, [1, 3, 0]);
}
3. Effect
3.1. The optional date is the first 14 days (including today)
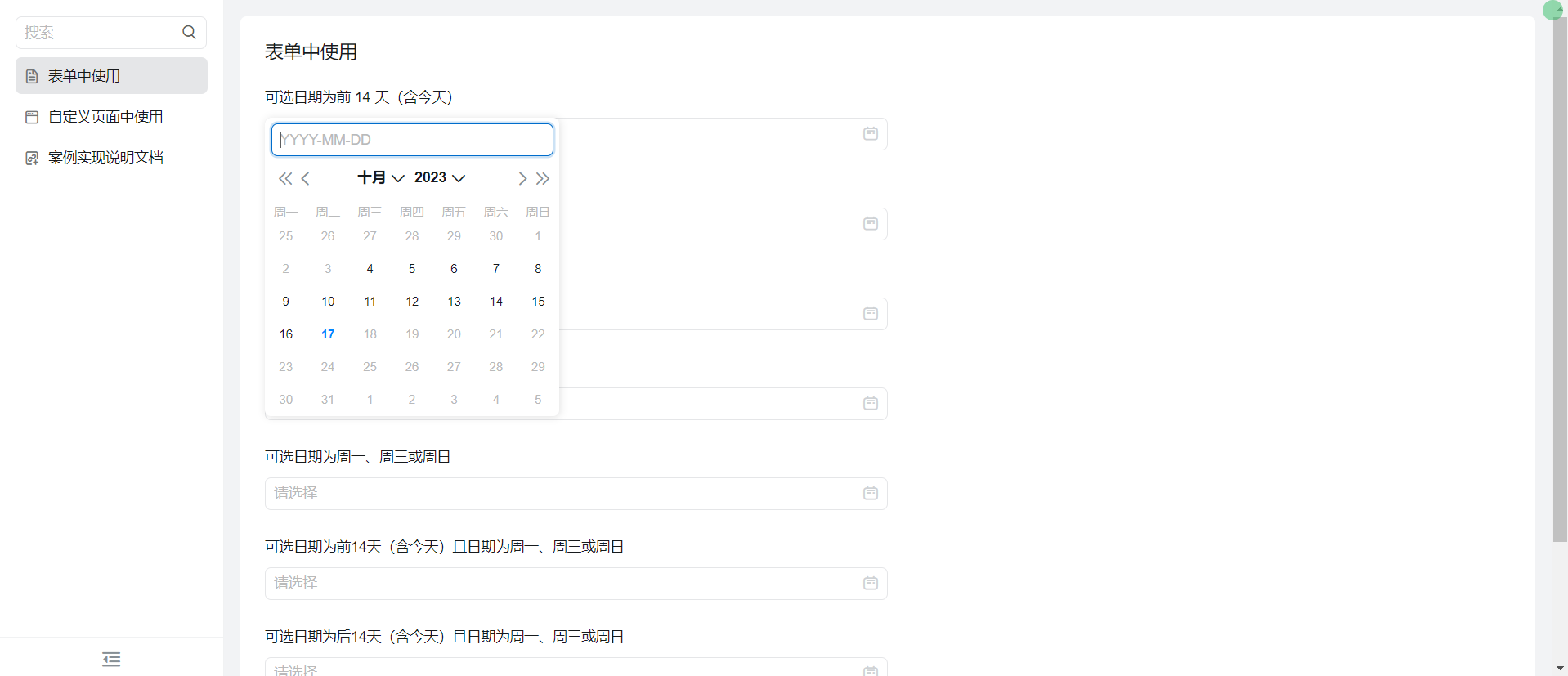
3.2. The optional date is the first 14 days (excluding today)
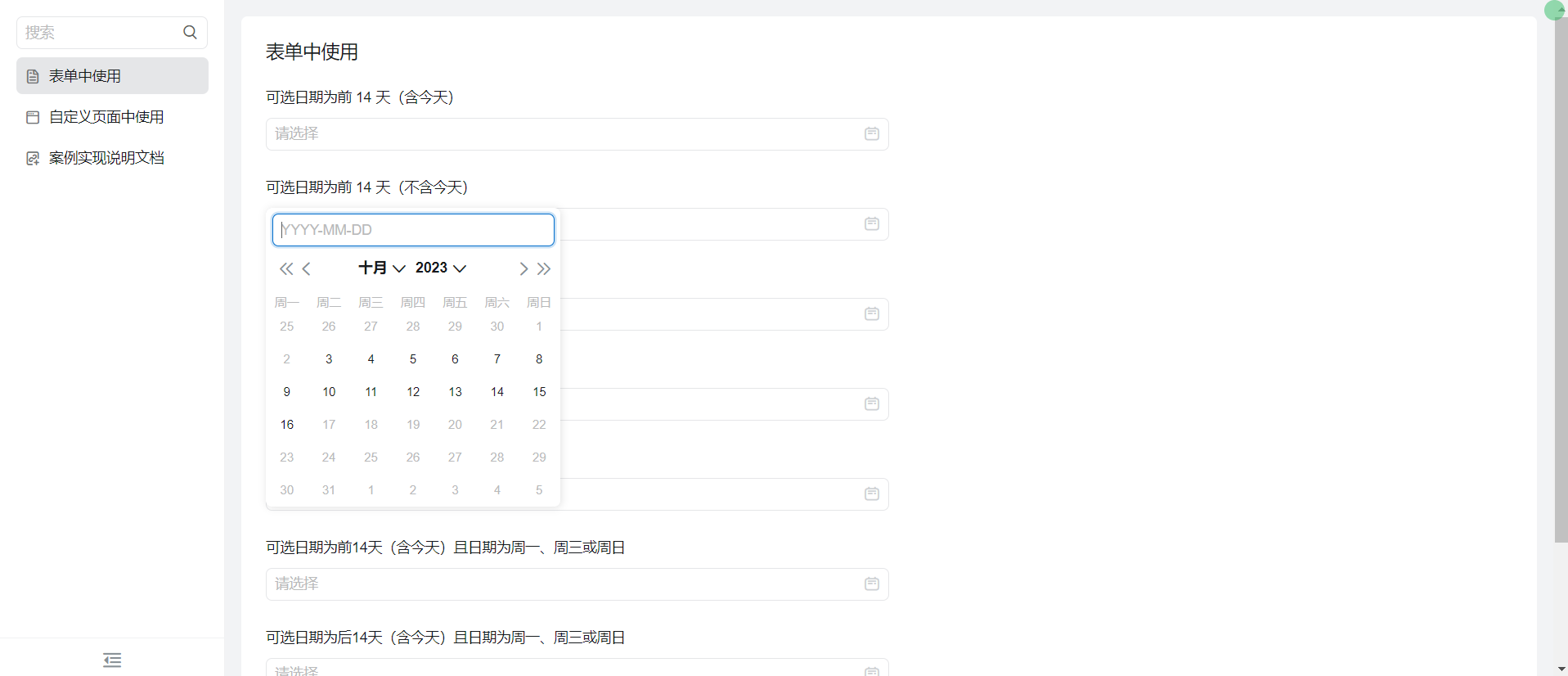
3.3. The optional date is the last 14 days (including today) and the date is Monday, Wednesday, or Sunday.
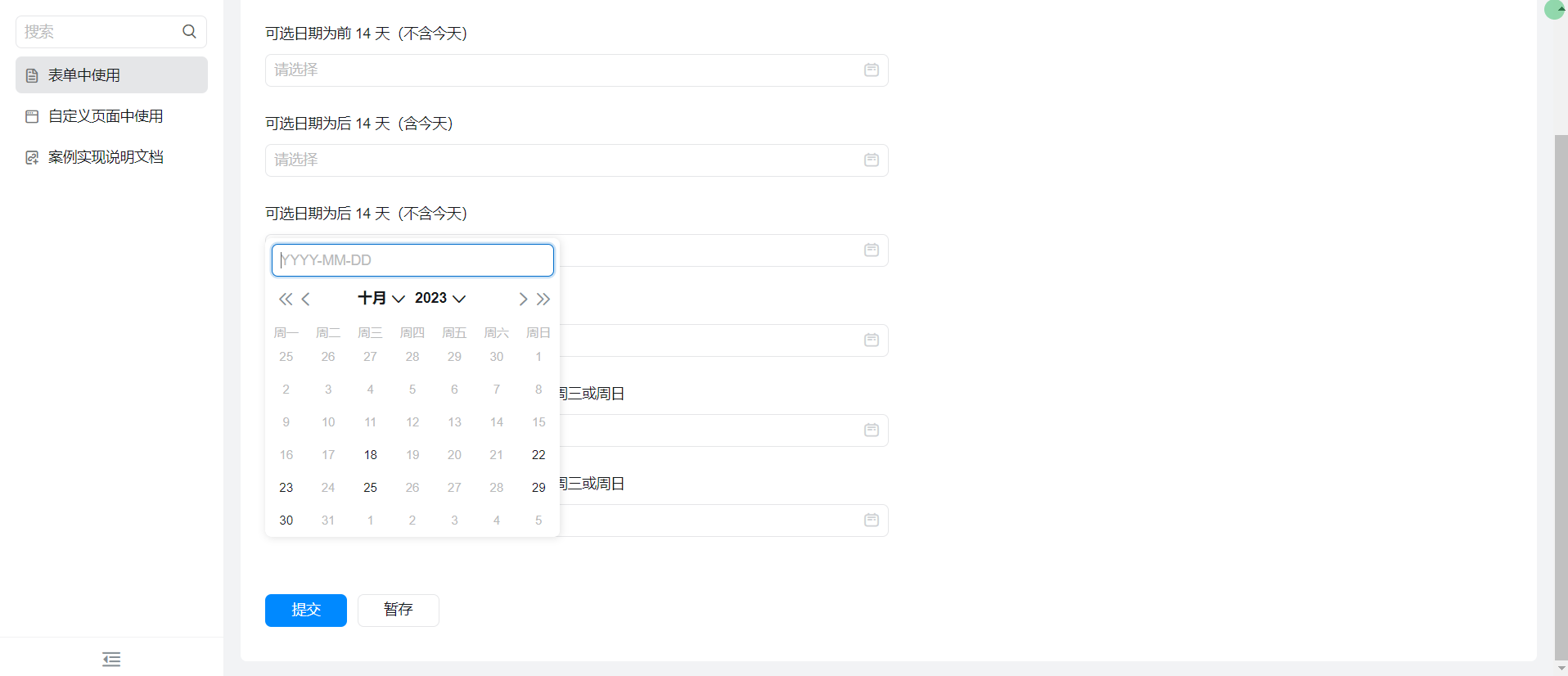
4. Try it online
This doc is generated using machine translation. Any discrepancies or differences created in the translation are not binding and have no legal effect for compliance or enforcement purposes.
本文档对您是否有帮助?